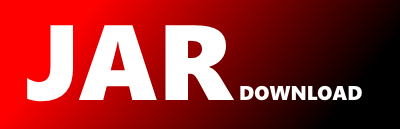
com.nextbreakpoint.flink.client.model.CheckpointConfigInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.ExternalizedCheckpointInfo;
import com.nextbreakpoint.flink.client.model.ProcessingMode;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* CheckpointConfigInfo
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class CheckpointConfigInfo {
@SerializedName("aligned_checkpoint_timeout")
private Long alignedCheckpointTimeout = null;
@SerializedName("changelog_periodic_materialization_interval")
private Long changelogPeriodicMaterializationInterval = null;
@SerializedName("changelog_storage")
private String changelogStorage = null;
@SerializedName("checkpoint_storage")
private String checkpointStorage = null;
@SerializedName("checkpoints_after_tasks_finish")
private Boolean checkpointsAfterTasksFinish = null;
@SerializedName("externalization")
private ExternalizedCheckpointInfo externalization = null;
@SerializedName("interval")
private Long interval = null;
@SerializedName("max_concurrent")
private Long maxConcurrent = null;
@SerializedName("min_pause")
private Long minPause = null;
@SerializedName("mode")
private ProcessingMode mode = null;
@SerializedName("state_backend")
private String stateBackend = null;
@SerializedName("state_changelog_enabled")
private Boolean stateChangelogEnabled = null;
@SerializedName("timeout")
private Long timeout = null;
@SerializedName("tolerable_failed_checkpoints")
private Integer tolerableFailedCheckpoints = null;
@SerializedName("unaligned_checkpoints")
private Boolean unalignedCheckpoints = null;
public CheckpointConfigInfo alignedCheckpointTimeout(Long alignedCheckpointTimeout) {
this.alignedCheckpointTimeout = alignedCheckpointTimeout;
return this;
}
/**
* Get alignedCheckpointTimeout
* @return alignedCheckpointTimeout
**/
@Schema(description = "")
public Long getAlignedCheckpointTimeout() {
return alignedCheckpointTimeout;
}
public void setAlignedCheckpointTimeout(Long alignedCheckpointTimeout) {
this.alignedCheckpointTimeout = alignedCheckpointTimeout;
}
public CheckpointConfigInfo changelogPeriodicMaterializationInterval(Long changelogPeriodicMaterializationInterval) {
this.changelogPeriodicMaterializationInterval = changelogPeriodicMaterializationInterval;
return this;
}
/**
* Get changelogPeriodicMaterializationInterval
* @return changelogPeriodicMaterializationInterval
**/
@Schema(description = "")
public Long getChangelogPeriodicMaterializationInterval() {
return changelogPeriodicMaterializationInterval;
}
public void setChangelogPeriodicMaterializationInterval(Long changelogPeriodicMaterializationInterval) {
this.changelogPeriodicMaterializationInterval = changelogPeriodicMaterializationInterval;
}
public CheckpointConfigInfo changelogStorage(String changelogStorage) {
this.changelogStorage = changelogStorage;
return this;
}
/**
* Get changelogStorage
* @return changelogStorage
**/
@Schema(description = "")
public String getChangelogStorage() {
return changelogStorage;
}
public void setChangelogStorage(String changelogStorage) {
this.changelogStorage = changelogStorage;
}
public CheckpointConfigInfo checkpointStorage(String checkpointStorage) {
this.checkpointStorage = checkpointStorage;
return this;
}
/**
* Get checkpointStorage
* @return checkpointStorage
**/
@Schema(description = "")
public String getCheckpointStorage() {
return checkpointStorage;
}
public void setCheckpointStorage(String checkpointStorage) {
this.checkpointStorage = checkpointStorage;
}
public CheckpointConfigInfo checkpointsAfterTasksFinish(Boolean checkpointsAfterTasksFinish) {
this.checkpointsAfterTasksFinish = checkpointsAfterTasksFinish;
return this;
}
/**
* Get checkpointsAfterTasksFinish
* @return checkpointsAfterTasksFinish
**/
@Schema(description = "")
public Boolean isCheckpointsAfterTasksFinish() {
return checkpointsAfterTasksFinish;
}
public void setCheckpointsAfterTasksFinish(Boolean checkpointsAfterTasksFinish) {
this.checkpointsAfterTasksFinish = checkpointsAfterTasksFinish;
}
public CheckpointConfigInfo externalization(ExternalizedCheckpointInfo externalization) {
this.externalization = externalization;
return this;
}
/**
* Get externalization
* @return externalization
**/
@Schema(description = "")
public ExternalizedCheckpointInfo getExternalization() {
return externalization;
}
public void setExternalization(ExternalizedCheckpointInfo externalization) {
this.externalization = externalization;
}
public CheckpointConfigInfo interval(Long interval) {
this.interval = interval;
return this;
}
/**
* Get interval
* @return interval
**/
@Schema(description = "")
public Long getInterval() {
return interval;
}
public void setInterval(Long interval) {
this.interval = interval;
}
public CheckpointConfigInfo maxConcurrent(Long maxConcurrent) {
this.maxConcurrent = maxConcurrent;
return this;
}
/**
* Get maxConcurrent
* @return maxConcurrent
**/
@Schema(description = "")
public Long getMaxConcurrent() {
return maxConcurrent;
}
public void setMaxConcurrent(Long maxConcurrent) {
this.maxConcurrent = maxConcurrent;
}
public CheckpointConfigInfo minPause(Long minPause) {
this.minPause = minPause;
return this;
}
/**
* Get minPause
* @return minPause
**/
@Schema(description = "")
public Long getMinPause() {
return minPause;
}
public void setMinPause(Long minPause) {
this.minPause = minPause;
}
public CheckpointConfigInfo mode(ProcessingMode mode) {
this.mode = mode;
return this;
}
/**
* Get mode
* @return mode
**/
@Schema(description = "")
public ProcessingMode getMode() {
return mode;
}
public void setMode(ProcessingMode mode) {
this.mode = mode;
}
public CheckpointConfigInfo stateBackend(String stateBackend) {
this.stateBackend = stateBackend;
return this;
}
/**
* Get stateBackend
* @return stateBackend
**/
@Schema(description = "")
public String getStateBackend() {
return stateBackend;
}
public void setStateBackend(String stateBackend) {
this.stateBackend = stateBackend;
}
public CheckpointConfigInfo stateChangelogEnabled(Boolean stateChangelogEnabled) {
this.stateChangelogEnabled = stateChangelogEnabled;
return this;
}
/**
* Get stateChangelogEnabled
* @return stateChangelogEnabled
**/
@Schema(description = "")
public Boolean isStateChangelogEnabled() {
return stateChangelogEnabled;
}
public void setStateChangelogEnabled(Boolean stateChangelogEnabled) {
this.stateChangelogEnabled = stateChangelogEnabled;
}
public CheckpointConfigInfo timeout(Long timeout) {
this.timeout = timeout;
return this;
}
/**
* Get timeout
* @return timeout
**/
@Schema(description = "")
public Long getTimeout() {
return timeout;
}
public void setTimeout(Long timeout) {
this.timeout = timeout;
}
public CheckpointConfigInfo tolerableFailedCheckpoints(Integer tolerableFailedCheckpoints) {
this.tolerableFailedCheckpoints = tolerableFailedCheckpoints;
return this;
}
/**
* Get tolerableFailedCheckpoints
* @return tolerableFailedCheckpoints
**/
@Schema(description = "")
public Integer getTolerableFailedCheckpoints() {
return tolerableFailedCheckpoints;
}
public void setTolerableFailedCheckpoints(Integer tolerableFailedCheckpoints) {
this.tolerableFailedCheckpoints = tolerableFailedCheckpoints;
}
public CheckpointConfigInfo unalignedCheckpoints(Boolean unalignedCheckpoints) {
this.unalignedCheckpoints = unalignedCheckpoints;
return this;
}
/**
* Get unalignedCheckpoints
* @return unalignedCheckpoints
**/
@Schema(description = "")
public Boolean isUnalignedCheckpoints() {
return unalignedCheckpoints;
}
public void setUnalignedCheckpoints(Boolean unalignedCheckpoints) {
this.unalignedCheckpoints = unalignedCheckpoints;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CheckpointConfigInfo checkpointConfigInfo = (CheckpointConfigInfo) o;
return Objects.equals(this.alignedCheckpointTimeout, checkpointConfigInfo.alignedCheckpointTimeout) &&
Objects.equals(this.changelogPeriodicMaterializationInterval, checkpointConfigInfo.changelogPeriodicMaterializationInterval) &&
Objects.equals(this.changelogStorage, checkpointConfigInfo.changelogStorage) &&
Objects.equals(this.checkpointStorage, checkpointConfigInfo.checkpointStorage) &&
Objects.equals(this.checkpointsAfterTasksFinish, checkpointConfigInfo.checkpointsAfterTasksFinish) &&
Objects.equals(this.externalization, checkpointConfigInfo.externalization) &&
Objects.equals(this.interval, checkpointConfigInfo.interval) &&
Objects.equals(this.maxConcurrent, checkpointConfigInfo.maxConcurrent) &&
Objects.equals(this.minPause, checkpointConfigInfo.minPause) &&
Objects.equals(this.mode, checkpointConfigInfo.mode) &&
Objects.equals(this.stateBackend, checkpointConfigInfo.stateBackend) &&
Objects.equals(this.stateChangelogEnabled, checkpointConfigInfo.stateChangelogEnabled) &&
Objects.equals(this.timeout, checkpointConfigInfo.timeout) &&
Objects.equals(this.tolerableFailedCheckpoints, checkpointConfigInfo.tolerableFailedCheckpoints) &&
Objects.equals(this.unalignedCheckpoints, checkpointConfigInfo.unalignedCheckpoints);
}
@Override
public int hashCode() {
return Objects.hash(alignedCheckpointTimeout, changelogPeriodicMaterializationInterval, changelogStorage, checkpointStorage, checkpointsAfterTasksFinish, externalization, interval, maxConcurrent, minPause, mode, stateBackend, stateChangelogEnabled, timeout, tolerableFailedCheckpoints, unalignedCheckpoints);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CheckpointConfigInfo {\n");
sb.append(" alignedCheckpointTimeout: ").append(toIndentedString(alignedCheckpointTimeout)).append("\n");
sb.append(" changelogPeriodicMaterializationInterval: ").append(toIndentedString(changelogPeriodicMaterializationInterval)).append("\n");
sb.append(" changelogStorage: ").append(toIndentedString(changelogStorage)).append("\n");
sb.append(" checkpointStorage: ").append(toIndentedString(checkpointStorage)).append("\n");
sb.append(" checkpointsAfterTasksFinish: ").append(toIndentedString(checkpointsAfterTasksFinish)).append("\n");
sb.append(" externalization: ").append(toIndentedString(externalization)).append("\n");
sb.append(" interval: ").append(toIndentedString(interval)).append("\n");
sb.append(" maxConcurrent: ").append(toIndentedString(maxConcurrent)).append("\n");
sb.append(" minPause: ").append(toIndentedString(minPause)).append("\n");
sb.append(" mode: ").append(toIndentedString(mode)).append("\n");
sb.append(" stateBackend: ").append(toIndentedString(stateBackend)).append("\n");
sb.append(" stateChangelogEnabled: ").append(toIndentedString(stateChangelogEnabled)).append("\n");
sb.append(" timeout: ").append(toIndentedString(timeout)).append("\n");
sb.append(" tolerableFailedCheckpoints: ").append(toIndentedString(tolerableFailedCheckpoints)).append("\n");
sb.append(" unalignedCheckpoints: ").append(toIndentedString(unalignedCheckpoints)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy