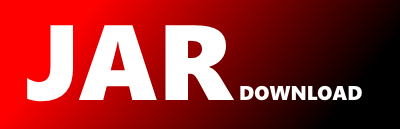
eu.mihosoft.vrl.v3d.Polyhedron Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package eu.mihosoft.vrl.v3d;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;
// TODO: Auto-generated Javadoc
/**
* Polyhedron.
*
* @author Michael Hoffer <[email protected]>
*/
public class Polyhedron extends Primitive {
/** The properties. */
private final PropertyStorage properties = new PropertyStorage();
/** The points. */
private final List points = new ArrayList<>();
/** The faces. */
private final List> faces = new ArrayList<>();
/**
* Constructor. Creates a polyhedron defined by a list of points and a list
* of faces.
*
* @param points points ({@link Vector3d} list)
* @param faces list of faces (list of point index lists)
*/
public Polyhedron(List points, List> faces) {
this.points.addAll(points);
this.faces.addAll(faces);
}
/**
* Constructor. Creates a polyhedron defined by a list of points and a list
* of faces.
*
* @param points points ({@link Vector3d} array)
* @param faces list of faces (array of point index arrays)
*/
public Polyhedron(Vector3d[] points, Integer[][] faces) {
this.points.addAll(Arrays.asList(points));
for (Integer[] list : faces) {
this.faces.add(Arrays.asList(list));
}
}
/* (non-Javadoc)
* @see eu.mihosoft.vrl.v3d.Primitive#toPolygons()
*/
@Override
public List toPolygons() {
Function indexToPoint = (Integer i) -> {
return points.get(i).clone();
};
Function, Polygon> faceListToPolygon
= (List faceList) -> {
return Polygon.fromPoints(faceList.stream().map(indexToPoint).
collect(Collectors.toList()), properties);
};
return faces.stream().map(faceListToPolygon).
collect(Collectors.toList());
}
/* (non-Javadoc)
* @see eu.mihosoft.vrl.v3d.Primitive#getProperties()
*/
@Override
public PropertyStorage getProperties() {
return properties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy