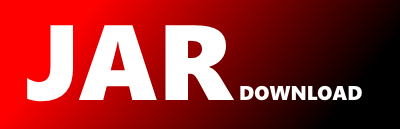
com.networknt.openapi.OpenApiHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-parser Show documentation
Show all versions of openapi-parser Show documentation
A light-weight, fast OpenAPI 3.0 parser and validator
/*
* Copyright (c) 2016 Network New Technologies Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.networknt.openapi;
import com.networknt.oas.OpenApiParser;
import com.networknt.oas.model.OpenApi3;
import com.networknt.oas.model.SecurityScheme;
import com.networknt.oas.model.Server;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.BiFunction;
/**
* This class load and cache openapi.json in a static block so that it can be
* shared by security for scope validation and validator for schema validation
*
* This handler supports openapi.yml, openapi.yaml and openapi.json and above
* is the loading sequence.
*
* Due to the high demand, we have changed this class from singleton to normal
* class to support multiple instances of specifications in light-rest-4j.
*
* Some of our users want to use one light-gateway to support multiple backend
* services to reduce the resource utilization.
*
* @author Steve Hu
*/
public class OpenApiHelper {
static final Logger logger = LoggerFactory.getLogger(OpenApiHelper.class);
public OpenApi3 openApi3;
public List oauth2Names;
public String basePath;
public OpenApiHelper(String spec) {
try {
openApi3 = (OpenApi3) new OpenApiParser().parse(spec, new URL("https://oas.lightapi.net/"));
} catch (MalformedURLException e) {
logger.error("MalformedURLException", e);
}
if(openApi3 == null) {
logger.error("Unable to load openapi.json");
throw new RuntimeException("Unable to load openapi.json");
} else {
oauth2Names = getOAuth2Name();
basePath = getBasePath();
}
}
/**
* merge inject map to openapi map
* @param openapi {@link Map} openapi
* @param inject {@link Map} openapi
*/
public static void merge(Map openapi, Map inject) {
if (inject == null) {
return;
}
for (Map.Entry entry : inject.entrySet()) {
openapi.merge(entry.getKey(), entry.getValue(), new Merger());
}
}
// merge in case of map, add in case of list
static class Merger implements BiFunction {
@Override
public Object apply(Object o, Object i) {
if (o instanceof Map && i instanceof Map) {
for (Map.Entry entry : ((Map) i).entrySet()) {
((Map) o).merge(entry.getKey(), entry.getValue(), new Merger());
}
} else if (o instanceof List && i instanceof List) {
((List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy