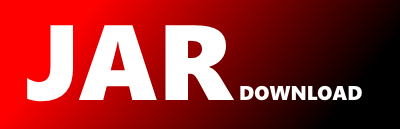
com.nextbreakpoint.flink.dummies.Utilities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flink.dummies Show documentation
Show all versions of com.nextbreakpoint.flink.dummies Show documentation
Collection of dummy jobs for Apache Flink
/*
* This file is part of Flink Dummies
* https://github.com/nextbreakpoint/flink-dummies
*/
package com.nextbreakpoint.flink.dummies;
import lombok.AccessLevel;
import lombok.NoArgsConstructor;
import org.apache.flink.core.fs.FileSystem;
import org.apache.flink.core.fs.Path;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.time.Clock;
import java.time.Instant;
import java.time.format.DateTimeFormatter;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
@NoArgsConstructor(access = AccessLevel.PRIVATE)
public class Utilities {
private static final DateTimeFormatter TIMESTAMP_FORMAT = DateTimeFormatter
.ofPattern("yyyy'-'MM'-'dd'T'HH:mm:ss.SSS'Z'").withZone(Clock.systemUTC().getZone());
private static final Pattern PROPERTY_PATTERN = Pattern.compile("(.*):(.*)");
private static final Pattern VARIABLE_PATTERN = Pattern.compile("\\$\\{([a-zA-Z]+[a-zA-Z0-9_]*)}");
public static @Nonnull Map getProperties(FileSystem fileSystem, Path path) throws IOException {
try (InputStream stream = fileSystem.open(path)) {
return getProperties(new String(stream.readAllBytes(), StandardCharsets.UTF_8), "\\n");
}
}
public static @Nonnull Map getProperties(String string, String separator) {
final String[] strings = resolveVariables(string).strip().split(separator);
return Arrays.stream(strings)
.filter(line -> !line.isBlank())
.filter(line -> !line.startsWith("#"))
.map(PROPERTY_PATTERN::matcher)
.filter(Matcher::matches)
.collect(Collectors.toMap(matcher -> matcher.group(1).trim(), matcher -> matcher.group(2).trim()));
}
public static @Nonnull String formatInstant(Instant instant) {
return TIMESTAMP_FORMAT.format(instant);
}
public static @Nonnull List getSensors(int size, List locations) {
return IntStream.range(0, size)
.mapToObj(i -> getSensor(locations.get(i % locations.size())))
.collect(Collectors.toList());
}
public static @Nonnull List getLocations(int size) {
return IntStream.range(0, size)
.mapToObj(i -> UUID.randomUUID().toString())
.collect(Collectors.toList());
}
public static @Nonnull TemperatureSensor getSensor(String locationId) {
return TemperatureSensor.builder()
.withSensorId(UUID.randomUUID().toString())
.withLocationId(locationId)
.withValue(someTemperature(20, 5))
.build();
}
public static int someTemperature(int value, int range) {
return (int) Math.rint(value + Math.random() * range);
}
public static int sampleTemperature(TemperatureSensor sensor, Instant timestamp) {
return (int) Math.rint(nextTemperatureValue(sensor.getValue().doubleValue(), timestamp.toEpochMilli()));
}
private static double nextTemperatureValue(double amplitude, long timestamp) {
return Math.sin(2 * Math.PI * (timestamp / 1000.0)) * amplitude / 10 + (Math.random() * amplitude / 20.0) + amplitude;
}
private static String resolveVariables(String input) {
if (input == null) {
return null;
}
final Matcher matcher = VARIABLE_PATTERN.matcher(input);
final StringBuilder sb = new StringBuilder();
while (matcher.find()) {
matcher.appendReplacement(sb, evaluate(matcher.group(1)));
}
matcher.appendTail(sb);
return sb.toString();
}
private static String evaluate(String expression) {
String value = System.getenv(expression);
if (value == null) {
throw new RuntimeException("Can't resolve expression: ${" + expression + "}");
}
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy