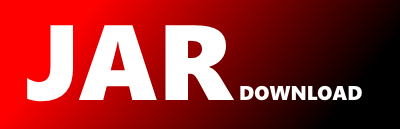
com.nextpls.sdk.secure.RsaKeyGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Use to connect to the nextpls
The newest version!
package com.nextpls.sdk.secure;
import com.nextpls.sdk.utils.ExceptionUtil;
import org.apache.commons.codec.binary.Base64;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.math.BigInteger;
import java.security.Key;
import java.security.KeyFactory;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.interfaces.RSAPrivateKey;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.RSAPrivateKeySpec;
import java.security.spec.RSAPublicKeySpec;
import java.util.Map;
public class RsaKeyGenerator {
private static Logger logger = LoggerFactory.getLogger(RsaKeyGenerator.class);
public static final String RSA_ALGORITHM = "RSA";
private static final String PUBLIC_KEY = "RSAPublicKey";
private static final String PRIVATE_KEY = "RSAPrivateKey";
//get RSA public key
public static String getPublicKey(Map keyMap) throws Exception {
Key key = (Key) keyMap.get(PUBLIC_KEY);
return encryptBASE64(key.getEncoded());
}
//get RSA private key
public static String getPrivateKey(Map keyMap) throws Exception {
Key key = (Key) keyMap.get(PRIVATE_KEY);
return encryptBASE64(key.getEncoded());
}
//
static byte[] decryptBASE64(String key) {
return Base64.decodeBase64(key.getBytes());
}
//
static String encryptBASE64(byte[] key) {
return Base64.encodeBase64String(key);
}
/**
* 使用模和指数生成RSA公钥
* 注意:此代码用了默认补位方式,为RSA/None/PKCS1Padding,不同JDK默认的补位方式可能不同,如Android默认是RSA/None/NoPadding
*
* @param modulus 模
* @param exponent 指数
* @return
*/
private static RSAPublicKey getPublicKey(String modulus, String exponent) {
try {
BigInteger b1 = new BigInteger(modulus);
BigInteger b2 = new BigInteger(exponent);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
RSAPublicKeySpec keySpec = new RSAPublicKeySpec(b1, b2);
return (RSAPublicKey) keyFactory.generatePublic(keySpec);
} catch (Exception e) {
logger.error(ExceptionUtil.toString(e));
return null;
}
}
/**
* 使用模和指数生成RSA私钥
* 注意:此代码用了默认补位方式,为RSA/None/PKCS1Padding,不同JDK默认的补位方式可能不同,如Android默认是RSA/None/NoPadding
*
* @param modulus 模
* @param exponent 指数
* @return
*/
private static RSAPrivateKey getPrivateKey(String modulus, String exponent) {
try {
BigInteger b1 = new BigInteger(modulus);
BigInteger b2 = new BigInteger(exponent);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
RSAPrivateKeySpec keySpec = new RSAPrivateKeySpec(b1, b2);
return (RSAPrivateKey) keyFactory.generatePrivate(keySpec);
} catch (Exception e) {
logger.error(ExceptionUtil.toString(e));
return null;
}
}
//
public static RsaKeypair getRsaKeypair() throws Exception {
//KeyPairGenerator RSA 1024bytes
KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance(RSA_ALGORITHM);
keyPairGen.initialize(1024);
KeyPair keyPair = keyPairGen.generateKeyPair();
RSAPublicKey publicKey = (RSAPublicKey) keyPair.getPublic();
RSAPrivateKey privateKey = (RSAPrivateKey) keyPair.getPrivate();
//mod
//String modulus = publicKey.getModulus().toString();
//public and private expo
//String public_exponent = publicKey.getPublicExponent().toString();
//String private_exponent = privateKey.getPrivateExponent().toString();
//RSAPublicKey pubKey = getPublicKey(modulus, public_exponent);
//RSAPrivateKey priKey = getPrivateKey(modulus, private_exponent);
return new RsaKeypair(publicKey, privateKey);
}
public static void main(String[] args) {
try {
RsaKeypair keypair = getRsaKeypair();
System.out.println(keypair.getPrivateKeyStr());
System.out.println(keypair.getPublicKeyStr());
} catch (Exception e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy