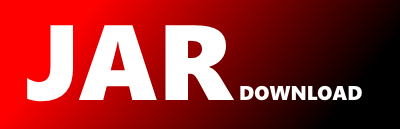
com.nextbreakpoint.flinkclient.api.DefaultApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.nextbreakpoint.flinkclient.core Show documentation
Show all versions of com.nextbreakpoint.flinkclient.core Show documentation
Cope API for managing Apache Flink
The newest version!
/*
* This file is part of FlinkClient
* https://github.com/nextbreakpoint/flinkclient
*
* OpenAPI spec version: v1/1.20-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.nextbreakpoint.flinkclient.api;
import com.nextbreakpoint.flinkclient.api.ApiCallback;
import com.nextbreakpoint.flinkclient.api.ApiClient;
import com.nextbreakpoint.flinkclient.api.ApiException;
import com.nextbreakpoint.flinkclient.api.ApiResponse;
import com.nextbreakpoint.flinkclient.api.Configuration;
import com.nextbreakpoint.flinkclient.api.Pair;
import com.nextbreakpoint.flinkclient.api.ProgressRequestBody;
import com.nextbreakpoint.flinkclient.api.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.nextbreakpoint.flinkclient.model.AggregatedMetricsResponseBody;
import com.nextbreakpoint.flinkclient.model.AggregationMode;
import com.nextbreakpoint.flinkclient.model.AsynchronousOperationResult;
import com.nextbreakpoint.flinkclient.model.CheckpointConfigInfo;
import com.nextbreakpoint.flinkclient.model.CheckpointStatistics;
import com.nextbreakpoint.flinkclient.model.CheckpointTriggerRequestBody;
import com.nextbreakpoint.flinkclient.model.CheckpointingStatistics;
import com.nextbreakpoint.flinkclient.model.ClusterDataSetListResponseBody;
import com.nextbreakpoint.flinkclient.model.ClusterOverviewWithVersion;
import com.nextbreakpoint.flinkclient.model.ConfigurationInfoEntry;
import com.nextbreakpoint.flinkclient.model.DashboardConfiguration;
import com.nextbreakpoint.flinkclient.model.EnvironmentInfo;
import com.nextbreakpoint.flinkclient.model.FailureLabel;
import java.io.File;
import com.nextbreakpoint.flinkclient.model.JarListInfo;
import com.nextbreakpoint.flinkclient.model.JarPlanRequestBody;
import com.nextbreakpoint.flinkclient.model.JarRunRequestBody;
import com.nextbreakpoint.flinkclient.model.JarRunResponseBody;
import com.nextbreakpoint.flinkclient.model.JarUploadResponseBody;
import com.nextbreakpoint.flinkclient.model.JobAccumulatorsInfo;
import com.nextbreakpoint.flinkclient.model.JobClientHeartbeatRequestBody;
import com.nextbreakpoint.flinkclient.model.JobConfigInfo;
import com.nextbreakpoint.flinkclient.model.JobDetailsInfo;
import com.nextbreakpoint.flinkclient.model.JobExceptionsInfoWithHistory;
import com.nextbreakpoint.flinkclient.model.JobExecutionResultResponseBody;
import com.nextbreakpoint.flinkclient.model.JobIdsWithStatusOverview;
import com.nextbreakpoint.flinkclient.model.JobPlanInfo;
import com.nextbreakpoint.flinkclient.model.JobResourceRequirementsBody;
import com.nextbreakpoint.flinkclient.model.JobStatusInfo;
import com.nextbreakpoint.flinkclient.model.JobSubmitRequestBody;
import com.nextbreakpoint.flinkclient.model.JobSubmitResponseBody;
import com.nextbreakpoint.flinkclient.model.JobVertexAccumulatorsInfo;
import com.nextbreakpoint.flinkclient.model.JobVertexBackPressureInfo;
import com.nextbreakpoint.flinkclient.model.JobVertexDetailsInfo;
import com.nextbreakpoint.flinkclient.model.JobVertexResourceRequirements;
import com.nextbreakpoint.flinkclient.model.JobVertexTaskManagersInfo;
import com.nextbreakpoint.flinkclient.model.LogListInfo;
import com.nextbreakpoint.flinkclient.model.LogUrlResponse;
import com.nextbreakpoint.flinkclient.model.MetricCollectionResponseBody;
import com.nextbreakpoint.flinkclient.model.MultipleJobsDetails;
import com.nextbreakpoint.flinkclient.model.SavepointDisposalRequest;
import com.nextbreakpoint.flinkclient.model.SavepointTriggerRequestBody;
import com.nextbreakpoint.flinkclient.model.StopWithSavepointRequestBody;
import com.nextbreakpoint.flinkclient.model.SubtaskExecutionAttemptAccumulatorsInfo;
import com.nextbreakpoint.flinkclient.model.SubtaskExecutionAttemptDetailsInfo;
import com.nextbreakpoint.flinkclient.model.SubtasksAllAccumulatorsInfo;
import com.nextbreakpoint.flinkclient.model.SubtasksTimesInfo;
import com.nextbreakpoint.flinkclient.model.TaskCheckpointStatisticsWithSubtaskDetails;
import com.nextbreakpoint.flinkclient.model.TaskManagerDetailsInfo;
import com.nextbreakpoint.flinkclient.model.TaskManagersInfo;
import com.nextbreakpoint.flinkclient.model.TerminationMode;
import com.nextbreakpoint.flinkclient.model.ThreadDumpInfo;
import com.nextbreakpoint.flinkclient.model.ThreadStates;
import com.nextbreakpoint.flinkclient.model.TriggerResponse;
import com.nextbreakpoint.flinkclient.model.VertexFlameGraph;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class DefaultApi {
private ApiClient apiClient;
public DefaultApi() {
this(Configuration.getDefaultApiClient());
}
public DefaultApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for cancelJob
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param mode String value that specifies the termination mode. The only supported value is: \"cancel\". (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call cancelJobCall(String jobid, TerminationMode mode, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (mode != null)
localVarQueryParams.addAll(apiClient.parameterToPair("mode", mode));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call cancelJobValidateBeforeCall(String jobid, TerminationMode mode, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling cancelJob(Async)");
}
okhttp3.Call call = cancelJobCall(jobid, mode, progressListener, progressRequestListener);
return call;
}
/**
*
* Terminates a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param mode String value that specifies the termination mode. The only supported value is: \"cancel\". (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void cancelJob(String jobid, TerminationMode mode) throws ApiException {
cancelJobWithHttpInfo(jobid, mode);
}
/**
*
* Terminates a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param mode String value that specifies the termination mode. The only supported value is: \"cancel\". (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse cancelJobWithHttpInfo(String jobid, TerminationMode mode) throws ApiException {
okhttp3.Call call = cancelJobValidateBeforeCall(jobid, mode, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
* Terminates a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param mode String value that specifies the termination mode. The only supported value is: \"cancel\". (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call cancelJobAsync(String jobid, TerminationMode mode, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = cancelJobValidateBeforeCall(jobid, mode, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteClusterDataSet
* @param datasetid 32-character hexadecimal string value that identifies a cluster data set. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call deleteClusterDataSetCall(String datasetid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/datasets/{datasetid}"
.replaceAll("\\{" + "datasetid" + "\\}", apiClient.escapeString(datasetid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call deleteClusterDataSetValidateBeforeCall(String datasetid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'datasetid' is set
if (datasetid == null) {
throw new ApiException("Missing the required parameter 'datasetid' when calling deleteClusterDataSet(Async)");
}
okhttp3.Call call = deleteClusterDataSetCall(datasetid, progressListener, progressRequestListener);
return call;
}
/**
*
* Triggers the deletion of a cluster data set. This async operation would return a 'triggerid' for further query identifier.
* @param datasetid 32-character hexadecimal string value that identifies a cluster data set. (required)
* @return TriggerResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TriggerResponse deleteClusterDataSet(String datasetid) throws ApiException {
ApiResponse resp = deleteClusterDataSetWithHttpInfo(datasetid);
return resp.getData();
}
/**
*
* Triggers the deletion of a cluster data set. This async operation would return a 'triggerid' for further query identifier.
* @param datasetid 32-character hexadecimal string value that identifies a cluster data set. (required)
* @return ApiResponse<TriggerResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteClusterDataSetWithHttpInfo(String datasetid) throws ApiException {
okhttp3.Call call = deleteClusterDataSetValidateBeforeCall(datasetid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Triggers the deletion of a cluster data set. This async operation would return a 'triggerid' for further query identifier.
* @param datasetid 32-character hexadecimal string value that identifies a cluster data set. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call deleteClusterDataSetAsync(String datasetid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = deleteClusterDataSetValidateBeforeCall(datasetid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteJar
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call deleteJarCall(String jarid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jars/{jarid}"
.replaceAll("\\{" + "jarid" + "\\}", apiClient.escapeString(jarid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call deleteJarValidateBeforeCall(String jarid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jarid' is set
if (jarid == null) {
throw new ApiException("Missing the required parameter 'jarid' when calling deleteJar(Async)");
}
okhttp3.Call call = deleteJarCall(jarid, progressListener, progressRequestListener);
return call;
}
/**
*
* Deletes a jar previously uploaded via '/jars/upload'.
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteJar(String jarid) throws ApiException {
deleteJarWithHttpInfo(jarid);
}
/**
*
* Deletes a jar previously uploaded via '/jars/upload'.
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteJarWithHttpInfo(String jarid) throws ApiException {
okhttp3.Call call = deleteJarValidateBeforeCall(jarid, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
* Deletes a jar previously uploaded via '/jars/upload'.
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call deleteJarAsync(String jarid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = deleteJarValidateBeforeCall(jarid, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for generatePlanFromJar
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @param body (optional)
* @param programArgs Deprecated, please use 'programArg' instead. String value that specifies the arguments for the program or plan (optional)
* @param programArg Comma-separated list of program arguments. (optional)
* @param entryClass String value that specifies the fully qualified name of the entry point class. Overrides the class defined in the jar file manifest. (optional)
* @param parallelism Positive integer value that specifies the desired parallelism for the job. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call generatePlanFromJarCall(String jarid, JarPlanRequestBody body, String programArgs, String programArg, String entryClass, Integer parallelism, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/jars/{jarid}/plan"
.replaceAll("\\{" + "jarid" + "\\}", apiClient.escapeString(jarid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (programArgs != null)
localVarQueryParams.addAll(apiClient.parameterToPair("program-args", programArgs));
if (programArg != null)
localVarQueryParams.addAll(apiClient.parameterToPair("programArg", programArg));
if (entryClass != null)
localVarQueryParams.addAll(apiClient.parameterToPair("entry-class", entryClass));
if (parallelism != null)
localVarQueryParams.addAll(apiClient.parameterToPair("parallelism", parallelism));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call generatePlanFromJarValidateBeforeCall(String jarid, JarPlanRequestBody body, String programArgs, String programArg, String entryClass, Integer parallelism, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jarid' is set
if (jarid == null) {
throw new ApiException("Missing the required parameter 'jarid' when calling generatePlanFromJar(Async)");
}
okhttp3.Call call = generatePlanFromJarCall(jarid, body, programArgs, programArg, entryClass, parallelism, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the dataflow plan of a job contained in a jar previously uploaded via '/jars/upload'. Program arguments can be passed both via the JSON request (recommended) or query parameters.
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @param body (optional)
* @param programArgs Deprecated, please use 'programArg' instead. String value that specifies the arguments for the program or plan (optional)
* @param programArg Comma-separated list of program arguments. (optional)
* @param entryClass String value that specifies the fully qualified name of the entry point class. Overrides the class defined in the jar file manifest. (optional)
* @param parallelism Positive integer value that specifies the desired parallelism for the job. (optional)
* @return JobPlanInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public JobPlanInfo generatePlanFromJar(String jarid, JarPlanRequestBody body, String programArgs, String programArg, String entryClass, Integer parallelism) throws ApiException {
ApiResponse resp = generatePlanFromJarWithHttpInfo(jarid, body, programArgs, programArg, entryClass, parallelism);
return resp.getData();
}
/**
*
* Returns the dataflow plan of a job contained in a jar previously uploaded via '/jars/upload'. Program arguments can be passed both via the JSON request (recommended) or query parameters.
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @param body (optional)
* @param programArgs Deprecated, please use 'programArg' instead. String value that specifies the arguments for the program or plan (optional)
* @param programArg Comma-separated list of program arguments. (optional)
* @param entryClass String value that specifies the fully qualified name of the entry point class. Overrides the class defined in the jar file manifest. (optional)
* @param parallelism Positive integer value that specifies the desired parallelism for the job. (optional)
* @return ApiResponse<JobPlanInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse generatePlanFromJarWithHttpInfo(String jarid, JarPlanRequestBody body, String programArgs, String programArg, String entryClass, Integer parallelism) throws ApiException {
okhttp3.Call call = generatePlanFromJarValidateBeforeCall(jarid, body, programArgs, programArg, entryClass, parallelism, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the dataflow plan of a job contained in a jar previously uploaded via '/jars/upload'. Program arguments can be passed both via the JSON request (recommended) or query parameters.
* @param jarid String value that identifies a jar. When uploading the jar a path is returned, where the filename is the ID. This value is equivalent to the `id` field in the list of uploaded jars (/jars). (required)
* @param body (optional)
* @param programArgs Deprecated, please use 'programArg' instead. String value that specifies the arguments for the program or plan (optional)
* @param programArg Comma-separated list of program arguments. (optional)
* @param entryClass String value that specifies the fully qualified name of the entry point class. Overrides the class defined in the jar file manifest. (optional)
* @param parallelism Positive integer value that specifies the desired parallelism for the job. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call generatePlanFromJarAsync(String jarid, JarPlanRequestBody body, String programArgs, String programArg, String entryClass, Integer parallelism, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = generatePlanFromJarValidateBeforeCall(jarid, body, programArgs, programArg, entryClass, parallelism, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getAggregatedJobMetrics
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param jobs Comma-separated list of 32-character hexadecimal strings to select specific jobs. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getAggregatedJobMetricsCall(String get, AggregationMode agg, String jobs, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/metrics";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (get != null)
localVarQueryParams.addAll(apiClient.parameterToPair("get", get));
if (agg != null)
localVarQueryParams.addAll(apiClient.parameterToPair("agg", agg));
if (jobs != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jobs", jobs));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getAggregatedJobMetricsValidateBeforeCall(String get, AggregationMode agg, String jobs, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getAggregatedJobMetricsCall(get, agg, jobs, progressListener, progressRequestListener);
return call;
}
/**
*
* Provides access to aggregated job metrics.
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param jobs Comma-separated list of 32-character hexadecimal strings to select specific jobs. (optional)
* @return AggregatedMetricsResponseBody
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AggregatedMetricsResponseBody getAggregatedJobMetrics(String get, AggregationMode agg, String jobs) throws ApiException {
ApiResponse resp = getAggregatedJobMetricsWithHttpInfo(get, agg, jobs);
return resp.getData();
}
/**
*
* Provides access to aggregated job metrics.
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param jobs Comma-separated list of 32-character hexadecimal strings to select specific jobs. (optional)
* @return ApiResponse<AggregatedMetricsResponseBody>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAggregatedJobMetricsWithHttpInfo(String get, AggregationMode agg, String jobs) throws ApiException {
okhttp3.Call call = getAggregatedJobMetricsValidateBeforeCall(get, agg, jobs, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Provides access to aggregated job metrics.
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param jobs Comma-separated list of 32-character hexadecimal strings to select specific jobs. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getAggregatedJobMetricsAsync(String get, AggregationMode agg, String jobs, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getAggregatedJobMetricsValidateBeforeCall(get, agg, jobs, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getAggregatedSubtaskMetrics
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param vertexid 32-character hexadecimal string value that identifies a job vertex. (required)
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param subtasks Comma-separated list of integer ranges (e.g. \"1,3,5-9\") to select specific subtasks. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getAggregatedSubtaskMetricsCall(String jobid, String vertexid, String get, AggregationMode agg, String subtasks, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}/vertices/{vertexid}/subtasks/metrics"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()))
.replaceAll("\\{" + "vertexid" + "\\}", apiClient.escapeString(vertexid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (get != null)
localVarQueryParams.addAll(apiClient.parameterToPair("get", get));
if (agg != null)
localVarQueryParams.addAll(apiClient.parameterToPair("agg", agg));
if (subtasks != null)
localVarQueryParams.addAll(apiClient.parameterToPair("subtasks", subtasks));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getAggregatedSubtaskMetricsValidateBeforeCall(String jobid, String vertexid, String get, AggregationMode agg, String subtasks, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getAggregatedSubtaskMetrics(Async)");
}
// verify the required parameter 'vertexid' is set
if (vertexid == null) {
throw new ApiException("Missing the required parameter 'vertexid' when calling getAggregatedSubtaskMetrics(Async)");
}
okhttp3.Call call = getAggregatedSubtaskMetricsCall(jobid, vertexid, get, agg, subtasks, progressListener, progressRequestListener);
return call;
}
/**
*
* Provides access to aggregated subtask metrics.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param vertexid 32-character hexadecimal string value that identifies a job vertex. (required)
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param subtasks Comma-separated list of integer ranges (e.g. \"1,3,5-9\") to select specific subtasks. (optional)
* @return AggregatedMetricsResponseBody
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AggregatedMetricsResponseBody getAggregatedSubtaskMetrics(String jobid, String vertexid, String get, AggregationMode agg, String subtasks) throws ApiException {
ApiResponse resp = getAggregatedSubtaskMetricsWithHttpInfo(jobid, vertexid, get, agg, subtasks);
return resp.getData();
}
/**
*
* Provides access to aggregated subtask metrics.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param vertexid 32-character hexadecimal string value that identifies a job vertex. (required)
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param subtasks Comma-separated list of integer ranges (e.g. \"1,3,5-9\") to select specific subtasks. (optional)
* @return ApiResponse<AggregatedMetricsResponseBody>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAggregatedSubtaskMetricsWithHttpInfo(String jobid, String vertexid, String get, AggregationMode agg, String subtasks) throws ApiException {
okhttp3.Call call = getAggregatedSubtaskMetricsValidateBeforeCall(jobid, vertexid, get, agg, subtasks, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Provides access to aggregated subtask metrics.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param vertexid 32-character hexadecimal string value that identifies a job vertex. (required)
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param subtasks Comma-separated list of integer ranges (e.g. \"1,3,5-9\") to select specific subtasks. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getAggregatedSubtaskMetricsAsync(String jobid, String vertexid, String get, AggregationMode agg, String subtasks, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getAggregatedSubtaskMetricsValidateBeforeCall(jobid, vertexid, get, agg, subtasks, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getAggregatedTaskManagerMetrics
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param taskmanagers Comma-separated list of 32-character hexadecimal strings to select specific task managers. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getAggregatedTaskManagerMetricsCall(String get, AggregationMode agg, String taskmanagers, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/taskmanagers/metrics";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (get != null)
localVarQueryParams.addAll(apiClient.parameterToPair("get", get));
if (agg != null)
localVarQueryParams.addAll(apiClient.parameterToPair("agg", agg));
if (taskmanagers != null)
localVarQueryParams.addAll(apiClient.parameterToPair("taskmanagers", taskmanagers));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getAggregatedTaskManagerMetricsValidateBeforeCall(String get, AggregationMode agg, String taskmanagers, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getAggregatedTaskManagerMetricsCall(get, agg, taskmanagers, progressListener, progressRequestListener);
return call;
}
/**
*
* Provides access to aggregated task manager metrics.
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param taskmanagers Comma-separated list of 32-character hexadecimal strings to select specific task managers. (optional)
* @return AggregatedMetricsResponseBody
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AggregatedMetricsResponseBody getAggregatedTaskManagerMetrics(String get, AggregationMode agg, String taskmanagers) throws ApiException {
ApiResponse resp = getAggregatedTaskManagerMetricsWithHttpInfo(get, agg, taskmanagers);
return resp.getData();
}
/**
*
* Provides access to aggregated task manager metrics.
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param taskmanagers Comma-separated list of 32-character hexadecimal strings to select specific task managers. (optional)
* @return ApiResponse<AggregatedMetricsResponseBody>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAggregatedTaskManagerMetricsWithHttpInfo(String get, AggregationMode agg, String taskmanagers) throws ApiException {
okhttp3.Call call = getAggregatedTaskManagerMetricsValidateBeforeCall(get, agg, taskmanagers, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Provides access to aggregated task manager metrics.
* @param get Comma-separated list of string values to select specific metrics. (optional)
* @param agg Comma-separated list of aggregation modes which should be calculated. Available aggregations are: \"min, max, sum, avg, skew\". (optional)
* @param taskmanagers Comma-separated list of 32-character hexadecimal strings to select specific task managers. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getAggregatedTaskManagerMetricsAsync(String get, AggregationMode agg, String taskmanagers, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getAggregatedTaskManagerMetricsValidateBeforeCall(get, agg, taskmanagers, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getCheckpointConfig
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getCheckpointConfigCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}/checkpoints/config"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getCheckpointConfigValidateBeforeCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getCheckpointConfig(Async)");
}
okhttp3.Call call = getCheckpointConfigCall(jobid, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the checkpointing configuration.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @return CheckpointConfigInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CheckpointConfigInfo getCheckpointConfig(String jobid) throws ApiException {
ApiResponse resp = getCheckpointConfigWithHttpInfo(jobid);
return resp.getData();
}
/**
*
* Returns the checkpointing configuration.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @return ApiResponse<CheckpointConfigInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getCheckpointConfigWithHttpInfo(String jobid) throws ApiException {
okhttp3.Call call = getCheckpointConfigValidateBeforeCall(jobid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the checkpointing configuration.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getCheckpointConfigAsync(String jobid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getCheckpointConfigValidateBeforeCall(jobid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getCheckpointStatisticDetails
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param checkpointid Long value that identifies a checkpoint. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getCheckpointStatisticDetailsCall(String jobid, Long checkpointid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}/checkpoints/details/{checkpointid}"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()))
.replaceAll("\\{" + "checkpointid" + "\\}", apiClient.escapeString(checkpointid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getCheckpointStatisticDetailsValidateBeforeCall(String jobid, Long checkpointid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getCheckpointStatisticDetails(Async)");
}
// verify the required parameter 'checkpointid' is set
if (checkpointid == null) {
throw new ApiException("Missing the required parameter 'checkpointid' when calling getCheckpointStatisticDetails(Async)");
}
okhttp3.Call call = getCheckpointStatisticDetailsCall(jobid, checkpointid, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns details for a checkpoint.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param checkpointid Long value that identifies a checkpoint. (required)
* @return CheckpointStatistics
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CheckpointStatistics getCheckpointStatisticDetails(String jobid, Long checkpointid) throws ApiException {
ApiResponse resp = getCheckpointStatisticDetailsWithHttpInfo(jobid, checkpointid);
return resp.getData();
}
/**
*
* Returns details for a checkpoint.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param checkpointid Long value that identifies a checkpoint. (required)
* @return ApiResponse<CheckpointStatistics>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getCheckpointStatisticDetailsWithHttpInfo(String jobid, Long checkpointid) throws ApiException {
okhttp3.Call call = getCheckpointStatisticDetailsValidateBeforeCall(jobid, checkpointid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns details for a checkpoint.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param checkpointid Long value that identifies a checkpoint. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getCheckpointStatisticDetailsAsync(String jobid, Long checkpointid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getCheckpointStatisticDetailsValidateBeforeCall(jobid, checkpointid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getCheckpointStatus
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getCheckpointStatusCall(String jobid, String triggerid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}/checkpoints/{triggerid}"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()))
.replaceAll("\\{" + "triggerid" + "\\}", apiClient.escapeString(triggerid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getCheckpointStatusValidateBeforeCall(String jobid, String triggerid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getCheckpointStatus(Async)");
}
// verify the required parameter 'triggerid' is set
if (triggerid == null) {
throw new ApiException("Missing the required parameter 'triggerid' when calling getCheckpointStatus(Async)");
}
okhttp3.Call call = getCheckpointStatusCall(jobid, triggerid, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the status of a checkpoint trigger operation.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @return AsynchronousOperationResult
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AsynchronousOperationResult getCheckpointStatus(String jobid, String triggerid) throws ApiException {
ApiResponse resp = getCheckpointStatusWithHttpInfo(jobid, triggerid);
return resp.getData();
}
/**
*
* Returns the status of a checkpoint trigger operation.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @return ApiResponse<AsynchronousOperationResult>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getCheckpointStatusWithHttpInfo(String jobid, String triggerid) throws ApiException {
okhttp3.Call call = getCheckpointStatusValidateBeforeCall(jobid, triggerid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the status of a checkpoint trigger operation.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getCheckpointStatusAsync(String jobid, String triggerid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getCheckpointStatusValidateBeforeCall(jobid, triggerid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getCheckpointingStatistics
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getCheckpointingStatisticsCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}/checkpoints"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getCheckpointingStatisticsValidateBeforeCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getCheckpointingStatistics(Async)");
}
okhttp3.Call call = getCheckpointingStatisticsCall(jobid, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns checkpointing statistics for a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @return CheckpointingStatistics
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CheckpointingStatistics getCheckpointingStatistics(String jobid) throws ApiException {
ApiResponse resp = getCheckpointingStatisticsWithHttpInfo(jobid);
return resp.getData();
}
/**
*
* Returns checkpointing statistics for a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @return ApiResponse<CheckpointingStatistics>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getCheckpointingStatisticsWithHttpInfo(String jobid) throws ApiException {
okhttp3.Call call = getCheckpointingStatisticsValidateBeforeCall(jobid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns checkpointing statistics for a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getCheckpointingStatisticsAsync(String jobid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getCheckpointingStatisticsValidateBeforeCall(jobid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getClusterConfigurationInfo
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getClusterConfigurationInfoCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobmanager/config";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getClusterConfigurationInfoValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getClusterConfigurationInfoCall(progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the cluster configuration.
* @return List<ConfigurationInfoEntry>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List getClusterConfigurationInfo() throws ApiException {
ApiResponse> resp = getClusterConfigurationInfoWithHttpInfo();
return resp.getData();
}
/**
*
* Returns the cluster configuration.
* @return ApiResponse<List<ConfigurationInfoEntry>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> getClusterConfigurationInfoWithHttpInfo() throws ApiException {
okhttp3.Call call = getClusterConfigurationInfoValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the cluster configuration.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getClusterConfigurationInfoAsync(final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getClusterConfigurationInfoValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getClusterDataSetDeleteStatus
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getClusterDataSetDeleteStatusCall(String triggerid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/datasets/delete/{triggerid}"
.replaceAll("\\{" + "triggerid" + "\\}", apiClient.escapeString(triggerid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getClusterDataSetDeleteStatusValidateBeforeCall(String triggerid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'triggerid' is set
if (triggerid == null) {
throw new ApiException("Missing the required parameter 'triggerid' when calling getClusterDataSetDeleteStatus(Async)");
}
okhttp3.Call call = getClusterDataSetDeleteStatusCall(triggerid, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the status for the delete operation of a cluster data set.
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @return AsynchronousOperationResult
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AsynchronousOperationResult getClusterDataSetDeleteStatus(String triggerid) throws ApiException {
ApiResponse resp = getClusterDataSetDeleteStatusWithHttpInfo(triggerid);
return resp.getData();
}
/**
*
* Returns the status for the delete operation of a cluster data set.
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @return ApiResponse<AsynchronousOperationResult>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getClusterDataSetDeleteStatusWithHttpInfo(String triggerid) throws ApiException {
okhttp3.Call call = getClusterDataSetDeleteStatusValidateBeforeCall(triggerid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the status for the delete operation of a cluster data set.
* @param triggerid 32-character hexadecimal string that identifies an asynchronous operation trigger ID. The ID was returned then the operation was triggered. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getClusterDataSetDeleteStatusAsync(String triggerid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getClusterDataSetDeleteStatusValidateBeforeCall(triggerid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getClusterDataSetList
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getClusterDataSetListCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/datasets";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getClusterDataSetListValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getClusterDataSetListCall(progressListener, progressRequestListener);
return call;
}
/**
*
* Returns all cluster data sets.
* @return ClusterDataSetListResponseBody
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ClusterDataSetListResponseBody getClusterDataSetList() throws ApiException {
ApiResponse resp = getClusterDataSetListWithHttpInfo();
return resp.getData();
}
/**
*
* Returns all cluster data sets.
* @return ApiResponse<ClusterDataSetListResponseBody>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getClusterDataSetListWithHttpInfo() throws ApiException {
okhttp3.Call call = getClusterDataSetListValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns all cluster data sets.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getClusterDataSetListAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getClusterDataSetListValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getClusterOverview
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getClusterOverviewCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/overview";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getClusterOverviewValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getClusterOverviewCall(progressListener, progressRequestListener);
return call;
}
/**
*
* Returns an overview over the Flink cluster.
* @return ClusterOverviewWithVersion
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ClusterOverviewWithVersion getClusterOverview() throws ApiException {
ApiResponse resp = getClusterOverviewWithHttpInfo();
return resp.getData();
}
/**
*
* Returns an overview over the Flink cluster.
* @return ApiResponse<ClusterOverviewWithVersion>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getClusterOverviewWithHttpInfo() throws ApiException {
okhttp3.Call call = getClusterOverviewValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns an overview over the Flink cluster.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getClusterOverviewAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getClusterOverviewValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getDashboardConfiguration
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getDashboardConfigurationCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/config";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getDashboardConfigurationValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getDashboardConfigurationCall(progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the configuration of the WebUI.
* @return DashboardConfiguration
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DashboardConfiguration getDashboardConfiguration() throws ApiException {
ApiResponse resp = getDashboardConfigurationWithHttpInfo();
return resp.getData();
}
/**
*
* Returns the configuration of the WebUI.
* @return ApiResponse<DashboardConfiguration>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getDashboardConfigurationWithHttpInfo() throws ApiException {
okhttp3.Call call = getDashboardConfigurationValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the configuration of the WebUI.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getDashboardConfigurationAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getDashboardConfigurationValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getJarList
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getJarListCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jars";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getJarListValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getJarListCall(progressListener, progressRequestListener);
return call;
}
/**
*
* Returns a list of all jars previously uploaded via '/jars/upload'.
* @return JarListInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public JarListInfo getJarList() throws ApiException {
ApiResponse resp = getJarListWithHttpInfo();
return resp.getData();
}
/**
*
* Returns a list of all jars previously uploaded via '/jars/upload'.
* @return ApiResponse<JarListInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getJarListWithHttpInfo() throws ApiException {
okhttp3.Call call = getJarListValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns a list of all jars previously uploaded via '/jars/upload'.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getJarListAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getJarListValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getJobAccumulators
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param includeSerializedValue Boolean value that specifies whether serialized user task accumulators should be included in the response. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getJobAccumulatorsCall(String jobid, Boolean includeSerializedValue, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}/accumulators"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (includeSerializedValue != null)
localVarQueryParams.addAll(apiClient.parameterToPair("includeSerializedValue", includeSerializedValue));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getJobAccumulatorsValidateBeforeCall(String jobid, Boolean includeSerializedValue, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getJobAccumulators(Async)");
}
okhttp3.Call call = getJobAccumulatorsCall(jobid, includeSerializedValue, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the accumulators for all tasks of a job, aggregated across the respective subtasks.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param includeSerializedValue Boolean value that specifies whether serialized user task accumulators should be included in the response. (optional)
* @return JobAccumulatorsInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public JobAccumulatorsInfo getJobAccumulators(String jobid, Boolean includeSerializedValue) throws ApiException {
ApiResponse resp = getJobAccumulatorsWithHttpInfo(jobid, includeSerializedValue);
return resp.getData();
}
/**
*
* Returns the accumulators for all tasks of a job, aggregated across the respective subtasks.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param includeSerializedValue Boolean value that specifies whether serialized user task accumulators should be included in the response. (optional)
* @return ApiResponse<JobAccumulatorsInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getJobAccumulatorsWithHttpInfo(String jobid, Boolean includeSerializedValue) throws ApiException {
okhttp3.Call call = getJobAccumulatorsValidateBeforeCall(jobid, includeSerializedValue, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the accumulators for all tasks of a job, aggregated across the respective subtasks.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param includeSerializedValue Boolean value that specifies whether serialized user task accumulators should be included in the response. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getJobAccumulatorsAsync(String jobid, Boolean includeSerializedValue, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getJobAccumulatorsValidateBeforeCall(jobid, includeSerializedValue, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getJobConfig
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getJobConfigCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}/config"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getJobConfigValidateBeforeCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getJobConfig(Async)");
}
okhttp3.Call call = getJobConfigCall(jobid, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns the configuration of a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @return JobConfigInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public JobConfigInfo getJobConfig(String jobid) throws ApiException {
ApiResponse resp = getJobConfigWithHttpInfo(jobid);
return resp.getData();
}
/**
*
* Returns the configuration of a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @return ApiResponse<JobConfigInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getJobConfigWithHttpInfo(String jobid) throws ApiException {
okhttp3.Call call = getJobConfigValidateBeforeCall(jobid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the configuration of a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getJobConfigAsync(String jobid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getJobConfigValidateBeforeCall(jobid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getJobDetails
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getJobDetailsCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/jobs/{jobid}"
.replaceAll("\\{" + "jobid" + "\\}", apiClient.escapeString(jobid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.setHttpClient(apiClient.getHttpClient().newBuilder().addInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
}).build());
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getJobDetailsValidateBeforeCall(String jobid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'jobid' is set
if (jobid == null) {
throw new ApiException("Missing the required parameter 'jobid' when calling getJobDetails(Async)");
}
okhttp3.Call call = getJobDetailsCall(jobid, progressListener, progressRequestListener);
return call;
}
/**
*
* Returns details of a job.
* @param jobid 32-character hexadecimal string value that identifies a job. (required)
* @return JobDetailsInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public JobDetailsInfo getJobDetails(String jobid) throws ApiException {
ApiResponse