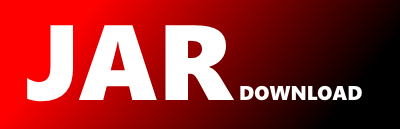
com.networknt.oas.validator.NumericUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-parser Show documentation
Show all versions of openapi-parser Show documentation
A light-weight, fast OpenAPI 3.0 parser and validator
/*******************************************************************************
* Copyright (c) 2017 ModelSolv, Inc. and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* ModelSolv, Inc. - initial API and implementation and/or initial documentation
*******************************************************************************/
package com.networknt.oas.validator;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.HashMap;
import java.util.Map;
public class NumericUtils {
public static boolean isNumeric(Object obj) {
return NumericType.of(obj) != null;
}
public static boolean isIntegral(Object obj) {
switch (NumericType.of(obj)) {
case BIG_INTEGER:
case BYTE:
case INTEGER:
case LONG:
case SHORT:
return true;
default:
return false;
}
}
public static T zero(T value) {
Number result;
switch (NumericType.of(value)) {
case BIG_DECIMAL:
result = BigDecimal.ZERO;
break;
case BIG_INTEGER:
result = BigInteger.ZERO;
break;
case BYTE:
result = Byte.valueOf((byte) 0);
break;
case DOUBLE:
result = Double.valueOf(0.0);
break;
case FLOAT:
result = Float.valueOf(0.0f);
break;
case INTEGER:
result = Integer.valueOf(0);
break;
case LONG:
result = Long.valueOf(0L);
break;
case SHORT:
result = Short.valueOf((short) 0);
break;
default:
return null; // shouldn't ever happen
}
@SuppressWarnings("unchecked")
T tResult = (T) result;
return tResult;
}
public static boolean gt(T x, T y) {
return compare(x, y) > 0;
}
public static boolean ge(T x, T y) {
return compare(x, y) >= 0;
}
public static boolean lt(T x, T y) {
return compare(x, y) < 0;
}
public static boolean le(T x, T y) {
return compare(x, y) <= 0;
}
public static boolean eq(T x, T y) {
return compare(x, y) == 0;
}
public static boolean ne(T x, T y) {
return compare(x, y) != 0;
}
public static int compare(T x, T y) {
NumericType type = NumericType.of(x);
if (type != NumericType.of(y)) {
throw new IllegalArgumentException();
}
switch (type) {
case BIG_DECIMAL:
return ((BigDecimal) x).compareTo((BigDecimal) y);
case BIG_INTEGER:
return ((BigInteger) x).compareTo((BigInteger) y);
case BYTE:
return ((Byte) x).compareTo((Byte) y);
case DOUBLE:
return ((Double) x).compareTo((Double) y);
case FLOAT:
return ((Float) x).compareTo((Float) y);
case INTEGER:
return ((Integer) x).compareTo((Integer) y);
case LONG:
return ((Long) x).compareTo((Long) y);
case SHORT:
return ((Short) x).compareTo((Short) y);
default:
throw new IllegalArgumentException();
}
}
enum NumericType {
BIG_DECIMAL(BigDecimal.class), //
BIG_INTEGER(BigInteger.class), //
BYTE(Byte.class), //
DOUBLE(Double.class), //
FLOAT(Float.class), //
INTEGER(Integer.class), //
LONG(Long.class), //
SHORT(Short.class);
private static Map, NumericType> types;
private NumericType(Class extends Number> cls) {
register(cls, this);
}
private void register(Class extends Number> cls, NumericType type) {
if (NumericType.types == null)
NumericType.types = new HashMap<>();
types.put(cls, type);
}
public static NumericType of(Object value) {
return value != null ? types.get(value.getClass()) : null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy