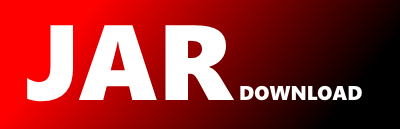
com.nextbreakpoint.flink.client.model.DashboardConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.Features;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* DashboardConfiguration
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class DashboardConfiguration {
@SerializedName("features")
private Features features = null;
@SerializedName("flink-revision")
private String flinkRevision = null;
@SerializedName("flink-version")
private String flinkVersion = null;
@SerializedName("refresh-interval")
private Long refreshInterval = null;
@SerializedName("timezone-name")
private String timezoneName = null;
@SerializedName("timezone-offset")
private Integer timezoneOffset = null;
public DashboardConfiguration features(Features features) {
this.features = features;
return this;
}
/**
* Get features
* @return features
**/
@Schema(description = "")
public Features getFeatures() {
return features;
}
public void setFeatures(Features features) {
this.features = features;
}
public DashboardConfiguration flinkRevision(String flinkRevision) {
this.flinkRevision = flinkRevision;
return this;
}
/**
* Get flinkRevision
* @return flinkRevision
**/
@Schema(description = "")
public String getFlinkRevision() {
return flinkRevision;
}
public void setFlinkRevision(String flinkRevision) {
this.flinkRevision = flinkRevision;
}
public DashboardConfiguration flinkVersion(String flinkVersion) {
this.flinkVersion = flinkVersion;
return this;
}
/**
* Get flinkVersion
* @return flinkVersion
**/
@Schema(description = "")
public String getFlinkVersion() {
return flinkVersion;
}
public void setFlinkVersion(String flinkVersion) {
this.flinkVersion = flinkVersion;
}
public DashboardConfiguration refreshInterval(Long refreshInterval) {
this.refreshInterval = refreshInterval;
return this;
}
/**
* Get refreshInterval
* @return refreshInterval
**/
@Schema(description = "")
public Long getRefreshInterval() {
return refreshInterval;
}
public void setRefreshInterval(Long refreshInterval) {
this.refreshInterval = refreshInterval;
}
public DashboardConfiguration timezoneName(String timezoneName) {
this.timezoneName = timezoneName;
return this;
}
/**
* Get timezoneName
* @return timezoneName
**/
@Schema(description = "")
public String getTimezoneName() {
return timezoneName;
}
public void setTimezoneName(String timezoneName) {
this.timezoneName = timezoneName;
}
public DashboardConfiguration timezoneOffset(Integer timezoneOffset) {
this.timezoneOffset = timezoneOffset;
return this;
}
/**
* Get timezoneOffset
* @return timezoneOffset
**/
@Schema(description = "")
public Integer getTimezoneOffset() {
return timezoneOffset;
}
public void setTimezoneOffset(Integer timezoneOffset) {
this.timezoneOffset = timezoneOffset;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DashboardConfiguration dashboardConfiguration = (DashboardConfiguration) o;
return Objects.equals(this.features, dashboardConfiguration.features) &&
Objects.equals(this.flinkRevision, dashboardConfiguration.flinkRevision) &&
Objects.equals(this.flinkVersion, dashboardConfiguration.flinkVersion) &&
Objects.equals(this.refreshInterval, dashboardConfiguration.refreshInterval) &&
Objects.equals(this.timezoneName, dashboardConfiguration.timezoneName) &&
Objects.equals(this.timezoneOffset, dashboardConfiguration.timezoneOffset);
}
@Override
public int hashCode() {
return Objects.hash(features, flinkRevision, flinkVersion, refreshInterval, timezoneName, timezoneOffset);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DashboardConfiguration {\n");
sb.append(" features: ").append(toIndentedString(features)).append("\n");
sb.append(" flinkRevision: ").append(toIndentedString(flinkRevision)).append("\n");
sb.append(" flinkVersion: ").append(toIndentedString(flinkVersion)).append("\n");
sb.append(" refreshInterval: ").append(toIndentedString(refreshInterval)).append("\n");
sb.append(" timezoneName: ").append(toIndentedString(timezoneName)).append("\n");
sb.append(" timezoneOffset: ").append(toIndentedString(timezoneOffset)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy