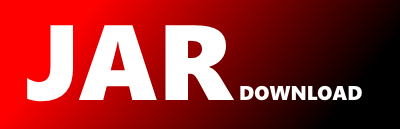
com.nextbreakpoint.flink.client.model.CheckpointStatisticsSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.StatsSummaryDto;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* CheckpointStatisticsSummary
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class CheckpointStatisticsSummary {
@SerializedName("alignment_buffered")
private StatsSummaryDto alignmentBuffered = null;
@SerializedName("checkpointed_size")
private StatsSummaryDto checkpointedSize = null;
@SerializedName("end_to_end_duration")
private StatsSummaryDto endToEndDuration = null;
@SerializedName("persisted_data")
private StatsSummaryDto persistedData = null;
@SerializedName("processed_data")
private StatsSummaryDto processedData = null;
@SerializedName("state_size")
private StatsSummaryDto stateSize = null;
public CheckpointStatisticsSummary alignmentBuffered(StatsSummaryDto alignmentBuffered) {
this.alignmentBuffered = alignmentBuffered;
return this;
}
/**
* Get alignmentBuffered
* @return alignmentBuffered
**/
@Schema(description = "")
public StatsSummaryDto getAlignmentBuffered() {
return alignmentBuffered;
}
public void setAlignmentBuffered(StatsSummaryDto alignmentBuffered) {
this.alignmentBuffered = alignmentBuffered;
}
public CheckpointStatisticsSummary checkpointedSize(StatsSummaryDto checkpointedSize) {
this.checkpointedSize = checkpointedSize;
return this;
}
/**
* Get checkpointedSize
* @return checkpointedSize
**/
@Schema(description = "")
public StatsSummaryDto getCheckpointedSize() {
return checkpointedSize;
}
public void setCheckpointedSize(StatsSummaryDto checkpointedSize) {
this.checkpointedSize = checkpointedSize;
}
public CheckpointStatisticsSummary endToEndDuration(StatsSummaryDto endToEndDuration) {
this.endToEndDuration = endToEndDuration;
return this;
}
/**
* Get endToEndDuration
* @return endToEndDuration
**/
@Schema(description = "")
public StatsSummaryDto getEndToEndDuration() {
return endToEndDuration;
}
public void setEndToEndDuration(StatsSummaryDto endToEndDuration) {
this.endToEndDuration = endToEndDuration;
}
public CheckpointStatisticsSummary persistedData(StatsSummaryDto persistedData) {
this.persistedData = persistedData;
return this;
}
/**
* Get persistedData
* @return persistedData
**/
@Schema(description = "")
public StatsSummaryDto getPersistedData() {
return persistedData;
}
public void setPersistedData(StatsSummaryDto persistedData) {
this.persistedData = persistedData;
}
public CheckpointStatisticsSummary processedData(StatsSummaryDto processedData) {
this.processedData = processedData;
return this;
}
/**
* Get processedData
* @return processedData
**/
@Schema(description = "")
public StatsSummaryDto getProcessedData() {
return processedData;
}
public void setProcessedData(StatsSummaryDto processedData) {
this.processedData = processedData;
}
public CheckpointStatisticsSummary stateSize(StatsSummaryDto stateSize) {
this.stateSize = stateSize;
return this;
}
/**
* Get stateSize
* @return stateSize
**/
@Schema(description = "")
public StatsSummaryDto getStateSize() {
return stateSize;
}
public void setStateSize(StatsSummaryDto stateSize) {
this.stateSize = stateSize;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CheckpointStatisticsSummary checkpointStatisticsSummary = (CheckpointStatisticsSummary) o;
return Objects.equals(this.alignmentBuffered, checkpointStatisticsSummary.alignmentBuffered) &&
Objects.equals(this.checkpointedSize, checkpointStatisticsSummary.checkpointedSize) &&
Objects.equals(this.endToEndDuration, checkpointStatisticsSummary.endToEndDuration) &&
Objects.equals(this.persistedData, checkpointStatisticsSummary.persistedData) &&
Objects.equals(this.processedData, checkpointStatisticsSummary.processedData) &&
Objects.equals(this.stateSize, checkpointStatisticsSummary.stateSize);
}
@Override
public int hashCode() {
return Objects.hash(alignmentBuffered, checkpointedSize, endToEndDuration, persistedData, processedData, stateSize);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CheckpointStatisticsSummary {\n");
sb.append(" alignmentBuffered: ").append(toIndentedString(alignmentBuffered)).append("\n");
sb.append(" checkpointedSize: ").append(toIndentedString(checkpointedSize)).append("\n");
sb.append(" endToEndDuration: ").append(toIndentedString(endToEndDuration)).append("\n");
sb.append(" persistedData: ").append(toIndentedString(persistedData)).append("\n");
sb.append(" processedData: ").append(toIndentedString(processedData)).append("\n");
sb.append(" stateSize: ").append(toIndentedString(stateSize)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy