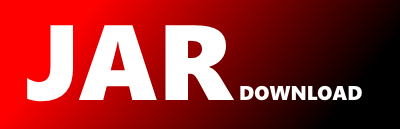
com.nextbreakpoint.flink.client.model.SubtaskExecutionAttemptDetailsInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.ExecutionState;
import com.nextbreakpoint.flink.client.model.IOMetricsInfo;
import com.nextbreakpoint.flink.client.model.SubtaskExecutionAttemptDetailsInfo;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* SubtaskExecutionAttemptDetailsInfo
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class SubtaskExecutionAttemptDetailsInfo {
@SerializedName("attempt")
private Integer attempt = null;
@SerializedName("duration")
private Long duration = null;
@SerializedName("end-time")
private Long endTime = null;
@SerializedName("endpoint")
private String endpoint = null;
@SerializedName("host")
private String host = null;
@SerializedName("metrics")
private IOMetricsInfo metrics = null;
@SerializedName("other-concurrent-attempts")
private List otherConcurrentAttempts = null;
@SerializedName("start-time")
private Long startTime = null;
@SerializedName("status")
private ExecutionState status = null;
@SerializedName("status-duration")
private Map statusDuration = null;
@SerializedName("subtask")
private Integer subtask = null;
@SerializedName("taskmanager-id")
private String taskmanagerId = null;
public SubtaskExecutionAttemptDetailsInfo attempt(Integer attempt) {
this.attempt = attempt;
return this;
}
/**
* Get attempt
* @return attempt
**/
@Schema(description = "")
public Integer getAttempt() {
return attempt;
}
public void setAttempt(Integer attempt) {
this.attempt = attempt;
}
public SubtaskExecutionAttemptDetailsInfo duration(Long duration) {
this.duration = duration;
return this;
}
/**
* Get duration
* @return duration
**/
@Schema(description = "")
public Long getDuration() {
return duration;
}
public void setDuration(Long duration) {
this.duration = duration;
}
public SubtaskExecutionAttemptDetailsInfo endTime(Long endTime) {
this.endTime = endTime;
return this;
}
/**
* Get endTime
* @return endTime
**/
@Schema(description = "")
public Long getEndTime() {
return endTime;
}
public void setEndTime(Long endTime) {
this.endTime = endTime;
}
public SubtaskExecutionAttemptDetailsInfo endpoint(String endpoint) {
this.endpoint = endpoint;
return this;
}
/**
* Get endpoint
* @return endpoint
**/
@Schema(description = "")
public String getEndpoint() {
return endpoint;
}
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
public SubtaskExecutionAttemptDetailsInfo host(String host) {
this.host = host;
return this;
}
/**
* Get host
* @return host
**/
@Schema(description = "")
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public SubtaskExecutionAttemptDetailsInfo metrics(IOMetricsInfo metrics) {
this.metrics = metrics;
return this;
}
/**
* Get metrics
* @return metrics
**/
@Schema(description = "")
public IOMetricsInfo getMetrics() {
return metrics;
}
public void setMetrics(IOMetricsInfo metrics) {
this.metrics = metrics;
}
public SubtaskExecutionAttemptDetailsInfo otherConcurrentAttempts(List otherConcurrentAttempts) {
this.otherConcurrentAttempts = otherConcurrentAttempts;
return this;
}
public SubtaskExecutionAttemptDetailsInfo addOtherConcurrentAttemptsItem(SubtaskExecutionAttemptDetailsInfo otherConcurrentAttemptsItem) {
if (this.otherConcurrentAttempts == null) {
this.otherConcurrentAttempts = new ArrayList<>();
}
this.otherConcurrentAttempts.add(otherConcurrentAttemptsItem);
return this;
}
/**
* Get otherConcurrentAttempts
* @return otherConcurrentAttempts
**/
@Schema(description = "")
public List getOtherConcurrentAttempts() {
return otherConcurrentAttempts;
}
public void setOtherConcurrentAttempts(List otherConcurrentAttempts) {
this.otherConcurrentAttempts = otherConcurrentAttempts;
}
public SubtaskExecutionAttemptDetailsInfo startTime(Long startTime) {
this.startTime = startTime;
return this;
}
/**
* Get startTime
* @return startTime
**/
@Schema(description = "")
public Long getStartTime() {
return startTime;
}
public void setStartTime(Long startTime) {
this.startTime = startTime;
}
public SubtaskExecutionAttemptDetailsInfo status(ExecutionState status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public ExecutionState getStatus() {
return status;
}
public void setStatus(ExecutionState status) {
this.status = status;
}
public SubtaskExecutionAttemptDetailsInfo statusDuration(Map statusDuration) {
this.statusDuration = statusDuration;
return this;
}
public SubtaskExecutionAttemptDetailsInfo putStatusDurationItem(String key, Long statusDurationItem) {
if (this.statusDuration == null) {
this.statusDuration = new HashMap<>();
}
this.statusDuration.put(key, statusDurationItem);
return this;
}
/**
* Get statusDuration
* @return statusDuration
**/
@Schema(description = "")
public Map getStatusDuration() {
return statusDuration;
}
public void setStatusDuration(Map statusDuration) {
this.statusDuration = statusDuration;
}
public SubtaskExecutionAttemptDetailsInfo subtask(Integer subtask) {
this.subtask = subtask;
return this;
}
/**
* Get subtask
* @return subtask
**/
@Schema(description = "")
public Integer getSubtask() {
return subtask;
}
public void setSubtask(Integer subtask) {
this.subtask = subtask;
}
public SubtaskExecutionAttemptDetailsInfo taskmanagerId(String taskmanagerId) {
this.taskmanagerId = taskmanagerId;
return this;
}
/**
* Get taskmanagerId
* @return taskmanagerId
**/
@Schema(description = "")
public String getTaskmanagerId() {
return taskmanagerId;
}
public void setTaskmanagerId(String taskmanagerId) {
this.taskmanagerId = taskmanagerId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubtaskExecutionAttemptDetailsInfo subtaskExecutionAttemptDetailsInfo = (SubtaskExecutionAttemptDetailsInfo) o;
return Objects.equals(this.attempt, subtaskExecutionAttemptDetailsInfo.attempt) &&
Objects.equals(this.duration, subtaskExecutionAttemptDetailsInfo.duration) &&
Objects.equals(this.endTime, subtaskExecutionAttemptDetailsInfo.endTime) &&
Objects.equals(this.endpoint, subtaskExecutionAttemptDetailsInfo.endpoint) &&
Objects.equals(this.host, subtaskExecutionAttemptDetailsInfo.host) &&
Objects.equals(this.metrics, subtaskExecutionAttemptDetailsInfo.metrics) &&
Objects.equals(this.otherConcurrentAttempts, subtaskExecutionAttemptDetailsInfo.otherConcurrentAttempts) &&
Objects.equals(this.startTime, subtaskExecutionAttemptDetailsInfo.startTime) &&
Objects.equals(this.status, subtaskExecutionAttemptDetailsInfo.status) &&
Objects.equals(this.statusDuration, subtaskExecutionAttemptDetailsInfo.statusDuration) &&
Objects.equals(this.subtask, subtaskExecutionAttemptDetailsInfo.subtask) &&
Objects.equals(this.taskmanagerId, subtaskExecutionAttemptDetailsInfo.taskmanagerId);
}
@Override
public int hashCode() {
return Objects.hash(attempt, duration, endTime, endpoint, host, metrics, otherConcurrentAttempts, startTime, status, statusDuration, subtask, taskmanagerId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubtaskExecutionAttemptDetailsInfo {\n");
sb.append(" attempt: ").append(toIndentedString(attempt)).append("\n");
sb.append(" duration: ").append(toIndentedString(duration)).append("\n");
sb.append(" endTime: ").append(toIndentedString(endTime)).append("\n");
sb.append(" endpoint: ").append(toIndentedString(endpoint)).append("\n");
sb.append(" host: ").append(toIndentedString(host)).append("\n");
sb.append(" metrics: ").append(toIndentedString(metrics)).append("\n");
sb.append(" otherConcurrentAttempts: ").append(toIndentedString(otherConcurrentAttempts)).append("\n");
sb.append(" startTime: ").append(toIndentedString(startTime)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" statusDuration: ").append(toIndentedString(statusDuration)).append("\n");
sb.append(" subtask: ").append(toIndentedString(subtask)).append("\n");
sb.append(" taskmanagerId: ").append(toIndentedString(taskmanagerId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy