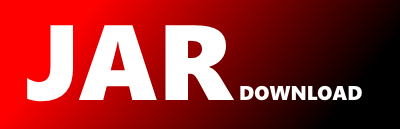
com.nimbusds.openid.connect.provider.spi.grants.AccessTokenSpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of c2id-server-sdk Show documentation
Show all versions of c2id-server-sdk Show documentation
Toolkit for developing Connect2id Server extensions, such as
custom OpenID Connect claims sources and grant handlers.
package com.nimbusds.openid.connect.provider.spi.grants;
import java.util.List;
import com.nimbusds.oauth2.sdk.ParseException;
import com.nimbusds.oauth2.sdk.id.Audience;
import com.nimbusds.oauth2.sdk.id.Subject;
import com.nimbusds.oauth2.sdk.token.TokenEncoding;
import com.nimbusds.oauth2.sdk.util.JSONObjectUtils;
import net.jcip.annotations.Immutable;
import net.minidev.json.JSONObject;
/**
* Access token specification..
*/
@Immutable
public class AccessTokenSpec extends TokenSpec {
/**
* Default access token specification. No explicit token lifetime is
* specified (to let the Connect2id server apply the default configured
* lifetime for access tokens). No explicit token audience is
* specified. No subject in impersonation and delegation cases is
* specified. The token is self-contained (JWT-encoded) and not
* encrypted.
*/
public static final AccessTokenSpec DEFAULT = new AccessTokenSpec();
/**
* The access token encoding.
*/
private final TokenEncoding encoding;
/**
* If {@code true} flags the access token for encryption. Applies to
* self-contained access tokens only.
*/
private final boolean encrypt;
/**
* Creates a new default access token specification. No explicit
* token lifetime is specified (to let the Connect2id server apply the
* default configured lifetime for access tokens). No explicit token
* audience is specified. No subject in impersonation and delegation
* cases is specified. The token is self-contained (JWT-encoded) and
* not encrypted.
*/
public AccessTokenSpec() {
this(0L, null, TokenEncoding.SELF_CONTAINED, null, false);
}
/**
* Creates a new access token specification.
*
* @param lifetime The access token lifetime, in seconds,
* zero if not specified (to let the
* Connect2id server apply the default
* configured lifetime for access tokens).
* @param audList Explicit list of audiences for the access
* token, {@code null} if not specified.
* @param encoding The access token encoding. Must not be
* {@code null}.
* @param impersonatedSubject The subject in impersonation and
* delegation cases, {@code null} if not
* applicable.
* @param encrypt If {@code true} flags the access token
* for encryption. Applies to self-contained
* (JWT) access tokens only.
*/
public AccessTokenSpec(final long lifetime,
final List audList,
final TokenEncoding encoding,
final Subject impersonatedSubject,
final boolean encrypt) {
super(lifetime, audList, impersonatedSubject);
if (encoding == null) {
throw new IllegalArgumentException("The access token encoding must not be null");
}
this.encoding = encoding;
// Only JWT tokens may be encrypted
this.encrypt = encoding.equals(TokenEncoding.SELF_CONTAINED) && encrypt;
}
/**
* Creates a new access token specification. No subject in
* impersonation and delegation cases is specified.
*
* @param lifetime The access token lifetime, in seconds, zero if not
* specified (to let the Connect2id server apply the
* default configured lifetime for access tokens).
* @param audList Explicit list of audiences for the access token,
* {@code null} if not specified.
* @param encoding The access token encoding. Must not be {@code null}.
* @param encrypt If {@code true} flags the access token for
* encryption. Applies to self-contained (JWT) access
* tokens only.
*/
public AccessTokenSpec(final long lifetime,
final List audList,
final TokenEncoding encoding,
final boolean encrypt) {
this(lifetime, audList, encoding, null, encrypt);
}
/**
* Creates a new access token specification. No explicit token audience
* is specified. No subject in impersonation and delegation cases is
* specified.
*
* @param lifetime The access token lifetime, in seconds, zero if not
* specified (to let the Connect2id server apply the
* default configured lifetime for access tokens).
* @param encoding The access token encoding. Must not be {@code null}.
* @param encrypt If {@code true} flags the access token for
* encryption. Applies to self-contained (JWT) access
* tokens only.
*/
public AccessTokenSpec(final long lifetime,
final TokenEncoding encoding,
final boolean encrypt) {
this(lifetime, null, encoding, null, encrypt);
}
/**
* Returns the access token encoding.
*
* @return The access token encoding.
*/
public TokenEncoding getEncoding() {
return encoding;
}
/**
* Returns the access token encryption flag.
*
* @return If {@code true} the access token is flagged for encryption.
* Applies to self-contained access tokens only.
*/
public boolean encrypt() {
return encrypt;
}
@Override
public JSONObject toJSONObject() {
JSONObject o = super.toJSONObject();
o.put("encoding", encoding.toString());
if (encoding.equals(TokenEncoding.SELF_CONTAINED)) {
o.put("encrypt", encrypt);
}
return o;
}
/**
* Parses an access token specification from the specified JSON object.
*
* @param jsonObject The JSON object. Must not be {@code null}.
*
* @return The access token specification.
*
* @throws ParseException If parsing failed.
*/
public static AccessTokenSpec parse(final JSONObject jsonObject)
throws ParseException {
TokenSpec tokenSpec = TokenSpec.parse(jsonObject);
TokenEncoding encoding = TokenEncoding.SELF_CONTAINED;
boolean encrypt = false;
if (jsonObject.containsKey("encoding")) {
String c = JSONObjectUtils.getString(jsonObject, "encoding");
try {
encoding = TokenEncoding.valueOf(c.toUpperCase());
} catch (IllegalArgumentException e) {
throw new ParseException("Invalid access token encoding");
}
}
if (encoding.equals(TokenEncoding.SELF_CONTAINED)) {
if (jsonObject.containsKey("encrypt")) {
encrypt = JSONObjectUtils.getBoolean(jsonObject, "encrypt");
}
}
return new AccessTokenSpec(tokenSpec.getLifetime(), tokenSpec.getAudience(), encoding, tokenSpec.getImpersonatedSubject(), encrypt);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy