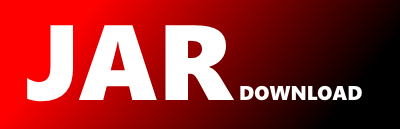
model.MARK_II.util.RegionConsoleViewer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of WalnutiQ Show documentation
Show all versions of WalnutiQ Show documentation
A Java based Neuron Modeling framework
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy