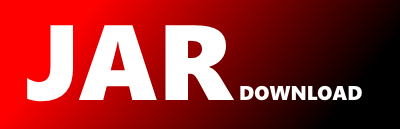
com.nextbreakpoint.flink.dummies.Parameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.dummies Show documentation
Show all versions of com.nextbreakpoint.flink.dummies Show documentation
Collection of dummy jobs for Apache Flink
/*
* This file is part of Flink Dummies
* https://github.com/nextbreakpoint/flink-dummies
*/
package com.nextbreakpoint.flink.dummies;
import lombok.AccessLevel;
import lombok.NoArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.apache.flink.api.java.utils.ParameterTool;
import javax.annotation.Nonnull;
@Slf4j
@NoArgsConstructor(access = AccessLevel.PRIVATE)
public class Parameters {
public static final String PARALLELISM = "PARALLELISM";
public static final String CONFIG_FILE = "CONFIG_FILE";
public static final String DEBUG_MODE = "DEBUG_MODE";
public static @Nonnull String getRequiredParam(ParameterTool parameters, String paramName) {
String param = parameters.get(paramName, System.getProperty(paramName, System.getenv(paramName)));
if (param == null || param.trim().isEmpty()) {
throw new RuntimeException(paramName + " parameter can not be empty");
} else {
return param;
}
}
public static @Nonnull String getOptionalParam(ParameterTool parameters, String paramName, String defaultValue) {
final String s = parameters.get(paramName, System.getProperty(paramName, System.getenv(paramName)));
if (s == null) {
log.info("Parameter '{}' is null (not set), using default '{}'", paramName, defaultValue);
}
return s != null ? s : defaultValue;
}
public static String getOptionalParam(ParameterTool parameters, String paramName) {
final String s = parameters.get(paramName, System.getProperty(paramName, System.getenv(paramName)));
if (s == null) {
log.info("Parameter '{}' is null (not set), using default or whatever...", paramName);
}
return s;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy