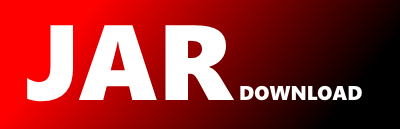
com.nextbreakpoint.flink.client.model.TaskManagerMetricsInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.client Show documentation
Show all versions of com.nextbreakpoint.flink.client Show documentation
Java client for managing Apache Flink via REST API
The newest version!
/*
* This file is part of Flink Client
* https://github.com/nextbreakpoint/flink-client
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flink.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.nextbreakpoint.flink.client.model.GarbageCollectorInfo;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* TaskManagerMetricsInfo
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2024-12-19T19:01:19.933513Z[Europe/London]")
public class TaskManagerMetricsInfo {
@SerializedName("directCount")
private Long directCount = null;
@SerializedName("directMax")
private Long directMax = null;
@SerializedName("directUsed")
private Long directUsed = null;
@SerializedName("garbageCollectors")
private List garbageCollectors = null;
@SerializedName("heapCommitted")
private Long heapCommitted = null;
@SerializedName("heapMax")
private Long heapMax = null;
@SerializedName("heapUsed")
private Long heapUsed = null;
@SerializedName("mappedCount")
private Long mappedCount = null;
@SerializedName("mappedMax")
private Long mappedMax = null;
@SerializedName("mappedUsed")
private Long mappedUsed = null;
@SerializedName("memorySegmentsAvailable")
private Long memorySegmentsAvailable = null;
@SerializedName("memorySegmentsTotal")
private Long memorySegmentsTotal = null;
@SerializedName("nettyShuffleMemoryAvailable")
private Long nettyShuffleMemoryAvailable = null;
@SerializedName("nettyShuffleMemorySegmentsAvailable")
private Long nettyShuffleMemorySegmentsAvailable = null;
@SerializedName("nettyShuffleMemorySegmentsTotal")
private Long nettyShuffleMemorySegmentsTotal = null;
@SerializedName("nettyShuffleMemorySegmentsUsed")
private Long nettyShuffleMemorySegmentsUsed = null;
@SerializedName("nettyShuffleMemoryTotal")
private Long nettyShuffleMemoryTotal = null;
@SerializedName("nettyShuffleMemoryUsed")
private Long nettyShuffleMemoryUsed = null;
@SerializedName("nonHeapCommitted")
private Long nonHeapCommitted = null;
@SerializedName("nonHeapMax")
private Long nonHeapMax = null;
@SerializedName("nonHeapUsed")
private Long nonHeapUsed = null;
public TaskManagerMetricsInfo directCount(Long directCount) {
this.directCount = directCount;
return this;
}
/**
* Get directCount
* @return directCount
**/
@Schema(description = "")
public Long getDirectCount() {
return directCount;
}
public void setDirectCount(Long directCount) {
this.directCount = directCount;
}
public TaskManagerMetricsInfo directMax(Long directMax) {
this.directMax = directMax;
return this;
}
/**
* Get directMax
* @return directMax
**/
@Schema(description = "")
public Long getDirectMax() {
return directMax;
}
public void setDirectMax(Long directMax) {
this.directMax = directMax;
}
public TaskManagerMetricsInfo directUsed(Long directUsed) {
this.directUsed = directUsed;
return this;
}
/**
* Get directUsed
* @return directUsed
**/
@Schema(description = "")
public Long getDirectUsed() {
return directUsed;
}
public void setDirectUsed(Long directUsed) {
this.directUsed = directUsed;
}
public TaskManagerMetricsInfo garbageCollectors(List garbageCollectors) {
this.garbageCollectors = garbageCollectors;
return this;
}
public TaskManagerMetricsInfo addGarbageCollectorsItem(GarbageCollectorInfo garbageCollectorsItem) {
if (this.garbageCollectors == null) {
this.garbageCollectors = new ArrayList<>();
}
this.garbageCollectors.add(garbageCollectorsItem);
return this;
}
/**
* Get garbageCollectors
* @return garbageCollectors
**/
@Schema(description = "")
public List getGarbageCollectors() {
return garbageCollectors;
}
public void setGarbageCollectors(List garbageCollectors) {
this.garbageCollectors = garbageCollectors;
}
public TaskManagerMetricsInfo heapCommitted(Long heapCommitted) {
this.heapCommitted = heapCommitted;
return this;
}
/**
* Get heapCommitted
* @return heapCommitted
**/
@Schema(description = "")
public Long getHeapCommitted() {
return heapCommitted;
}
public void setHeapCommitted(Long heapCommitted) {
this.heapCommitted = heapCommitted;
}
public TaskManagerMetricsInfo heapMax(Long heapMax) {
this.heapMax = heapMax;
return this;
}
/**
* Get heapMax
* @return heapMax
**/
@Schema(description = "")
public Long getHeapMax() {
return heapMax;
}
public void setHeapMax(Long heapMax) {
this.heapMax = heapMax;
}
public TaskManagerMetricsInfo heapUsed(Long heapUsed) {
this.heapUsed = heapUsed;
return this;
}
/**
* Get heapUsed
* @return heapUsed
**/
@Schema(description = "")
public Long getHeapUsed() {
return heapUsed;
}
public void setHeapUsed(Long heapUsed) {
this.heapUsed = heapUsed;
}
public TaskManagerMetricsInfo mappedCount(Long mappedCount) {
this.mappedCount = mappedCount;
return this;
}
/**
* Get mappedCount
* @return mappedCount
**/
@Schema(description = "")
public Long getMappedCount() {
return mappedCount;
}
public void setMappedCount(Long mappedCount) {
this.mappedCount = mappedCount;
}
public TaskManagerMetricsInfo mappedMax(Long mappedMax) {
this.mappedMax = mappedMax;
return this;
}
/**
* Get mappedMax
* @return mappedMax
**/
@Schema(description = "")
public Long getMappedMax() {
return mappedMax;
}
public void setMappedMax(Long mappedMax) {
this.mappedMax = mappedMax;
}
public TaskManagerMetricsInfo mappedUsed(Long mappedUsed) {
this.mappedUsed = mappedUsed;
return this;
}
/**
* Get mappedUsed
* @return mappedUsed
**/
@Schema(description = "")
public Long getMappedUsed() {
return mappedUsed;
}
public void setMappedUsed(Long mappedUsed) {
this.mappedUsed = mappedUsed;
}
public TaskManagerMetricsInfo memorySegmentsAvailable(Long memorySegmentsAvailable) {
this.memorySegmentsAvailable = memorySegmentsAvailable;
return this;
}
/**
* Get memorySegmentsAvailable
* @return memorySegmentsAvailable
**/
@Schema(description = "")
public Long getMemorySegmentsAvailable() {
return memorySegmentsAvailable;
}
public void setMemorySegmentsAvailable(Long memorySegmentsAvailable) {
this.memorySegmentsAvailable = memorySegmentsAvailable;
}
public TaskManagerMetricsInfo memorySegmentsTotal(Long memorySegmentsTotal) {
this.memorySegmentsTotal = memorySegmentsTotal;
return this;
}
/**
* Get memorySegmentsTotal
* @return memorySegmentsTotal
**/
@Schema(description = "")
public Long getMemorySegmentsTotal() {
return memorySegmentsTotal;
}
public void setMemorySegmentsTotal(Long memorySegmentsTotal) {
this.memorySegmentsTotal = memorySegmentsTotal;
}
public TaskManagerMetricsInfo nettyShuffleMemoryAvailable(Long nettyShuffleMemoryAvailable) {
this.nettyShuffleMemoryAvailable = nettyShuffleMemoryAvailable;
return this;
}
/**
* Get nettyShuffleMemoryAvailable
* @return nettyShuffleMemoryAvailable
**/
@Schema(description = "")
public Long getNettyShuffleMemoryAvailable() {
return nettyShuffleMemoryAvailable;
}
public void setNettyShuffleMemoryAvailable(Long nettyShuffleMemoryAvailable) {
this.nettyShuffleMemoryAvailable = nettyShuffleMemoryAvailable;
}
public TaskManagerMetricsInfo nettyShuffleMemorySegmentsAvailable(Long nettyShuffleMemorySegmentsAvailable) {
this.nettyShuffleMemorySegmentsAvailable = nettyShuffleMemorySegmentsAvailable;
return this;
}
/**
* Get nettyShuffleMemorySegmentsAvailable
* @return nettyShuffleMemorySegmentsAvailable
**/
@Schema(description = "")
public Long getNettyShuffleMemorySegmentsAvailable() {
return nettyShuffleMemorySegmentsAvailable;
}
public void setNettyShuffleMemorySegmentsAvailable(Long nettyShuffleMemorySegmentsAvailable) {
this.nettyShuffleMemorySegmentsAvailable = nettyShuffleMemorySegmentsAvailable;
}
public TaskManagerMetricsInfo nettyShuffleMemorySegmentsTotal(Long nettyShuffleMemorySegmentsTotal) {
this.nettyShuffleMemorySegmentsTotal = nettyShuffleMemorySegmentsTotal;
return this;
}
/**
* Get nettyShuffleMemorySegmentsTotal
* @return nettyShuffleMemorySegmentsTotal
**/
@Schema(description = "")
public Long getNettyShuffleMemorySegmentsTotal() {
return nettyShuffleMemorySegmentsTotal;
}
public void setNettyShuffleMemorySegmentsTotal(Long nettyShuffleMemorySegmentsTotal) {
this.nettyShuffleMemorySegmentsTotal = nettyShuffleMemorySegmentsTotal;
}
public TaskManagerMetricsInfo nettyShuffleMemorySegmentsUsed(Long nettyShuffleMemorySegmentsUsed) {
this.nettyShuffleMemorySegmentsUsed = nettyShuffleMemorySegmentsUsed;
return this;
}
/**
* Get nettyShuffleMemorySegmentsUsed
* @return nettyShuffleMemorySegmentsUsed
**/
@Schema(description = "")
public Long getNettyShuffleMemorySegmentsUsed() {
return nettyShuffleMemorySegmentsUsed;
}
public void setNettyShuffleMemorySegmentsUsed(Long nettyShuffleMemorySegmentsUsed) {
this.nettyShuffleMemorySegmentsUsed = nettyShuffleMemorySegmentsUsed;
}
public TaskManagerMetricsInfo nettyShuffleMemoryTotal(Long nettyShuffleMemoryTotal) {
this.nettyShuffleMemoryTotal = nettyShuffleMemoryTotal;
return this;
}
/**
* Get nettyShuffleMemoryTotal
* @return nettyShuffleMemoryTotal
**/
@Schema(description = "")
public Long getNettyShuffleMemoryTotal() {
return nettyShuffleMemoryTotal;
}
public void setNettyShuffleMemoryTotal(Long nettyShuffleMemoryTotal) {
this.nettyShuffleMemoryTotal = nettyShuffleMemoryTotal;
}
public TaskManagerMetricsInfo nettyShuffleMemoryUsed(Long nettyShuffleMemoryUsed) {
this.nettyShuffleMemoryUsed = nettyShuffleMemoryUsed;
return this;
}
/**
* Get nettyShuffleMemoryUsed
* @return nettyShuffleMemoryUsed
**/
@Schema(description = "")
public Long getNettyShuffleMemoryUsed() {
return nettyShuffleMemoryUsed;
}
public void setNettyShuffleMemoryUsed(Long nettyShuffleMemoryUsed) {
this.nettyShuffleMemoryUsed = nettyShuffleMemoryUsed;
}
public TaskManagerMetricsInfo nonHeapCommitted(Long nonHeapCommitted) {
this.nonHeapCommitted = nonHeapCommitted;
return this;
}
/**
* Get nonHeapCommitted
* @return nonHeapCommitted
**/
@Schema(description = "")
public Long getNonHeapCommitted() {
return nonHeapCommitted;
}
public void setNonHeapCommitted(Long nonHeapCommitted) {
this.nonHeapCommitted = nonHeapCommitted;
}
public TaskManagerMetricsInfo nonHeapMax(Long nonHeapMax) {
this.nonHeapMax = nonHeapMax;
return this;
}
/**
* Get nonHeapMax
* @return nonHeapMax
**/
@Schema(description = "")
public Long getNonHeapMax() {
return nonHeapMax;
}
public void setNonHeapMax(Long nonHeapMax) {
this.nonHeapMax = nonHeapMax;
}
public TaskManagerMetricsInfo nonHeapUsed(Long nonHeapUsed) {
this.nonHeapUsed = nonHeapUsed;
return this;
}
/**
* Get nonHeapUsed
* @return nonHeapUsed
**/
@Schema(description = "")
public Long getNonHeapUsed() {
return nonHeapUsed;
}
public void setNonHeapUsed(Long nonHeapUsed) {
this.nonHeapUsed = nonHeapUsed;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskManagerMetricsInfo taskManagerMetricsInfo = (TaskManagerMetricsInfo) o;
return Objects.equals(this.directCount, taskManagerMetricsInfo.directCount) &&
Objects.equals(this.directMax, taskManagerMetricsInfo.directMax) &&
Objects.equals(this.directUsed, taskManagerMetricsInfo.directUsed) &&
Objects.equals(this.garbageCollectors, taskManagerMetricsInfo.garbageCollectors) &&
Objects.equals(this.heapCommitted, taskManagerMetricsInfo.heapCommitted) &&
Objects.equals(this.heapMax, taskManagerMetricsInfo.heapMax) &&
Objects.equals(this.heapUsed, taskManagerMetricsInfo.heapUsed) &&
Objects.equals(this.mappedCount, taskManagerMetricsInfo.mappedCount) &&
Objects.equals(this.mappedMax, taskManagerMetricsInfo.mappedMax) &&
Objects.equals(this.mappedUsed, taskManagerMetricsInfo.mappedUsed) &&
Objects.equals(this.memorySegmentsAvailable, taskManagerMetricsInfo.memorySegmentsAvailable) &&
Objects.equals(this.memorySegmentsTotal, taskManagerMetricsInfo.memorySegmentsTotal) &&
Objects.equals(this.nettyShuffleMemoryAvailable, taskManagerMetricsInfo.nettyShuffleMemoryAvailable) &&
Objects.equals(this.nettyShuffleMemorySegmentsAvailable, taskManagerMetricsInfo.nettyShuffleMemorySegmentsAvailable) &&
Objects.equals(this.nettyShuffleMemorySegmentsTotal, taskManagerMetricsInfo.nettyShuffleMemorySegmentsTotal) &&
Objects.equals(this.nettyShuffleMemorySegmentsUsed, taskManagerMetricsInfo.nettyShuffleMemorySegmentsUsed) &&
Objects.equals(this.nettyShuffleMemoryTotal, taskManagerMetricsInfo.nettyShuffleMemoryTotal) &&
Objects.equals(this.nettyShuffleMemoryUsed, taskManagerMetricsInfo.nettyShuffleMemoryUsed) &&
Objects.equals(this.nonHeapCommitted, taskManagerMetricsInfo.nonHeapCommitted) &&
Objects.equals(this.nonHeapMax, taskManagerMetricsInfo.nonHeapMax) &&
Objects.equals(this.nonHeapUsed, taskManagerMetricsInfo.nonHeapUsed);
}
@Override
public int hashCode() {
return Objects.hash(directCount, directMax, directUsed, garbageCollectors, heapCommitted, heapMax, heapUsed, mappedCount, mappedMax, mappedUsed, memorySegmentsAvailable, memorySegmentsTotal, nettyShuffleMemoryAvailable, nettyShuffleMemorySegmentsAvailable, nettyShuffleMemorySegmentsTotal, nettyShuffleMemorySegmentsUsed, nettyShuffleMemoryTotal, nettyShuffleMemoryUsed, nonHeapCommitted, nonHeapMax, nonHeapUsed);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskManagerMetricsInfo {\n");
sb.append(" directCount: ").append(toIndentedString(directCount)).append("\n");
sb.append(" directMax: ").append(toIndentedString(directMax)).append("\n");
sb.append(" directUsed: ").append(toIndentedString(directUsed)).append("\n");
sb.append(" garbageCollectors: ").append(toIndentedString(garbageCollectors)).append("\n");
sb.append(" heapCommitted: ").append(toIndentedString(heapCommitted)).append("\n");
sb.append(" heapMax: ").append(toIndentedString(heapMax)).append("\n");
sb.append(" heapUsed: ").append(toIndentedString(heapUsed)).append("\n");
sb.append(" mappedCount: ").append(toIndentedString(mappedCount)).append("\n");
sb.append(" mappedMax: ").append(toIndentedString(mappedMax)).append("\n");
sb.append(" mappedUsed: ").append(toIndentedString(mappedUsed)).append("\n");
sb.append(" memorySegmentsAvailable: ").append(toIndentedString(memorySegmentsAvailable)).append("\n");
sb.append(" memorySegmentsTotal: ").append(toIndentedString(memorySegmentsTotal)).append("\n");
sb.append(" nettyShuffleMemoryAvailable: ").append(toIndentedString(nettyShuffleMemoryAvailable)).append("\n");
sb.append(" nettyShuffleMemorySegmentsAvailable: ").append(toIndentedString(nettyShuffleMemorySegmentsAvailable)).append("\n");
sb.append(" nettyShuffleMemorySegmentsTotal: ").append(toIndentedString(nettyShuffleMemorySegmentsTotal)).append("\n");
sb.append(" nettyShuffleMemorySegmentsUsed: ").append(toIndentedString(nettyShuffleMemorySegmentsUsed)).append("\n");
sb.append(" nettyShuffleMemoryTotal: ").append(toIndentedString(nettyShuffleMemoryTotal)).append("\n");
sb.append(" nettyShuffleMemoryUsed: ").append(toIndentedString(nettyShuffleMemoryUsed)).append("\n");
sb.append(" nonHeapCommitted: ").append(toIndentedString(nonHeapCommitted)).append("\n");
sb.append(" nonHeapMax: ").append(toIndentedString(nonHeapMax)).append("\n");
sb.append(" nonHeapUsed: ").append(toIndentedString(nonHeapUsed)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy