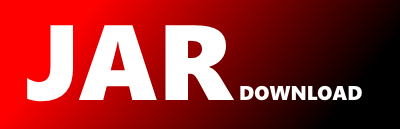
com.nhl.link.rest.meta.compiler.PojoEntityCompiler Maven / Gradle / Ivy
package com.nhl.link.rest.meta.compiler;
import com.nhl.link.rest.LinkRestException;
import com.nhl.link.rest.meta.DefaultLrEntity;
import com.nhl.link.rest.meta.LrDataMap;
import com.nhl.link.rest.meta.LrEntity;
import com.nhl.link.rest.meta.LrEntityBuilder;
import com.nhl.link.rest.meta.LrEntityOverlay;
import org.apache.cayenne.di.Inject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.ws.rs.core.Response;
import java.util.Map;
/**
* @since 1.24
*/
public class PojoEntityCompiler implements LrEntityCompiler {
private static final Logger LOGGER = LoggerFactory.getLogger(PojoEntityCompiler.class);
private Map entityOverlays;
public PojoEntityCompiler(@Inject Map entityOverlays) {
this.entityOverlays = entityOverlays;
}
@Override
public LrEntity compile(Class type, LrDataMap dataMap) {
return new LazyLrEntity<>(type, () -> doCompile(type, dataMap));
}
private LrEntity doCompile(Class type, LrDataMap dataMap) {
LOGGER.debug("compiling entity of type {}", type);
DefaultLrEntity entity = loadAnnotatedProperties(type, dataMap);
loadOverlays(entity, dataMap);
checkEntityValid(entity);
return entity;
}
protected DefaultLrEntity loadAnnotatedProperties(Class type, LrDataMap dataMap) {
return new LrEntityBuilder<>(type, dataMap).build();
}
protected void loadOverlays(DefaultLrEntity entity, LrDataMap dataMap) {
LrEntityOverlay> overlay = entityOverlays.get(entity.getType().getName());
if (overlay != null) {
overlay.getAttributes().forEach(entity::addAttribute);
overlay.getRelatonships(dataMap).forEach(entity::addRelationship);
}
}
protected void checkEntityValid(LrEntity entity) {
if (entity.getIds().isEmpty() && entity.getAttributes().isEmpty() && entity.getRelationships().isEmpty()) {
throw new LinkRestException(Response.Status.INTERNAL_SERVER_ERROR,
"Invalid entity '" + entity.getType().getName() + "' - no properties");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy