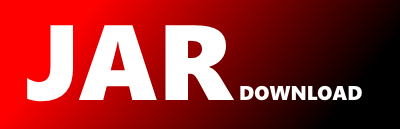
com.nextbreakpoint.flink.client.model.TaskCheckpointStatisticsWithSubtaskDetailsSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.CheckpointAlignmentSummary;
import com.nextbreakpoint.flink.client.model.CheckpointDurationSummary;
import com.nextbreakpoint.flink.client.model.StatsSummaryDto;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* TaskCheckpointStatisticsWithSubtaskDetailsSummary
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class TaskCheckpointStatisticsWithSubtaskDetailsSummary {
@SerializedName("alignment")
private CheckpointAlignmentSummary alignment = null;
@SerializedName("checkpoint_duration")
private CheckpointDurationSummary checkpointDuration = null;
@SerializedName("checkpointed_size")
private StatsSummaryDto checkpointedSize = null;
@SerializedName("end_to_end_duration")
private StatsSummaryDto endToEndDuration = null;
@SerializedName("start_delay")
private StatsSummaryDto startDelay = null;
@SerializedName("state_size")
private StatsSummaryDto stateSize = null;
public TaskCheckpointStatisticsWithSubtaskDetailsSummary alignment(CheckpointAlignmentSummary alignment) {
this.alignment = alignment;
return this;
}
/**
* Get alignment
* @return alignment
**/
@Schema(description = "")
public CheckpointAlignmentSummary getAlignment() {
return alignment;
}
public void setAlignment(CheckpointAlignmentSummary alignment) {
this.alignment = alignment;
}
public TaskCheckpointStatisticsWithSubtaskDetailsSummary checkpointDuration(CheckpointDurationSummary checkpointDuration) {
this.checkpointDuration = checkpointDuration;
return this;
}
/**
* Get checkpointDuration
* @return checkpointDuration
**/
@Schema(description = "")
public CheckpointDurationSummary getCheckpointDuration() {
return checkpointDuration;
}
public void setCheckpointDuration(CheckpointDurationSummary checkpointDuration) {
this.checkpointDuration = checkpointDuration;
}
public TaskCheckpointStatisticsWithSubtaskDetailsSummary checkpointedSize(StatsSummaryDto checkpointedSize) {
this.checkpointedSize = checkpointedSize;
return this;
}
/**
* Get checkpointedSize
* @return checkpointedSize
**/
@Schema(description = "")
public StatsSummaryDto getCheckpointedSize() {
return checkpointedSize;
}
public void setCheckpointedSize(StatsSummaryDto checkpointedSize) {
this.checkpointedSize = checkpointedSize;
}
public TaskCheckpointStatisticsWithSubtaskDetailsSummary endToEndDuration(StatsSummaryDto endToEndDuration) {
this.endToEndDuration = endToEndDuration;
return this;
}
/**
* Get endToEndDuration
* @return endToEndDuration
**/
@Schema(description = "")
public StatsSummaryDto getEndToEndDuration() {
return endToEndDuration;
}
public void setEndToEndDuration(StatsSummaryDto endToEndDuration) {
this.endToEndDuration = endToEndDuration;
}
public TaskCheckpointStatisticsWithSubtaskDetailsSummary startDelay(StatsSummaryDto startDelay) {
this.startDelay = startDelay;
return this;
}
/**
* Get startDelay
* @return startDelay
**/
@Schema(description = "")
public StatsSummaryDto getStartDelay() {
return startDelay;
}
public void setStartDelay(StatsSummaryDto startDelay) {
this.startDelay = startDelay;
}
public TaskCheckpointStatisticsWithSubtaskDetailsSummary stateSize(StatsSummaryDto stateSize) {
this.stateSize = stateSize;
return this;
}
/**
* Get stateSize
* @return stateSize
**/
@Schema(description = "")
public StatsSummaryDto getStateSize() {
return stateSize;
}
public void setStateSize(StatsSummaryDto stateSize) {
this.stateSize = stateSize;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskCheckpointStatisticsWithSubtaskDetailsSummary taskCheckpointStatisticsWithSubtaskDetailsSummary = (TaskCheckpointStatisticsWithSubtaskDetailsSummary) o;
return Objects.equals(this.alignment, taskCheckpointStatisticsWithSubtaskDetailsSummary.alignment) &&
Objects.equals(this.checkpointDuration, taskCheckpointStatisticsWithSubtaskDetailsSummary.checkpointDuration) &&
Objects.equals(this.checkpointedSize, taskCheckpointStatisticsWithSubtaskDetailsSummary.checkpointedSize) &&
Objects.equals(this.endToEndDuration, taskCheckpointStatisticsWithSubtaskDetailsSummary.endToEndDuration) &&
Objects.equals(this.startDelay, taskCheckpointStatisticsWithSubtaskDetailsSummary.startDelay) &&
Objects.equals(this.stateSize, taskCheckpointStatisticsWithSubtaskDetailsSummary.stateSize);
}
@Override
public int hashCode() {
return Objects.hash(alignment, checkpointDuration, checkpointedSize, endToEndDuration, startDelay, stateSize);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskCheckpointStatisticsWithSubtaskDetailsSummary {\n");
sb.append(" alignment: ").append(toIndentedString(alignment)).append("\n");
sb.append(" checkpointDuration: ").append(toIndentedString(checkpointDuration)).append("\n");
sb.append(" checkpointedSize: ").append(toIndentedString(checkpointedSize)).append("\n");
sb.append(" endToEndDuration: ").append(toIndentedString(endToEndDuration)).append("\n");
sb.append(" startDelay: ").append(toIndentedString(startDelay)).append("\n");
sb.append(" stateSize: ").append(toIndentedString(stateSize)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy