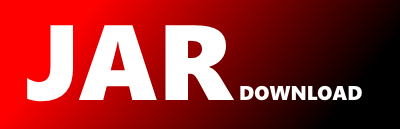
com.nextbreakpoint.flink.client.model.TaskCheckpointStatisticsWithSubtaskDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.CheckpointStatsStatus;
import com.nextbreakpoint.flink.client.model.SubtaskCheckpointStatistics;
import com.nextbreakpoint.flink.client.model.TaskCheckpointStatisticsWithSubtaskDetailsSummary;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* TaskCheckpointStatisticsWithSubtaskDetails
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class TaskCheckpointStatisticsWithSubtaskDetails {
@SerializedName("alignment_buffered")
private Long alignmentBuffered = null;
@SerializedName("checkpointed_size")
private Long checkpointedSize = null;
@SerializedName("end_to_end_duration")
private Long endToEndDuration = null;
@SerializedName("id")
private Long id = null;
@SerializedName("latest_ack_timestamp")
private Long latestAckTimestamp = null;
@SerializedName("num_acknowledged_subtasks")
private Integer numAcknowledgedSubtasks = null;
@SerializedName("num_subtasks")
private Integer numSubtasks = null;
@SerializedName("persisted_data")
private Long persistedData = null;
@SerializedName("processed_data")
private Long processedData = null;
@SerializedName("state_size")
private Long stateSize = null;
@SerializedName("status")
private CheckpointStatsStatus status = null;
@SerializedName("subtasks")
private List subtasks = null;
@SerializedName("summary")
private TaskCheckpointStatisticsWithSubtaskDetailsSummary summary = null;
public TaskCheckpointStatisticsWithSubtaskDetails alignmentBuffered(Long alignmentBuffered) {
this.alignmentBuffered = alignmentBuffered;
return this;
}
/**
* Get alignmentBuffered
* @return alignmentBuffered
**/
@Schema(description = "")
public Long getAlignmentBuffered() {
return alignmentBuffered;
}
public void setAlignmentBuffered(Long alignmentBuffered) {
this.alignmentBuffered = alignmentBuffered;
}
public TaskCheckpointStatisticsWithSubtaskDetails checkpointedSize(Long checkpointedSize) {
this.checkpointedSize = checkpointedSize;
return this;
}
/**
* Get checkpointedSize
* @return checkpointedSize
**/
@Schema(description = "")
public Long getCheckpointedSize() {
return checkpointedSize;
}
public void setCheckpointedSize(Long checkpointedSize) {
this.checkpointedSize = checkpointedSize;
}
public TaskCheckpointStatisticsWithSubtaskDetails endToEndDuration(Long endToEndDuration) {
this.endToEndDuration = endToEndDuration;
return this;
}
/**
* Get endToEndDuration
* @return endToEndDuration
**/
@Schema(description = "")
public Long getEndToEndDuration() {
return endToEndDuration;
}
public void setEndToEndDuration(Long endToEndDuration) {
this.endToEndDuration = endToEndDuration;
}
public TaskCheckpointStatisticsWithSubtaskDetails id(Long id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@Schema(description = "")
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public TaskCheckpointStatisticsWithSubtaskDetails latestAckTimestamp(Long latestAckTimestamp) {
this.latestAckTimestamp = latestAckTimestamp;
return this;
}
/**
* Get latestAckTimestamp
* @return latestAckTimestamp
**/
@Schema(description = "")
public Long getLatestAckTimestamp() {
return latestAckTimestamp;
}
public void setLatestAckTimestamp(Long latestAckTimestamp) {
this.latestAckTimestamp = latestAckTimestamp;
}
public TaskCheckpointStatisticsWithSubtaskDetails numAcknowledgedSubtasks(Integer numAcknowledgedSubtasks) {
this.numAcknowledgedSubtasks = numAcknowledgedSubtasks;
return this;
}
/**
* Get numAcknowledgedSubtasks
* @return numAcknowledgedSubtasks
**/
@Schema(description = "")
public Integer getNumAcknowledgedSubtasks() {
return numAcknowledgedSubtasks;
}
public void setNumAcknowledgedSubtasks(Integer numAcknowledgedSubtasks) {
this.numAcknowledgedSubtasks = numAcknowledgedSubtasks;
}
public TaskCheckpointStatisticsWithSubtaskDetails numSubtasks(Integer numSubtasks) {
this.numSubtasks = numSubtasks;
return this;
}
/**
* Get numSubtasks
* @return numSubtasks
**/
@Schema(description = "")
public Integer getNumSubtasks() {
return numSubtasks;
}
public void setNumSubtasks(Integer numSubtasks) {
this.numSubtasks = numSubtasks;
}
public TaskCheckpointStatisticsWithSubtaskDetails persistedData(Long persistedData) {
this.persistedData = persistedData;
return this;
}
/**
* Get persistedData
* @return persistedData
**/
@Schema(description = "")
public Long getPersistedData() {
return persistedData;
}
public void setPersistedData(Long persistedData) {
this.persistedData = persistedData;
}
public TaskCheckpointStatisticsWithSubtaskDetails processedData(Long processedData) {
this.processedData = processedData;
return this;
}
/**
* Get processedData
* @return processedData
**/
@Schema(description = "")
public Long getProcessedData() {
return processedData;
}
public void setProcessedData(Long processedData) {
this.processedData = processedData;
}
public TaskCheckpointStatisticsWithSubtaskDetails stateSize(Long stateSize) {
this.stateSize = stateSize;
return this;
}
/**
* Get stateSize
* @return stateSize
**/
@Schema(description = "")
public Long getStateSize() {
return stateSize;
}
public void setStateSize(Long stateSize) {
this.stateSize = stateSize;
}
public TaskCheckpointStatisticsWithSubtaskDetails status(CheckpointStatsStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public CheckpointStatsStatus getStatus() {
return status;
}
public void setStatus(CheckpointStatsStatus status) {
this.status = status;
}
public TaskCheckpointStatisticsWithSubtaskDetails subtasks(List subtasks) {
this.subtasks = subtasks;
return this;
}
public TaskCheckpointStatisticsWithSubtaskDetails addSubtasksItem(SubtaskCheckpointStatistics subtasksItem) {
if (this.subtasks == null) {
this.subtasks = new ArrayList<>();
}
this.subtasks.add(subtasksItem);
return this;
}
/**
* Get subtasks
* @return subtasks
**/
@Schema(description = "")
public List getSubtasks() {
return subtasks;
}
public void setSubtasks(List subtasks) {
this.subtasks = subtasks;
}
public TaskCheckpointStatisticsWithSubtaskDetails summary(TaskCheckpointStatisticsWithSubtaskDetailsSummary summary) {
this.summary = summary;
return this;
}
/**
* Get summary
* @return summary
**/
@Schema(description = "")
public TaskCheckpointStatisticsWithSubtaskDetailsSummary getSummary() {
return summary;
}
public void setSummary(TaskCheckpointStatisticsWithSubtaskDetailsSummary summary) {
this.summary = summary;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskCheckpointStatisticsWithSubtaskDetails taskCheckpointStatisticsWithSubtaskDetails = (TaskCheckpointStatisticsWithSubtaskDetails) o;
return Objects.equals(this.alignmentBuffered, taskCheckpointStatisticsWithSubtaskDetails.alignmentBuffered) &&
Objects.equals(this.checkpointedSize, taskCheckpointStatisticsWithSubtaskDetails.checkpointedSize) &&
Objects.equals(this.endToEndDuration, taskCheckpointStatisticsWithSubtaskDetails.endToEndDuration) &&
Objects.equals(this.id, taskCheckpointStatisticsWithSubtaskDetails.id) &&
Objects.equals(this.latestAckTimestamp, taskCheckpointStatisticsWithSubtaskDetails.latestAckTimestamp) &&
Objects.equals(this.numAcknowledgedSubtasks, taskCheckpointStatisticsWithSubtaskDetails.numAcknowledgedSubtasks) &&
Objects.equals(this.numSubtasks, taskCheckpointStatisticsWithSubtaskDetails.numSubtasks) &&
Objects.equals(this.persistedData, taskCheckpointStatisticsWithSubtaskDetails.persistedData) &&
Objects.equals(this.processedData, taskCheckpointStatisticsWithSubtaskDetails.processedData) &&
Objects.equals(this.stateSize, taskCheckpointStatisticsWithSubtaskDetails.stateSize) &&
Objects.equals(this.status, taskCheckpointStatisticsWithSubtaskDetails.status) &&
Objects.equals(this.subtasks, taskCheckpointStatisticsWithSubtaskDetails.subtasks) &&
Objects.equals(this.summary, taskCheckpointStatisticsWithSubtaskDetails.summary);
}
@Override
public int hashCode() {
return Objects.hash(alignmentBuffered, checkpointedSize, endToEndDuration, id, latestAckTimestamp, numAcknowledgedSubtasks, numSubtasks, persistedData, processedData, stateSize, status, subtasks, summary);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskCheckpointStatisticsWithSubtaskDetails {\n");
sb.append(" alignmentBuffered: ").append(toIndentedString(alignmentBuffered)).append("\n");
sb.append(" checkpointedSize: ").append(toIndentedString(checkpointedSize)).append("\n");
sb.append(" endToEndDuration: ").append(toIndentedString(endToEndDuration)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" latestAckTimestamp: ").append(toIndentedString(latestAckTimestamp)).append("\n");
sb.append(" numAcknowledgedSubtasks: ").append(toIndentedString(numAcknowledgedSubtasks)).append("\n");
sb.append(" numSubtasks: ").append(toIndentedString(numSubtasks)).append("\n");
sb.append(" persistedData: ").append(toIndentedString(persistedData)).append("\n");
sb.append(" processedData: ").append(toIndentedString(processedData)).append("\n");
sb.append(" stateSize: ").append(toIndentedString(stateSize)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" subtasks: ").append(toIndentedString(subtasks)).append("\n");
sb.append(" summary: ").append(toIndentedString(summary)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy