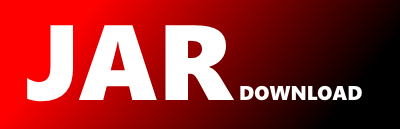
com.neuronrobotics.sdk.wireless.bluetooth.BlueCoveManager Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2010 Neuron Robotics, LLC
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package com.neuronrobotics.sdk.wireless.bluetooth;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import javax.bluetooth.BluetoothStateException;
import javax.bluetooth.DeviceClass;
import javax.bluetooth.DiscoveryAgent;
import javax.bluetooth.DiscoveryListener;
import javax.bluetooth.LocalDevice;
import javax.bluetooth.RemoteDevice;
import javax.bluetooth.ServiceRecord;
import javax.bluetooth.UUID;
import javax.microedition.io.Connector;
import javax.microedition.io.StreamConnection;
import com.neuronrobotics.sdk.common.Log;
import com.neuronrobotics.sdk.common.MissingNativeLibraryException;
// TODO: Auto-generated Javadoc
//import com.intel.bluetooth.test.SimpleClient.CancelThread;
/**
* The Class BlueCoveManager.
*/
public class BlueCoveManager implements DiscoveryListener {
/** The records. */
ArrayList records= new ArrayList();
/** The device list. */
ArrayList deviceList = new ArrayList();
/** The Constant uuid. */
static final UUID uuid = com.intel.bluetooth.BluetoothConsts.RFCOMM_PROTOCOL_UUID;
/** The selected. */
private String selected = null;
/** The ins. */
private DataInputStream ins;
/** The outs. */
private DataOutputStream outs;
/** The conn. */
private StreamConnection conn;
/** The search id. */
private int searchId=0xffff;
/**
* Instantiates a new blue cove manager.
*/
public BlueCoveManager(){
}
/**
* Find.
*
* @throws MissingNativeLibraryException the missing native library exception
*/
public synchronized void find() throws MissingNativeLibraryException {
for (RemoteDevice d:deviceList) {
if(d.getBluetoothAddress().equals(selected)){
try {Thread.sleep(100);} catch (InterruptedException e) {}
return;
}
}
disconnect();
Log.info("Finding Devices...");
try {
LocalDevice.getLocalDevice().getDiscoveryAgent().cancelInquiry(this);
LocalDevice.getLocalDevice().getDiscoveryAgent().startInquiry(DiscoveryAgent.GIAC, this);
try {wait();} catch (InterruptedException e) {e.printStackTrace();}
} catch (BluetoothStateException e) {
e.printStackTrace();
}
for (RemoteDevice d:deviceList) {
try {
Log.info("Device name: " + d.getFriendlyName(false)+" address: " + d.getBluetoothAddress());
} catch (IOException e) {
//e.printStackTrace();
}
}
if(deviceList.size()==0)
Log.info("No Devices Found!");
}
/* (non-Javadoc)
* @see javax.bluetooth.DiscoveryListener#deviceDiscovered(javax.bluetooth.RemoteDevice, javax.bluetooth.DeviceClass)
*/
public void deviceDiscovered(RemoteDevice btDevice, DeviceClass cod) {
deviceList.add(btDevice);
//Log.info("deviceDiscovered " + btDevice.getBluetoothAddress() + " DeviceClass: " + ((Object)cod).toString());
}
/* (non-Javadoc)
* @see javax.bluetooth.DiscoveryListener#inquiryCompleted(int)
*/
public synchronized void inquiryCompleted(int discType) {
notifyAll();
}
/* (non-Javadoc)
* @see javax.bluetooth.DiscoveryListener#servicesDiscovered(int, javax.bluetooth.ServiceRecord[])
*/
public void servicesDiscovered(int transID, ServiceRecord[] servRecord) {
//Log.info("###Record list added: "+servRecord.length);
for ( int i=0; i< servRecord.length;i++)
records.add(servRecord[i]);
}
/* (non-Javadoc)
* @see javax.bluetooth.DiscoveryListener#serviceSearchCompleted(int, int)
*/
public synchronized void serviceSearchCompleted(int transID, int respCode) {
notifyAll();
}
/**
* Gets the available serial devices.
*
* @param refresh the refresh
* @return the available serial devices
*/
public String [] getAvailableSerialDevices(boolean refresh) {
if(refresh){
find();
}
String [] s=new String[deviceList.size()];
String tmp="";
int i=0;
for (RemoteDevice d: deviceList){
try {
tmp=d.getFriendlyName(false);
if (tmp == "")
tmp = "No Name";
s[i]=tmp+"_"+d.getBluetoothAddress();
} catch (Exception e) {
s[i]="Failed Name"+"_"+d.getBluetoothAddress();
}
i++;
}
return s;
}
/**
* Gets the device.
*
* @param name the name
* @return the device
*/
public synchronized RemoteDevice getDevice(String name){
String addr = name.substring(name.indexOf('_')+1);
//System.out.println("Getting device with address: "+addr);
String [] s=getAvailableSerialDevices(false);
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy