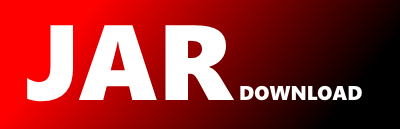
com.nextbreakpoint.flink.client.model.SubtaskBackPressureInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.SubtaskBackPressureInfo;
import com.nextbreakpoint.flink.client.model.VertexBackPressureLevel;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* SubtaskBackPressureInfo
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class SubtaskBackPressureInfo {
@SerializedName("attempt-number")
private Integer attemptNumber = null;
@SerializedName("backpressureLevel")
private VertexBackPressureLevel backpressureLevel = null;
@SerializedName("busyRatio")
private Double busyRatio = null;
@SerializedName("idleRatio")
private Double idleRatio = null;
@SerializedName("other-concurrent-attempts")
private List otherConcurrentAttempts = null;
@SerializedName("ratio")
private Double ratio = null;
@SerializedName("subtask")
private Integer subtask = null;
public SubtaskBackPressureInfo attemptNumber(Integer attemptNumber) {
this.attemptNumber = attemptNumber;
return this;
}
/**
* Get attemptNumber
* @return attemptNumber
**/
@Schema(description = "")
public Integer getAttemptNumber() {
return attemptNumber;
}
public void setAttemptNumber(Integer attemptNumber) {
this.attemptNumber = attemptNumber;
}
public SubtaskBackPressureInfo backpressureLevel(VertexBackPressureLevel backpressureLevel) {
this.backpressureLevel = backpressureLevel;
return this;
}
/**
* Get backpressureLevel
* @return backpressureLevel
**/
@Schema(description = "")
public VertexBackPressureLevel getBackpressureLevel() {
return backpressureLevel;
}
public void setBackpressureLevel(VertexBackPressureLevel backpressureLevel) {
this.backpressureLevel = backpressureLevel;
}
public SubtaskBackPressureInfo busyRatio(Double busyRatio) {
this.busyRatio = busyRatio;
return this;
}
/**
* Get busyRatio
* @return busyRatio
**/
@Schema(description = "")
public Double getBusyRatio() {
return busyRatio;
}
public void setBusyRatio(Double busyRatio) {
this.busyRatio = busyRatio;
}
public SubtaskBackPressureInfo idleRatio(Double idleRatio) {
this.idleRatio = idleRatio;
return this;
}
/**
* Get idleRatio
* @return idleRatio
**/
@Schema(description = "")
public Double getIdleRatio() {
return idleRatio;
}
public void setIdleRatio(Double idleRatio) {
this.idleRatio = idleRatio;
}
public SubtaskBackPressureInfo otherConcurrentAttempts(List otherConcurrentAttempts) {
this.otherConcurrentAttempts = otherConcurrentAttempts;
return this;
}
public SubtaskBackPressureInfo addOtherConcurrentAttemptsItem(SubtaskBackPressureInfo otherConcurrentAttemptsItem) {
if (this.otherConcurrentAttempts == null) {
this.otherConcurrentAttempts = new ArrayList<>();
}
this.otherConcurrentAttempts.add(otherConcurrentAttemptsItem);
return this;
}
/**
* Get otherConcurrentAttempts
* @return otherConcurrentAttempts
**/
@Schema(description = "")
public List getOtherConcurrentAttempts() {
return otherConcurrentAttempts;
}
public void setOtherConcurrentAttempts(List otherConcurrentAttempts) {
this.otherConcurrentAttempts = otherConcurrentAttempts;
}
public SubtaskBackPressureInfo ratio(Double ratio) {
this.ratio = ratio;
return this;
}
/**
* Get ratio
* @return ratio
**/
@Schema(description = "")
public Double getRatio() {
return ratio;
}
public void setRatio(Double ratio) {
this.ratio = ratio;
}
public SubtaskBackPressureInfo subtask(Integer subtask) {
this.subtask = subtask;
return this;
}
/**
* Get subtask
* @return subtask
**/
@Schema(description = "")
public Integer getSubtask() {
return subtask;
}
public void setSubtask(Integer subtask) {
this.subtask = subtask;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubtaskBackPressureInfo subtaskBackPressureInfo = (SubtaskBackPressureInfo) o;
return Objects.equals(this.attemptNumber, subtaskBackPressureInfo.attemptNumber) &&
Objects.equals(this.backpressureLevel, subtaskBackPressureInfo.backpressureLevel) &&
Objects.equals(this.busyRatio, subtaskBackPressureInfo.busyRatio) &&
Objects.equals(this.idleRatio, subtaskBackPressureInfo.idleRatio) &&
Objects.equals(this.otherConcurrentAttempts, subtaskBackPressureInfo.otherConcurrentAttempts) &&
Objects.equals(this.ratio, subtaskBackPressureInfo.ratio) &&
Objects.equals(this.subtask, subtaskBackPressureInfo.subtask);
}
@Override
public int hashCode() {
return Objects.hash(attemptNumber, backpressureLevel, busyRatio, idleRatio, otherConcurrentAttempts, ratio, subtask);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubtaskBackPressureInfo {\n");
sb.append(" attemptNumber: ").append(toIndentedString(attemptNumber)).append("\n");
sb.append(" backpressureLevel: ").append(toIndentedString(backpressureLevel)).append("\n");
sb.append(" busyRatio: ").append(toIndentedString(busyRatio)).append("\n");
sb.append(" idleRatio: ").append(toIndentedString(idleRatio)).append("\n");
sb.append(" otherConcurrentAttempts: ").append(toIndentedString(otherConcurrentAttempts)).append("\n");
sb.append(" ratio: ").append(toIndentedString(ratio)).append("\n");
sb.append(" subtask: ").append(toIndentedString(subtask)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy