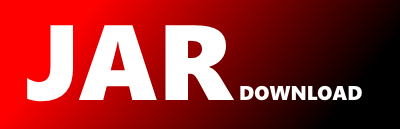
com.nextbreakpoint.flink.client.model.TaskManagerInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.HardwareDescription;
import com.nextbreakpoint.flink.client.model.ResourceProfileInfo;
import com.nextbreakpoint.flink.client.model.TaskExecutorMemoryConfiguration;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
/**
* TaskManagerInfo
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class TaskManagerInfo {
@SerializedName("blocked")
private Boolean blocked = null;
@SerializedName("dataPort")
private Integer dataPort = null;
@SerializedName("freeResource")
private ResourceProfileInfo freeResource = null;
@SerializedName("freeSlots")
private Integer freeSlots = null;
@SerializedName("hardware")
private HardwareDescription hardware = null;
@SerializedName("id")
private String id = null;
@SerializedName("jmxPort")
private Integer jmxPort = null;
@SerializedName("memoryConfiguration")
private TaskExecutorMemoryConfiguration memoryConfiguration = null;
@SerializedName("path")
private String path = null;
@SerializedName("slotsNumber")
private Integer slotsNumber = null;
@SerializedName("timeSinceLastHeartbeat")
private Long timeSinceLastHeartbeat = null;
@SerializedName("totalResource")
private ResourceProfileInfo totalResource = null;
public TaskManagerInfo blocked(Boolean blocked) {
this.blocked = blocked;
return this;
}
/**
* Get blocked
* @return blocked
**/
@Schema(description = "")
public Boolean isBlocked() {
return blocked;
}
public void setBlocked(Boolean blocked) {
this.blocked = blocked;
}
public TaskManagerInfo dataPort(Integer dataPort) {
this.dataPort = dataPort;
return this;
}
/**
* Get dataPort
* @return dataPort
**/
@Schema(description = "")
public Integer getDataPort() {
return dataPort;
}
public void setDataPort(Integer dataPort) {
this.dataPort = dataPort;
}
public TaskManagerInfo freeResource(ResourceProfileInfo freeResource) {
this.freeResource = freeResource;
return this;
}
/**
* Get freeResource
* @return freeResource
**/
@Schema(description = "")
public ResourceProfileInfo getFreeResource() {
return freeResource;
}
public void setFreeResource(ResourceProfileInfo freeResource) {
this.freeResource = freeResource;
}
public TaskManagerInfo freeSlots(Integer freeSlots) {
this.freeSlots = freeSlots;
return this;
}
/**
* Get freeSlots
* @return freeSlots
**/
@Schema(description = "")
public Integer getFreeSlots() {
return freeSlots;
}
public void setFreeSlots(Integer freeSlots) {
this.freeSlots = freeSlots;
}
public TaskManagerInfo hardware(HardwareDescription hardware) {
this.hardware = hardware;
return this;
}
/**
* Get hardware
* @return hardware
**/
@Schema(description = "")
public HardwareDescription getHardware() {
return hardware;
}
public void setHardware(HardwareDescription hardware) {
this.hardware = hardware;
}
public TaskManagerInfo id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@Schema(description = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public TaskManagerInfo jmxPort(Integer jmxPort) {
this.jmxPort = jmxPort;
return this;
}
/**
* Get jmxPort
* @return jmxPort
**/
@Schema(description = "")
public Integer getJmxPort() {
return jmxPort;
}
public void setJmxPort(Integer jmxPort) {
this.jmxPort = jmxPort;
}
public TaskManagerInfo memoryConfiguration(TaskExecutorMemoryConfiguration memoryConfiguration) {
this.memoryConfiguration = memoryConfiguration;
return this;
}
/**
* Get memoryConfiguration
* @return memoryConfiguration
**/
@Schema(description = "")
public TaskExecutorMemoryConfiguration getMemoryConfiguration() {
return memoryConfiguration;
}
public void setMemoryConfiguration(TaskExecutorMemoryConfiguration memoryConfiguration) {
this.memoryConfiguration = memoryConfiguration;
}
public TaskManagerInfo path(String path) {
this.path = path;
return this;
}
/**
* Get path
* @return path
**/
@Schema(description = "")
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public TaskManagerInfo slotsNumber(Integer slotsNumber) {
this.slotsNumber = slotsNumber;
return this;
}
/**
* Get slotsNumber
* @return slotsNumber
**/
@Schema(description = "")
public Integer getSlotsNumber() {
return slotsNumber;
}
public void setSlotsNumber(Integer slotsNumber) {
this.slotsNumber = slotsNumber;
}
public TaskManagerInfo timeSinceLastHeartbeat(Long timeSinceLastHeartbeat) {
this.timeSinceLastHeartbeat = timeSinceLastHeartbeat;
return this;
}
/**
* Get timeSinceLastHeartbeat
* @return timeSinceLastHeartbeat
**/
@Schema(description = "")
public Long getTimeSinceLastHeartbeat() {
return timeSinceLastHeartbeat;
}
public void setTimeSinceLastHeartbeat(Long timeSinceLastHeartbeat) {
this.timeSinceLastHeartbeat = timeSinceLastHeartbeat;
}
public TaskManagerInfo totalResource(ResourceProfileInfo totalResource) {
this.totalResource = totalResource;
return this;
}
/**
* Get totalResource
* @return totalResource
**/
@Schema(description = "")
public ResourceProfileInfo getTotalResource() {
return totalResource;
}
public void setTotalResource(ResourceProfileInfo totalResource) {
this.totalResource = totalResource;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskManagerInfo taskManagerInfo = (TaskManagerInfo) o;
return Objects.equals(this.blocked, taskManagerInfo.blocked) &&
Objects.equals(this.dataPort, taskManagerInfo.dataPort) &&
Objects.equals(this.freeResource, taskManagerInfo.freeResource) &&
Objects.equals(this.freeSlots, taskManagerInfo.freeSlots) &&
Objects.equals(this.hardware, taskManagerInfo.hardware) &&
Objects.equals(this.id, taskManagerInfo.id) &&
Objects.equals(this.jmxPort, taskManagerInfo.jmxPort) &&
Objects.equals(this.memoryConfiguration, taskManagerInfo.memoryConfiguration) &&
Objects.equals(this.path, taskManagerInfo.path) &&
Objects.equals(this.slotsNumber, taskManagerInfo.slotsNumber) &&
Objects.equals(this.timeSinceLastHeartbeat, taskManagerInfo.timeSinceLastHeartbeat) &&
Objects.equals(this.totalResource, taskManagerInfo.totalResource);
}
@Override
public int hashCode() {
return Objects.hash(blocked, dataPort, freeResource, freeSlots, hardware, id, jmxPort, memoryConfiguration, path, slotsNumber, timeSinceLastHeartbeat, totalResource);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskManagerInfo {\n");
sb.append(" blocked: ").append(toIndentedString(blocked)).append("\n");
sb.append(" dataPort: ").append(toIndentedString(dataPort)).append("\n");
sb.append(" freeResource: ").append(toIndentedString(freeResource)).append("\n");
sb.append(" freeSlots: ").append(toIndentedString(freeSlots)).append("\n");
sb.append(" hardware: ").append(toIndentedString(hardware)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" jmxPort: ").append(toIndentedString(jmxPort)).append("\n");
sb.append(" memoryConfiguration: ").append(toIndentedString(memoryConfiguration)).append("\n");
sb.append(" path: ").append(toIndentedString(path)).append("\n");
sb.append(" slotsNumber: ").append(toIndentedString(slotsNumber)).append("\n");
sb.append(" timeSinceLastHeartbeat: ").append(toIndentedString(timeSinceLastHeartbeat)).append("\n");
sb.append(" totalResource: ").append(toIndentedString(totalResource)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy