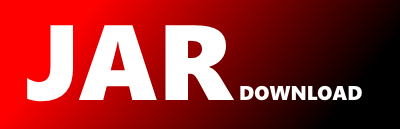
com.networknt.oas.jsonoverlay.ListOverlay Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-parser Show documentation
Show all versions of openapi-parser Show documentation
A light-weight, fast OpenAPI 3.0 parser and validator
/*******************************************************************************
* Copyright (c) 2017 ModelSolv, Inc. and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* ModelSolv, Inc. - initial API and implementation and/or initial documentation
*******************************************************************************/
package com.networknt.oas.jsonoverlay;
import com.fasterxml.jackson.core.JsonPointer;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.networknt.oas.jsonoverlay.SerializationOptions.Option;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
public class ListOverlay> extends JsonOverlay> {
private OverlayFactory itemFactory;
private List> overlays = new LinkedList<>();
public ListOverlay(Collection value, JsonOverlay> parent, OverlayFactory itemFactory,
ReferenceRegistry refReg) {
super(value, parent, refReg);
this.itemFactory = itemFactory;
fillWithValues();
}
public ListOverlay(JsonNode json, JsonOverlay> parent, OverlayFactory itemFactory,
ReferenceRegistry refReg) {
super(json, parent, refReg);
this.itemFactory = itemFactory;
fillWithJson();
}
private void fillWithValues() {
overlays.clear();
if (value != null) {
for (V item : value) {
overlays.add(new ChildOverlay(null, item, this, itemFactory, refReg));
}
}
}
private void fillWithJson() {
value.clear();
overlays.clear();
for (JsonNode itemJson : iterable(json.elements())) {
ChildOverlay overlay = new ChildOverlay<>(null, itemJson, this, itemFactory, refReg);
overlays.add(overlay);
value.add(overlay.get(false));
}
}
public Collection get(boolean elaborate) {
return value;
}
@Override
protected Collection fromJson(JsonNode json) {
return new ArrayList<>();
}
@Override
public IJsonOverlay> _find(JsonPointer path) {
int index = path.getMatchingIndex();
return overlays.size() > index ? overlays.get(index).find(path.tail()) : null;
}
@Override
public JsonNode toJson(SerializationOptions options) {
ArrayNode array = jsonArray();
for (IJsonOverlay overlay : overlays) {
array.add(overlay.toJson(options.plus(Option.KEEP_ONE_EMPTY)));
}
return array.size() > 0 || options.isKeepThisEmpty() ? array : jsonMissing();
}
public V get(int index) {
IJsonOverlay overlay = overlays.get(index);
return overlay != null ? overlay.get() : null;
}
public IJsonOverlay getOverlay(int index) {
return overlays.get(index);
}
public void set(int index, V value) {
overlays.set(index, itemFactory.create(value, this, refReg));
}
public void add(V value) {
overlays.add(itemFactory.create(value, this, refReg));
}
public void insert(int index, V value) {
overlays.add(index, itemFactory.create(value, this, refReg));
}
public void remove(int index) {
overlays.remove(index);
}
public int size() {
return overlays.size();
}
public boolean isReference(int index) {
@SuppressWarnings("unchecked")
ChildOverlay childOverlay = (ChildOverlay) overlays.get(index);
return childOverlay.isReference();
}
public Reference getReference(int index) {
@SuppressWarnings("unchecked")
ChildOverlay childOverlay = (ChildOverlay) overlays.get(index);
return childOverlay.getReference();
}
public static > OverlayFactory, ListOverlay> getFactory(
OverlayFactory itemFactory) {
return new ListOverlayFactory(itemFactory);
}
private static class ListOverlayFactory>
extends OverlayFactory, ListOverlay> {
private OverlayFactory itemFactory;
public ListOverlayFactory(OverlayFactory itemFactory) {
this.itemFactory = itemFactory;
}
@Override
protected Class super ListOverlay> getOverlayClass() {
return ListOverlay.class;
}
@Override
public ListOverlay _create(Collection value, JsonOverlay> parent, ReferenceRegistry refReg) {
return new ListOverlay(value, parent, itemFactory, refReg);
}
@Override
public ListOverlay _create(JsonNode json, JsonOverlay> parent, ReferenceRegistry refReg) {
return new ListOverlay(json, parent, itemFactory, refReg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy