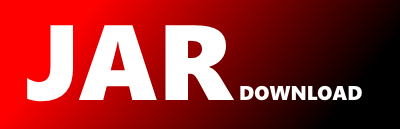
com.networknt.oas.jsonoverlay.PropertiesOverlay Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-parser Show documentation
Show all versions of openapi-parser Show documentation
A light-weight, fast OpenAPI 3.0 parser and validator
/*******************************************************************************
* Copyright (c) 2017 ModelSolv, Inc. and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* ModelSolv, Inc. - initial API and implementation and/or initial documentation
*******************************************************************************/
package com.networknt.oas.jsonoverlay;
import com.fasterxml.jackson.core.JsonPointer;
import com.fasterxml.jackson.databind.JsonNode;
import com.networknt.oas.jsonoverlay.SerializationOptions.Option;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
public abstract class PropertiesOverlay> extends JsonOverlay {
private List> children = new ArrayList<>();
protected Map> refables = new LinkedHashMap<>();
private boolean elaborated = false;
private boolean deferElaboration = false;
protected PropertiesOverlay(JsonNode json, JsonOverlay> parent, ReferenceRegistry refReg) {
super(json, parent, refReg);
deferElaboration = json.isMissingNode();
}
public PropertiesOverlay(V value, JsonOverlay> parent, ReferenceRegistry refReg) {
super(value, parent, refReg);
elaborated = true;
}
protected void maybeElaborateChildrenAtCreation() {
if (!deferElaboration) {
ensureElaborated();
}
}
protected void ensureElaborated() {
if (!elaborated) {
elaborateChildren();
elaborated = true;
}
}
protected abstract void elaborateChildren();
@Override
public boolean isElaborated() {
return elaborated;
}
@Override
public IJsonOverlay> _find(JsonPointer path) {
for (ChildOverlay, ?> child : children) {
if (child.matchesPath(path)) {
IJsonOverlay> found = child.find(child.tailPath(path));
if (found != null) {
return found;
}
}
}
return null;
}
@Override
public V fromJson(JsonNode json) {
// parsing of the json node is expected to be done in the constructor of the
// subclass, so nothing is done here. But we do establish this object as its own
// value.
@SuppressWarnings("unchecked")
V result = (V) this;
return result;
}
@Override
public JsonNode toJson(SerializationOptions options) {
JsonNode obj = jsonMissing();
for (ChildOverlay, ?> child : children) {
JsonNode childJson = child.toJson(options.minus(Option.KEEP_ONE_EMPTY));
if (!childJson.isMissingNode()) {
obj = child.getPath().setInPath(obj, childJson);
}
}
JsonNode result = fixJson(obj);
return result.size() > 0 || options.isKeepThisEmpty() ? result : jsonMissing();
}
protected JsonNode fixJson(JsonNode json) {
return json;
}
@Override
public V get(boolean elaborate) {
if (elaborate) {
ensureElaborated();
}
return value;
}
@Override
public void set(V value) {
super.set(value);
elaborateChildren();
}
protected > ChildOverlay createChild(boolean create, String path,
JsonOverlay> parent, OverlayFactory factory) {
return create ? createChild(path, parent, factory) : null;
}
protected > ChildOverlay createChild(String path, JsonOverlay> parent,
OverlayFactory factory) {
ChildOverlay child = new ChildOverlay<>(path, json.at("/" + path), parent, factory, refReg);
if (child.getOverlay() != null) {
child.getOverlay().setPathInParent(path);
}
children.add(child);
return child;
}
protected > ChildMapOverlay createChildMap(boolean create, String path,
JsonOverlay> parent, OverlayFactory factory, String keyPattern) {
return create ? createChildMap(path, parent, factory, keyPattern) : null;
}
protected > ChildMapOverlay createChildMap(String path,
JsonOverlay> parent, OverlayFactory factory, String keyPattern) {
ChildMapOverlay child = new ChildMapOverlay(path, json.at(pathPointer(path)), parent,
MapOverlay.getFactory(factory, keyPattern), refReg);
child.getOverlay().setPathInParent(path);
children.add(child);
return child;
}
protected > ChildListOverlay createChildList(boolean create, String path,
JsonOverlay> parent, OverlayFactory factory) {
return create ? createChildList(path, parent, factory) : null;
}
protected > ChildListOverlay createChildList(String path,
JsonOverlay> parent, OverlayFactory factory) {
ChildListOverlay child = new ChildListOverlay(path, json.at(pathPointer(path)), parent,
ListOverlay.getFactory(factory), refReg);
child.getOverlay().setPathInParent(path);
children.add(child);
return child;
}
public Iterable getRefablePaths() {
return refables.keySet();
}
public boolean isReference(String path) {
ChildOverlay, ?> child = (ChildOverlay, ?>) refables.get(path);
return child != null ? child.isReference() : false;
}
public Reference getReference(String path) {
ChildOverlay, ?> child = (ChildOverlay, ?>) refables.get(path);
return child != null ? child.getReference() : null;
}
private JsonPointer pathPointer(String path) {
return (path == null || path.isEmpty()) ? JsonPointer.compile("") : JsonPointer.compile("/" + path);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy