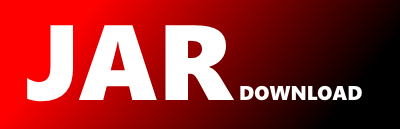
com.neuronrobotics.imageprovider.Example Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of BowlerScriptingKernel Show documentation
Show all versions of BowlerScriptingKernel Show documentation
A command line utility for accsing the bowler framework.
package com.neuronrobotics.imageprovider;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JFrame;
import javax.swing.JPanel;
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.Point;
import org.opencv.core.Scalar;
import org.opencv.core.Size;
import org.opencv.highgui.VideoCapture;
import org.opencv.imgproc.Imgproc;
import org.opencv.core.CvType;
class Panel extends JPanel{
private static final long serialVersionUID = 1L;
private BufferedImage image;
// Create a constructor method
public Panel(){
super();
}
private BufferedImage getimage(){
return image;
}
public void setimage(BufferedImage newimage){
image=newimage;
return;
}
public void setimagewithMat(Mat newimage){
image=this.matToBufferedImage(newimage);
return;
}
/**
* Converts/writes a Mat into a BufferedImage.
*
* @param matrix Mat of type CV_8UC3 or CV_8UC1
* @return BufferedImage of type TYPE_3BYTE_BGR or TYPE_BYTE_GRAY
*/
public BufferedImage matToBufferedImage(Mat matrix) {
int cols = matrix.cols();
int rows = matrix.rows();
int elemSize = (int)matrix.elemSize();
byte[] data = new byte[cols * rows * elemSize];
int type;
matrix.get(0, 0, data);
switch (matrix.channels()) {
case 1:
type = BufferedImage.TYPE_BYTE_GRAY;
break;
case 3:
type = BufferedImage.TYPE_3BYTE_BGR;
// bgr to rgb
byte b;
for(int i=0; i lhsv = new ArrayList(3);
Mat circles = new Mat(); // No need (and don't know how) to initialize it.
// The function later will do it... (to a 1*N*CV_32FC3)
Scalar hsv_min = new Scalar(0, 50, 50, 0);
Scalar hsv_max = new Scalar(6, 255, 255, 0);
Scalar hsv_min2 = new Scalar(175, 50, 50, 0);
Scalar hsv_max2 = new Scalar(179, 255, 255, 0);
double[] data=new double[3];
if( capture.isOpened())
{
while( true )
{
capture.read(webcam_image);
if( !webcam_image.empty() )
{
// One way to select a range of colors by Hue
Imgproc.cvtColor(webcam_image, hsv_image, Imgproc.COLOR_BGR2HSV);
Core.inRange(hsv_image, hsv_min, hsv_max, thresholded);
Core.inRange(hsv_image, hsv_min2, hsv_max2, thresholded2);
Core.bitwise_or(thresholded, thresholded2, thresholded);
// Notice that the thresholds don't really work as a "distance"
// Ideally we would like to cut the image by hue and then pick just
// the area where S combined V are largest.
// Strictly speaking, this would be something like sqrt((255-S)^2+(255-V)^2)>Range
// But if we want to be "faster" we can do just (255-S)+(255-V)>Range
// Or otherwise 510-S-V>Range
// Anyhow, we do the following... Will see how fast it goes...
Core.split(hsv_image, lhsv); // We get 3 2D one channel Mats
Mat S = lhsv.get(1);
Mat V = lhsv.get(2);
Core.subtract(array255, S, S);
Core.subtract(array255, V, V);
S.convertTo(S, CvType.CV_32F);
V.convertTo(V, CvType.CV_32F);
Core.magnitude(S, V, distance);
Core.inRange(distance,new Scalar(0.0), new Scalar(200.0), thresholded2);
Core.bitwise_and(thresholded, thresholded2, thresholded);
// Apply the Hough Transform to find the circles
Imgproc.GaussianBlur(thresholded, thresholded, new Size(9,9),0,0);
Imgproc.HoughCircles(thresholded, circles, Imgproc.CV_HOUGH_GRADIENT, 2, thresholded.height()/4, 500, 50, 0, 0);
//Imgproc.Canny(thresholded, thresholded, 500, 250);
//-- 4. Add some info to the image
Core.line(webcam_image, new Point(150,50), new Point(202,200), new Scalar(100,10,10)/*CV_BGR(100,10,10)*/, 3);
Core.circle(webcam_image, new Point(210,210), 10, new Scalar(100,10,10),3);
data=webcam_image.get(210, 210);
Core.putText(webcam_image,String.format("("+String.valueOf(data[0])+","+String.valueOf(data[1])+","+String.valueOf(data[2])+")"),new Point(30, 30) , 3 //FONT_HERSHEY_SCRIPT_SIMPLEX
,1.0,new Scalar(100,10,10,255),3);
//int cols = circles.cols();
int rows = circles.rows();
int elemSize = (int)circles.elemSize(); // Returns 12 (3 * 4bytes in a float)
float[] data2 = new float[rows * elemSize/4];
if (data2.length>0){
circles.get(0, 0, data2); // Points to the first element and reads the whole thing
// into data2
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy