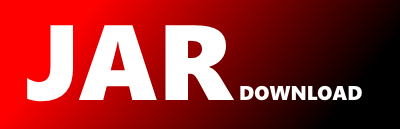
com.nextbreakpoint.flink.client.model.JobDetailsVertexInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.ExecutionState;
import com.nextbreakpoint.flink.client.model.IOMetricsInfo;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* JobDetailsVertexInfo
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class JobDetailsVertexInfo {
@SerializedName("duration")
private Long duration = null;
@SerializedName("end-time")
private Long endTime = null;
@SerializedName("id")
private String id = null;
@SerializedName("maxParallelism")
private Integer maxParallelism = null;
@SerializedName("metrics")
private IOMetricsInfo metrics = null;
@SerializedName("name")
private String name = null;
@SerializedName("parallelism")
private Integer parallelism = null;
@SerializedName("slotSharingGroupId")
private String slotSharingGroupId = null;
@SerializedName("start-time")
private Long startTime = null;
@SerializedName("status")
private ExecutionState status = null;
@SerializedName("tasks")
private Map tasks = null;
public JobDetailsVertexInfo duration(Long duration) {
this.duration = duration;
return this;
}
/**
* Get duration
* @return duration
**/
@Schema(description = "")
public Long getDuration() {
return duration;
}
public void setDuration(Long duration) {
this.duration = duration;
}
public JobDetailsVertexInfo endTime(Long endTime) {
this.endTime = endTime;
return this;
}
/**
* Get endTime
* @return endTime
**/
@Schema(description = "")
public Long getEndTime() {
return endTime;
}
public void setEndTime(Long endTime) {
this.endTime = endTime;
}
public JobDetailsVertexInfo id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@Schema(description = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public JobDetailsVertexInfo maxParallelism(Integer maxParallelism) {
this.maxParallelism = maxParallelism;
return this;
}
/**
* Get maxParallelism
* @return maxParallelism
**/
@Schema(description = "")
public Integer getMaxParallelism() {
return maxParallelism;
}
public void setMaxParallelism(Integer maxParallelism) {
this.maxParallelism = maxParallelism;
}
public JobDetailsVertexInfo metrics(IOMetricsInfo metrics) {
this.metrics = metrics;
return this;
}
/**
* Get metrics
* @return metrics
**/
@Schema(description = "")
public IOMetricsInfo getMetrics() {
return metrics;
}
public void setMetrics(IOMetricsInfo metrics) {
this.metrics = metrics;
}
public JobDetailsVertexInfo name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@Schema(description = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public JobDetailsVertexInfo parallelism(Integer parallelism) {
this.parallelism = parallelism;
return this;
}
/**
* Get parallelism
* @return parallelism
**/
@Schema(description = "")
public Integer getParallelism() {
return parallelism;
}
public void setParallelism(Integer parallelism) {
this.parallelism = parallelism;
}
public JobDetailsVertexInfo slotSharingGroupId(String slotSharingGroupId) {
this.slotSharingGroupId = slotSharingGroupId;
return this;
}
/**
* Get slotSharingGroupId
* @return slotSharingGroupId
**/
@Schema(description = "")
public String getSlotSharingGroupId() {
return slotSharingGroupId;
}
public void setSlotSharingGroupId(String slotSharingGroupId) {
this.slotSharingGroupId = slotSharingGroupId;
}
public JobDetailsVertexInfo startTime(Long startTime) {
this.startTime = startTime;
return this;
}
/**
* Get startTime
* @return startTime
**/
@Schema(description = "")
public Long getStartTime() {
return startTime;
}
public void setStartTime(Long startTime) {
this.startTime = startTime;
}
public JobDetailsVertexInfo status(ExecutionState status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public ExecutionState getStatus() {
return status;
}
public void setStatus(ExecutionState status) {
this.status = status;
}
public JobDetailsVertexInfo tasks(Map tasks) {
this.tasks = tasks;
return this;
}
public JobDetailsVertexInfo putTasksItem(String key, Integer tasksItem) {
if (this.tasks == null) {
this.tasks = new HashMap<>();
}
this.tasks.put(key, tasksItem);
return this;
}
/**
* Get tasks
* @return tasks
**/
@Schema(description = "")
public Map getTasks() {
return tasks;
}
public void setTasks(Map tasks) {
this.tasks = tasks;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
JobDetailsVertexInfo jobDetailsVertexInfo = (JobDetailsVertexInfo) o;
return Objects.equals(this.duration, jobDetailsVertexInfo.duration) &&
Objects.equals(this.endTime, jobDetailsVertexInfo.endTime) &&
Objects.equals(this.id, jobDetailsVertexInfo.id) &&
Objects.equals(this.maxParallelism, jobDetailsVertexInfo.maxParallelism) &&
Objects.equals(this.metrics, jobDetailsVertexInfo.metrics) &&
Objects.equals(this.name, jobDetailsVertexInfo.name) &&
Objects.equals(this.parallelism, jobDetailsVertexInfo.parallelism) &&
Objects.equals(this.slotSharingGroupId, jobDetailsVertexInfo.slotSharingGroupId) &&
Objects.equals(this.startTime, jobDetailsVertexInfo.startTime) &&
Objects.equals(this.status, jobDetailsVertexInfo.status) &&
Objects.equals(this.tasks, jobDetailsVertexInfo.tasks);
}
@Override
public int hashCode() {
return Objects.hash(duration, endTime, id, maxParallelism, metrics, name, parallelism, slotSharingGroupId, startTime, status, tasks);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class JobDetailsVertexInfo {\n");
sb.append(" duration: ").append(toIndentedString(duration)).append("\n");
sb.append(" endTime: ").append(toIndentedString(endTime)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" maxParallelism: ").append(toIndentedString(maxParallelism)).append("\n");
sb.append(" metrics: ").append(toIndentedString(metrics)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" parallelism: ").append(toIndentedString(parallelism)).append("\n");
sb.append(" slotSharingGroupId: ").append(toIndentedString(slotSharingGroupId)).append("\n");
sb.append(" startTime: ").append(toIndentedString(startTime)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tasks: ").append(toIndentedString(tasks)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy