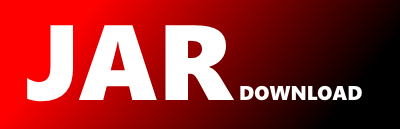
com.nextbreakpoint.flink.client.model.CompletedSubtaskCheckpointStatistics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.CheckpointAlignment;
import com.nextbreakpoint.flink.client.model.CheckpointDuration;
import com.nextbreakpoint.flink.client.model.SubtaskCheckpointStatistics;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* CompletedSubtaskCheckpointStatistics
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class CompletedSubtaskCheckpointStatistics extends SubtaskCheckpointStatistics {
@SerializedName("ack_timestamp")
private Long ackTimestamp = null;
@SerializedName("end_to_end_duration")
private Long endToEndDuration = null;
@SerializedName("checkpointed_size")
private Long checkpointedSize = null;
@SerializedName("state_size")
private Long stateSize = null;
@SerializedName("checkpoint")
private CheckpointDuration checkpoint = null;
@SerializedName("alignment")
private CheckpointAlignment alignment = null;
@SerializedName("start_delay")
private Long startDelay = null;
@SerializedName("unaligned_checkpoint")
private Boolean unalignedCheckpoint = null;
@SerializedName("aborted")
private Boolean aborted = null;
public CompletedSubtaskCheckpointStatistics ackTimestamp(Long ackTimestamp) {
this.ackTimestamp = ackTimestamp;
return this;
}
/**
* Get ackTimestamp
* @return ackTimestamp
**/
@Schema(description = "")
public Long getAckTimestamp() {
return ackTimestamp;
}
public void setAckTimestamp(Long ackTimestamp) {
this.ackTimestamp = ackTimestamp;
}
public CompletedSubtaskCheckpointStatistics endToEndDuration(Long endToEndDuration) {
this.endToEndDuration = endToEndDuration;
return this;
}
/**
* Get endToEndDuration
* @return endToEndDuration
**/
@Schema(description = "")
public Long getEndToEndDuration() {
return endToEndDuration;
}
public void setEndToEndDuration(Long endToEndDuration) {
this.endToEndDuration = endToEndDuration;
}
public CompletedSubtaskCheckpointStatistics checkpointedSize(Long checkpointedSize) {
this.checkpointedSize = checkpointedSize;
return this;
}
/**
* Get checkpointedSize
* @return checkpointedSize
**/
@Schema(description = "")
public Long getCheckpointedSize() {
return checkpointedSize;
}
public void setCheckpointedSize(Long checkpointedSize) {
this.checkpointedSize = checkpointedSize;
}
public CompletedSubtaskCheckpointStatistics stateSize(Long stateSize) {
this.stateSize = stateSize;
return this;
}
/**
* Get stateSize
* @return stateSize
**/
@Schema(description = "")
public Long getStateSize() {
return stateSize;
}
public void setStateSize(Long stateSize) {
this.stateSize = stateSize;
}
public CompletedSubtaskCheckpointStatistics checkpoint(CheckpointDuration checkpoint) {
this.checkpoint = checkpoint;
return this;
}
/**
* Get checkpoint
* @return checkpoint
**/
@Schema(description = "")
public CheckpointDuration getCheckpoint() {
return checkpoint;
}
public void setCheckpoint(CheckpointDuration checkpoint) {
this.checkpoint = checkpoint;
}
public CompletedSubtaskCheckpointStatistics alignment(CheckpointAlignment alignment) {
this.alignment = alignment;
return this;
}
/**
* Get alignment
* @return alignment
**/
@Schema(description = "")
public CheckpointAlignment getAlignment() {
return alignment;
}
public void setAlignment(CheckpointAlignment alignment) {
this.alignment = alignment;
}
public CompletedSubtaskCheckpointStatistics startDelay(Long startDelay) {
this.startDelay = startDelay;
return this;
}
/**
* Get startDelay
* @return startDelay
**/
@Schema(description = "")
public Long getStartDelay() {
return startDelay;
}
public void setStartDelay(Long startDelay) {
this.startDelay = startDelay;
}
public CompletedSubtaskCheckpointStatistics unalignedCheckpoint(Boolean unalignedCheckpoint) {
this.unalignedCheckpoint = unalignedCheckpoint;
return this;
}
/**
* Get unalignedCheckpoint
* @return unalignedCheckpoint
**/
@Schema(description = "")
public Boolean isUnalignedCheckpoint() {
return unalignedCheckpoint;
}
public void setUnalignedCheckpoint(Boolean unalignedCheckpoint) {
this.unalignedCheckpoint = unalignedCheckpoint;
}
public CompletedSubtaskCheckpointStatistics aborted(Boolean aborted) {
this.aborted = aborted;
return this;
}
/**
* Get aborted
* @return aborted
**/
@Schema(description = "")
public Boolean isAborted() {
return aborted;
}
public void setAborted(Boolean aborted) {
this.aborted = aborted;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CompletedSubtaskCheckpointStatistics completedSubtaskCheckpointStatistics = (CompletedSubtaskCheckpointStatistics) o;
return Objects.equals(this.ackTimestamp, completedSubtaskCheckpointStatistics.ackTimestamp) &&
Objects.equals(this.endToEndDuration, completedSubtaskCheckpointStatistics.endToEndDuration) &&
Objects.equals(this.checkpointedSize, completedSubtaskCheckpointStatistics.checkpointedSize) &&
Objects.equals(this.stateSize, completedSubtaskCheckpointStatistics.stateSize) &&
Objects.equals(this.checkpoint, completedSubtaskCheckpointStatistics.checkpoint) &&
Objects.equals(this.alignment, completedSubtaskCheckpointStatistics.alignment) &&
Objects.equals(this.startDelay, completedSubtaskCheckpointStatistics.startDelay) &&
Objects.equals(this.unalignedCheckpoint, completedSubtaskCheckpointStatistics.unalignedCheckpoint) &&
Objects.equals(this.aborted, completedSubtaskCheckpointStatistics.aborted) &&
super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(ackTimestamp, endToEndDuration, checkpointedSize, stateSize, checkpoint, alignment, startDelay, unalignedCheckpoint, aborted, super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CompletedSubtaskCheckpointStatistics {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" ackTimestamp: ").append(toIndentedString(ackTimestamp)).append("\n");
sb.append(" endToEndDuration: ").append(toIndentedString(endToEndDuration)).append("\n");
sb.append(" checkpointedSize: ").append(toIndentedString(checkpointedSize)).append("\n");
sb.append(" stateSize: ").append(toIndentedString(stateSize)).append("\n");
sb.append(" checkpoint: ").append(toIndentedString(checkpoint)).append("\n");
sb.append(" alignment: ").append(toIndentedString(alignment)).append("\n");
sb.append(" startDelay: ").append(toIndentedString(startDelay)).append("\n");
sb.append(" unalignedCheckpoint: ").append(toIndentedString(unalignedCheckpoint)).append("\n");
sb.append(" aborted: ").append(toIndentedString(aborted)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy