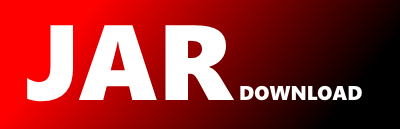
com.networknt.openapi.SimpleJwtVerifyHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-security Show documentation
Show all versions of openapi-security Show documentation
An OpenAPI Specification 3.0 security module that contains all handlers to protect your server
The newest version!
package com.networknt.openapi;
import com.networknt.config.Config;
import com.networknt.handler.Handler;
import com.networknt.handler.MiddlewareHandler;
import com.networknt.handler.config.HandlerConfig;
import com.networknt.security.*;
import com.networknt.utility.ModuleRegistry;
import io.undertow.Handlers;
import io.undertow.server.HttpHandler;
import io.undertow.server.HttpServerExchange;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This is very simple jwt verify handler that is used to verify jwt token without scopes. Other than scopes, it is
* the same as the normal JwtVerifyHandler.
*
* @author Steve Hu
*/
public class SimpleJwtVerifyHandler extends AbstractSimpleJwtVerifyHandler {
static final Logger logger = LoggerFactory.getLogger(SimpleJwtVerifyHandler.class);
String basePath;
public SimpleJwtVerifyHandler() {
// at this moment, we assume that the OpenApiHandler is fully loaded with a single spec or multiple specs.
// And the basePath is the correct one from the OpenApiHandler helper or helperMap if multiple is used.
config = SecurityConfig.load();
jwtVerifier = new JwtVerifier(config);
// in case that the specification doesn't exist, get the basePath from the handler.yml for endpoint lookup.
HandlerConfig handlerConfig = HandlerConfig.load();
this.basePath = handlerConfig == null ? "/" : handlerConfig.getBasePath();
}
@Override
public HttpHandler getNext() {
return next;
}
@Override
public MiddlewareHandler setNext(final HttpHandler next) {
Handlers.handlerNotNull(next);
this.next = next;
return this;
}
@Override
public boolean isEnabled() {
return config.isEnableVerifyJwt();
}
@Override
public void register() {
ModuleRegistry.registerModule(SecurityConfig.CONFIG_NAME, SimpleJwtVerifyHandler.class.getName(), Config.getNoneDecryptedInstance().getJsonMapConfigNoCache(SecurityConfig.CONFIG_NAME), null);
}
@Override
public void reload() {
config.reload();
jwtVerifier = new JwtVerifier(config);
ModuleRegistry.registerModule(SecurityConfig.CONFIG_NAME, SimpleJwtVerifyHandler.class.getName(), Config.getNoneDecryptedInstance().getJsonMapConfigNoCache(SecurityConfig.CONFIG_NAME), null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy