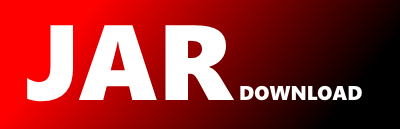
com.nhl.bootique.BQBinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bootique Show documentation
Show all versions of bootique Show documentation
Bootique is a simple DI-based framework for launching command-line Java applications
of any kind. Be it webservices, webapps, jobs, etc.
package com.nhl.bootique;
import java.util.Arrays;
import java.util.Collection;
import com.google.common.base.Preconditions;
import com.google.inject.Binder;
import com.google.inject.multibindings.MapBinder;
import com.google.inject.multibindings.Multibinder;
import com.nhl.bootique.annotation.EnvironmentProperties;
import com.nhl.bootique.cli.CliOption;
import com.nhl.bootique.command.Command;
/**
* A helper class used inside Modules that allows to contribute commands and
* properties to Bootique runtime. Create and invoke this binder inside your
* Module's "configure" method to provide your own properties and/or Commands.
*
* @since 0.8
* @deprecated since 0.12 per-module binding utilities are associated with the
* Module itself, so use
* {@link BQCoreModule#contributeCommands(Binder)},
* {@link BQCoreModule#contributeOptions(Binder)},
* {@link BQCoreModule#contributeProperties(Binder)}.
*/
@Deprecated
public class BQBinder {
public static BQBinder contributeTo(Binder binder) {
return new BQBinder(binder);
}
private Binder binder;
BQBinder(Binder binder) {
this.binder = binder;
}
MapBinder propsBinder() {
return MapBinder.newMapBinder(binder, String.class, String.class, EnvironmentProperties.class);
}
Multibinder commandsBinder() {
return Multibinder.newSetBinder(binder, Command.class);
}
Multibinder optionsBinder() {
return Multibinder.newSetBinder(binder, CliOption.class);
}
/**
* Utility method to contribute custom environment properties to DI.
*
* @param key
* environment parameter name.
* @param value
* environment parameter value.
*/
public void property(String key, String value) {
propsBinder().addBinding(key).toInstance(value);
}
/**
* Utility method to contribute custom commands to DI.
*
* @param commands
* {@link Command} types for singleton commands to add to
* Bootique.
*/
@SafeVarargs
public final void commandTypes(Class extends Command>... commands) {
commandTypes(Arrays.asList(Preconditions.checkNotNull(commands)));
}
/**
* Utility method to contribute custom commands to DI.
*
* @param commands
* {@link Command} types for singleton commands to add to
* Bootique.
*/
public void commandTypes(Collection> commands) {
Multibinder commandBinder = commandsBinder();
Preconditions.checkNotNull(commands).forEach(ct -> commandBinder.addBinding().to(ct));
}
/**
* Utility method to contribute custom commands to DI.
*
* @param commands
* {@link Command} instances to add to Bootique.
*/
public void commands(Collection extends Command> commands) {
Multibinder commandBinder = commandsBinder();
Preconditions.checkNotNull(commands).forEach(c -> commandBinder.addBinding().toInstance(c));
}
/**
* Binds global options not explicitly associated with Commands
*
* @since 0.12
* @param options
* an array of options to recognize when parsing command line,
* which should be merged into existing set of options.
*/
public void options(CliOption... options) {
options(Arrays.asList(Preconditions.checkNotNull(options)));
}
/**
* Binds global options not explicitly associated with Commands
*
* @since 0.12
* @param options
* a collection of options to recognize when parsing command
* line, which should be merged into existing set of options.
*/
public void options(Collection options) {
Multibinder optionsBinder = optionsBinder();
Preconditions.checkNotNull(options).forEach(o -> optionsBinder.addBinding().toInstance(o));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy