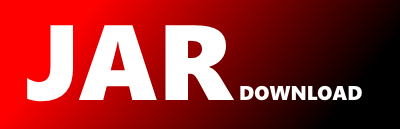
com.newmainsoftech.ant.taskdefs.maven.MavenProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ant-util Show documentation
Show all versions of ant-util Show documentation
Ant utilities for stand-alone Ant environment and maven-antrun-plugin environment.
/*
* Copyright (C) 2012 NewMain Softech
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package com.newmainsoftech.ant.taskdefs.maven;
import java.util.Properties;
import org.apache.tools.ant.taskdefs.Property;
import org.apache.tools.ant.types.LogLevel;
import com.newmainsoftech.ant.MavenProjectAccessor;
import com.newmainsoftech.ant.types.maven.MavenPath;
import com.newmainsoftech.ant.types.maven.MavenPath.MvnPathElement;
/**
* Extension of {@link Property} to conveniently get path to dependency binary in Maven local
* repository and define it as a property, or to add Ant property or reference to Maven property
* especially in the usage case via maven-antrun-plugin.
* This can also be used in stand-alone Ant environment; in such usage, {@link #anttoo} attribute
* should be set true.
*
* Usage example
* Use mvnproperty
tag in Ant's target
tag (what can be defined in
* configuration
tag of manve-antrun-plugin's execution
tag as well
* as in an Ant file).
*
* - To get and define dependency binary's path in Maven local repository
* Let's say that you don't have the dependency tag defined for gwt-dev.jar in the pom.xml
* of your Maven project, or you may like to have the path to a dependency binary in the Maven
* local repository in the stand-alone Ant environment.
*
* <mvnproperty
* dependency="com.google.gwt:gwt-dev:2.4.0" />
*
* After that, you can get the path to the gwt-dev.jar file in the Maven local repository by
* referring the ${com.google.gwt:gwt-dev:jar} property value as if it had been defined in
* the dependency section of the Maven pom.xml file.
*
* - To have Maven property defined at same time
* The usage is identical with the Ant's regular property
tag.
*
*
* <mvnproperty
* name="mvnTestProp1"
* value="mvnTestProp1Value" />
* <mvnproperty
* name="mvnTestProp2"
* location="${calculated-dependency-path}" />
*
*
* By those, Maven properties, mvnTestProp1 and mvnTestProp2 are defined with specified values and
* Ant properties as well at the same time.
* Note: Ant property is well known as immutable, however Maven property is mutable at least within
* a component project though it's not persistent to super project or sibling project.
*
*
*
* Configuration
*
* - Define
path
tag for this project's archive ant-util.jar file and sub-dependencies.
*
* - In the case of using maven-antrun-plugin and you can define com.newmainsoftech:ant-util:jar
* as a dependency of maven-antrun-plugin, you can do like below.
*
*
* <property
* name="plugin_classpath"
* refid="maven.plugin.classpath" />
* <path id="antutil_path"
* path="${plugin_classpath}" />
*
*
*
* - If you cannot define com.newmainsoftech:ant-util:jar as a dependency of maven-antrun-plugin
* in project's pom.xml, or the case of stand alone Ant environment, then you need to provide path to
* com.newmainsoftech:ant-util:jar.
* And if you use the dependency
attribute, then
* additionally provide path to the following components:
*
* - org.sonatype.aether:aether-api:jar
* - org.sonatype.aether:aether-util:jar
* - org.sonatype.aether:aether-spi:jar
* - org.sonatype.aether:aether-connector-wagon:jar
* - org.apache.httpcomponents:httpcore:jar
* - commons-logging:commons-logging:jar
* - org.apache.httpcomponents:httpclient:jar
* - org.apache.maven.wagon:wagon-http:jar
* - org.apache.maven.wagon:wagon-http-shared4:jar
*
* And define path
tag with those paths:
*
* <path id="antutil_path"
* path="${lengthy_path_of_antutil_and_fellows}" />
*
*
*
*
* - Define
taskdef
tag for the ant-util's antlib.xml
*
*
* <taskdef
* resource="com/newmainsoftech/ant/antlib.xml"
* classpathref="antutil_path" />
*
*
*
*
*
*
* @author Arata Y.
*/
public class MavenProperty extends Property {
private MavenProjectAccessor mavenProjectAccessor = new MavenProjectAccessor();
protected MavenProjectAccessor getMavenProjectAccessor() {
return mavenProjectAccessor;
}
protected void setMavenProjectAccessor( MavenProjectAccessor mavenProjectAccessor) {
this.mavenProjectAccessor = mavenProjectAccessor;
}
/**
* Attribute to switch whether set property as Ant property too additionally to Maven property.
* Default value is true.
*/
private boolean anttoo = true;
/**
* Get {@link #anttoo} attribute value what is switch to controls whether set property as
* Ant property too additionally to Maven property.
* @return value of anttoo
attribute.
*/
public synchronized boolean getAnttoo() {
return anttoo;
}
/**
* Set value to {@link #anttoo} attribute what is switch to controls whether set property as
* Ant property too additionally to Maven property.
* @param anttoo
* @see #addProperty(String, Object)
*/
public synchronized void setAnttoo( boolean anttoo) {
this.anttoo = anttoo;
}
/**
* Add a value pair of name
and value
inputs as Maven property.
* When {@link #anttoo} attribute value is true, the pair will be added as Ant property too as long
* as another Ant property for same name does not exist since Ant property is immutable.
*
* @param name name of property being added.
* @param value value of property being added.
*/
@Override
protected void addProperty( String name, Object value) {
if ( name == null) {
throw new IllegalArgumentException( "Value of name input cannot be null.");
}
// Obtain access to properties of MavenProject --------------------------------------------
MavenProjectAccessor mavenProjectAccessor = getMavenProjectAccessor();
Object mavenProjectObj = mavenProjectAccessor.getMavenProject( this);
Properties mavenProperties = mavenProjectAccessor.getMavenProperties( mavenProjectObj);
if ( mavenProjectObj == null) {
if ( getAnttoo()) {
log(
String.format(
"Since could not detect the reference to MavenProject object, and "
+ "anttoo attribute is set to true, going to attempt to set a pair "
+ "of name (%1$s) and value (%2$s) only as an Ant property but a Maven "
+ "property.",
name, value
),
LogLevel.INFO.getLevel()
);
}
else {
log(
String.format(
"Since could not detect the reference to MavenProject object, and "
+ "anttoo attribute is set to false, returning without attempting to "
+ "set a pair of name (%1$s) and value (%2$s) at all.",
name, value
),
LogLevel.WARN.getLevel()
);
return;
}
}
else if ( mavenProperties == null) {
throw new IllegalStateException(
String.format(
"Could not obtain the access to properties of Maven project (%1$s).",
mavenProjectObj
)
);
}
// ----------------------------------------------------------------------------------------
// Set Maven property ---------------------------------------------------------------------
if ( mavenProperties != null) {
String presetValue = mavenProperties.getProperty( name);
boolean equalsFlag = false;
if ( presetValue == value) equalsFlag = true;
// Either preSetAntValue or value is not null
else if (( value != null) && value.toString().equals( presetValue)) equalsFlag = true;
if ( !equalsFlag) {
mavenProperties.setProperty( name, value.toString());
if ( presetValue == null) {
this.log(
String.format(
"Added property (key:%1$s, value:%2$s) to %3$s Maven project.",
name,
value.toString(),
mavenProjectAccessor.getMavenProjectName( mavenProjectObj)
),
LogLevel.VERBOSE.getLevel()
);
}
else {
this.log(
String.format(
"Overwrited value of %1$s property from %2$s to %3$s in "
+ "%4$s Maven project.",
name,
((presetValue == null) ? "null" : presetValue),
((value == null) ? "null" : value.toString()),
mavenProjectAccessor.getMavenProjectName( mavenProjectObj)
),
LogLevel.INFO.getLevel()
);
}
}
else {
this.log(
String.format(
"Skipped to add property (key:%1$s, value:%2$s) to %3$s Maven project "
+ "since it has already been defined with the same value.",
name,
value.toString(),
mavenProjectAccessor.getMavenProjectName( mavenProjectObj)
),
LogLevel.VERBOSE.getLevel()
);
}
}
// ----------------------------------------------------------------------------------------
if ( getAnttoo()) {
String preSetAntValue = getProject().getProperty( name);
boolean equalsFlag = false;
if ( preSetAntValue == value) equalsFlag = true;
// Either preSetAntValue or value is not null
else if (( value != null) && value.toString().equals( preSetAntValue)) equalsFlag = true;
if ( !equalsFlag) {
super.addProperty( name, value);
if ( preSetAntValue == null) {
log(
String.format(
"Added an Ant property of what name is %1$s and value is %2$s.",
name,
value),
LogLevel.VERBOSE.getLevel()
);
}
else {
log(
String.format(
"Though overwrited value of %1$s Ant property from %2$s to %3$s, "
+ "it will be short live since Ant property is immutable.",
name,
preSetAntValue,
((value == null) ? "null" : value.toString())),
LogLevel.INFO.getLevel()
);
}
}
else {
this.log(
String.format(
"Skipped to add property (key:%1$s, value:%2$s) as an Ant property "
+ "since it has already been defined with the same value.",
name,
value.toString()
),
LogLevel.VERBOSE.getLevel()
);
}
}
}
@Override
protected void addProperty( String name, String valueStr) {
this.addProperty( name, (Object)valueStr);
}
public void setDependency( final String dependencyId) {
MavenPath mavenPath = new MavenPath( getProject());
MvnPathElement mvnPathElement = mavenPath.createMvnPathElement();
mvnPathElement.setDependencyId( dependencyId);
String dependencyIdCopy = mvnPathElement.getDependencyId();
this.setName( dependencyIdCopy.substring( 0, dependencyIdCopy.lastIndexOf( ":")));
this.setValue( mvnPathElement.getDependencyPath());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy