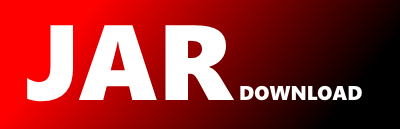
com.nextbreakpoint.flink.client.model.IOMetricsInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* IOMetricsInfo
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class IOMetricsInfo {
@SerializedName("accumulated-backpressured-time")
private Long accumulatedBackpressuredTime = null;
@SerializedName("accumulated-busy-time")
private Double accumulatedBusyTime = null;
@SerializedName("accumulated-idle-time")
private Long accumulatedIdleTime = null;
@SerializedName("read-bytes")
private Long readBytes = null;
@SerializedName("read-bytes-complete")
private Boolean readBytesComplete = null;
@SerializedName("read-records")
private Long readRecords = null;
@SerializedName("read-records-complete")
private Boolean readRecordsComplete = null;
@SerializedName("write-bytes")
private Long writeBytes = null;
@SerializedName("write-bytes-complete")
private Boolean writeBytesComplete = null;
@SerializedName("write-records")
private Long writeRecords = null;
@SerializedName("write-records-complete")
private Boolean writeRecordsComplete = null;
public IOMetricsInfo accumulatedBackpressuredTime(Long accumulatedBackpressuredTime) {
this.accumulatedBackpressuredTime = accumulatedBackpressuredTime;
return this;
}
/**
* Get accumulatedBackpressuredTime
* @return accumulatedBackpressuredTime
**/
@Schema(description = "")
public Long getAccumulatedBackpressuredTime() {
return accumulatedBackpressuredTime;
}
public void setAccumulatedBackpressuredTime(Long accumulatedBackpressuredTime) {
this.accumulatedBackpressuredTime = accumulatedBackpressuredTime;
}
public IOMetricsInfo accumulatedBusyTime(Double accumulatedBusyTime) {
this.accumulatedBusyTime = accumulatedBusyTime;
return this;
}
/**
* Get accumulatedBusyTime
* @return accumulatedBusyTime
**/
@Schema(description = "")
public Double getAccumulatedBusyTime() {
return accumulatedBusyTime;
}
public void setAccumulatedBusyTime(Double accumulatedBusyTime) {
this.accumulatedBusyTime = accumulatedBusyTime;
}
public IOMetricsInfo accumulatedIdleTime(Long accumulatedIdleTime) {
this.accumulatedIdleTime = accumulatedIdleTime;
return this;
}
/**
* Get accumulatedIdleTime
* @return accumulatedIdleTime
**/
@Schema(description = "")
public Long getAccumulatedIdleTime() {
return accumulatedIdleTime;
}
public void setAccumulatedIdleTime(Long accumulatedIdleTime) {
this.accumulatedIdleTime = accumulatedIdleTime;
}
public IOMetricsInfo readBytes(Long readBytes) {
this.readBytes = readBytes;
return this;
}
/**
* Get readBytes
* @return readBytes
**/
@Schema(description = "")
public Long getReadBytes() {
return readBytes;
}
public void setReadBytes(Long readBytes) {
this.readBytes = readBytes;
}
public IOMetricsInfo readBytesComplete(Boolean readBytesComplete) {
this.readBytesComplete = readBytesComplete;
return this;
}
/**
* Get readBytesComplete
* @return readBytesComplete
**/
@Schema(description = "")
public Boolean isReadBytesComplete() {
return readBytesComplete;
}
public void setReadBytesComplete(Boolean readBytesComplete) {
this.readBytesComplete = readBytesComplete;
}
public IOMetricsInfo readRecords(Long readRecords) {
this.readRecords = readRecords;
return this;
}
/**
* Get readRecords
* @return readRecords
**/
@Schema(description = "")
public Long getReadRecords() {
return readRecords;
}
public void setReadRecords(Long readRecords) {
this.readRecords = readRecords;
}
public IOMetricsInfo readRecordsComplete(Boolean readRecordsComplete) {
this.readRecordsComplete = readRecordsComplete;
return this;
}
/**
* Get readRecordsComplete
* @return readRecordsComplete
**/
@Schema(description = "")
public Boolean isReadRecordsComplete() {
return readRecordsComplete;
}
public void setReadRecordsComplete(Boolean readRecordsComplete) {
this.readRecordsComplete = readRecordsComplete;
}
public IOMetricsInfo writeBytes(Long writeBytes) {
this.writeBytes = writeBytes;
return this;
}
/**
* Get writeBytes
* @return writeBytes
**/
@Schema(description = "")
public Long getWriteBytes() {
return writeBytes;
}
public void setWriteBytes(Long writeBytes) {
this.writeBytes = writeBytes;
}
public IOMetricsInfo writeBytesComplete(Boolean writeBytesComplete) {
this.writeBytesComplete = writeBytesComplete;
return this;
}
/**
* Get writeBytesComplete
* @return writeBytesComplete
**/
@Schema(description = "")
public Boolean isWriteBytesComplete() {
return writeBytesComplete;
}
public void setWriteBytesComplete(Boolean writeBytesComplete) {
this.writeBytesComplete = writeBytesComplete;
}
public IOMetricsInfo writeRecords(Long writeRecords) {
this.writeRecords = writeRecords;
return this;
}
/**
* Get writeRecords
* @return writeRecords
**/
@Schema(description = "")
public Long getWriteRecords() {
return writeRecords;
}
public void setWriteRecords(Long writeRecords) {
this.writeRecords = writeRecords;
}
public IOMetricsInfo writeRecordsComplete(Boolean writeRecordsComplete) {
this.writeRecordsComplete = writeRecordsComplete;
return this;
}
/**
* Get writeRecordsComplete
* @return writeRecordsComplete
**/
@Schema(description = "")
public Boolean isWriteRecordsComplete() {
return writeRecordsComplete;
}
public void setWriteRecordsComplete(Boolean writeRecordsComplete) {
this.writeRecordsComplete = writeRecordsComplete;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IOMetricsInfo ioMetricsInfo = (IOMetricsInfo) o;
return Objects.equals(this.accumulatedBackpressuredTime, ioMetricsInfo.accumulatedBackpressuredTime) &&
Objects.equals(this.accumulatedBusyTime, ioMetricsInfo.accumulatedBusyTime) &&
Objects.equals(this.accumulatedIdleTime, ioMetricsInfo.accumulatedIdleTime) &&
Objects.equals(this.readBytes, ioMetricsInfo.readBytes) &&
Objects.equals(this.readBytesComplete, ioMetricsInfo.readBytesComplete) &&
Objects.equals(this.readRecords, ioMetricsInfo.readRecords) &&
Objects.equals(this.readRecordsComplete, ioMetricsInfo.readRecordsComplete) &&
Objects.equals(this.writeBytes, ioMetricsInfo.writeBytes) &&
Objects.equals(this.writeBytesComplete, ioMetricsInfo.writeBytesComplete) &&
Objects.equals(this.writeRecords, ioMetricsInfo.writeRecords) &&
Objects.equals(this.writeRecordsComplete, ioMetricsInfo.writeRecordsComplete);
}
@Override
public int hashCode() {
return Objects.hash(accumulatedBackpressuredTime, accumulatedBusyTime, accumulatedIdleTime, readBytes, readBytesComplete, readRecords, readRecordsComplete, writeBytes, writeBytesComplete, writeRecords, writeRecordsComplete);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class IOMetricsInfo {\n");
sb.append(" accumulatedBackpressuredTime: ").append(toIndentedString(accumulatedBackpressuredTime)).append("\n");
sb.append(" accumulatedBusyTime: ").append(toIndentedString(accumulatedBusyTime)).append("\n");
sb.append(" accumulatedIdleTime: ").append(toIndentedString(accumulatedIdleTime)).append("\n");
sb.append(" readBytes: ").append(toIndentedString(readBytes)).append("\n");
sb.append(" readBytesComplete: ").append(toIndentedString(readBytesComplete)).append("\n");
sb.append(" readRecords: ").append(toIndentedString(readRecords)).append("\n");
sb.append(" readRecordsComplete: ").append(toIndentedString(readRecordsComplete)).append("\n");
sb.append(" writeBytes: ").append(toIndentedString(writeBytes)).append("\n");
sb.append(" writeBytesComplete: ").append(toIndentedString(writeBytesComplete)).append("\n");
sb.append(" writeRecords: ").append(toIndentedString(writeRecords)).append("\n");
sb.append(" writeRecordsComplete: ").append(toIndentedString(writeRecordsComplete)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy