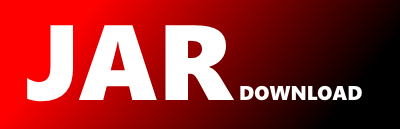
com.nimbusds.openid.connect.provider.spi.grants.GrantAuthorization Maven / Gradle / Ivy
Show all versions of c2id-server-sdk Show documentation
package com.nimbusds.openid.connect.provider.spi.grants;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import net.jcip.annotations.Immutable;
import net.minidev.json.JSONObject;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.collections4.MapUtils;
import com.nimbusds.oauth2.sdk.ParseException;
import com.nimbusds.oauth2.sdk.Scope;
import com.nimbusds.oauth2.sdk.id.Audience;
import com.nimbusds.oauth2.sdk.util.JSONObjectUtils;
/**
* Basic OAuth 2.0 authorisation produced by a {@link GrantHandler}.
*
* Required authorisation details:
*
*
* - The authorised scope.
*
*
* All other parameters are optional or have suitable defaults.
*/
@Immutable
public class GrantAuthorization {
/**
* The authorised scope values.
*/
private final Scope scope;
/**
* The access token specification.
*/
private final AccessTokenSpec accessTokenSpec;
/**
* Optional authorisation data as a JSON object, {@code null} if not
* specified.
*/
private final JSONObject data;
/**
* Creates a new basic authorisation.
*
* @param scope The authorised scope values. Must not be {@code null}.
*/
public GrantAuthorization(final Scope scope) {
this(scope, AccessTokenSpec.DEFAULT, null);
}
/**
* Creates a new basic authorisation.
*
* @param scope The authorised scope values. Must not be
* {@code null}.
* @param accessTokenSpec The access token specification. Must not be
* {@code null}.
* @param data Additional data as a JSON object,
* {@code null} if not specified.
*/
public GrantAuthorization(final Scope scope,
final AccessTokenSpec accessTokenSpec,
final JSONObject data) {
if (scope == null) {
throw new IllegalArgumentException("The scope must not be null");
}
this.scope = scope;
if (accessTokenSpec == null) {
throw new IllegalArgumentException("The access token specification must not be null");
}
this.accessTokenSpec = accessTokenSpec;
this.data = data;
}
/**
* Creates a new basic authorisation.
*
* @param scope The authorised scope values. Must not be
* {@code null}.
* @param audList Explicit list of audiences for the access
* token, {@code null} if not specified.
* @param accessTokenSpec The access token specification. Must not be
* {@code null}.
* @param data Additional data as a JSON object,
* {@code null} if not specified.
*/
public GrantAuthorization(final Scope scope,
final List audList,
final AccessTokenSpec accessTokenSpec,
final JSONObject data) {
this(scope,
new AccessTokenSpec(
accessTokenSpec.getLifetime(),
audList, // override with top-level parameter, backward compat API
accessTokenSpec.getEncoding(),
accessTokenSpec.encrypt()),
data);
}
/**
* Returns the authorised scope values.
*
* @return The authorised scope values.
*/
public Scope getScope() {
return scope;
}
/**
* Returns the explicit list of audiences for the access token.
*
* @return The explicit list of audiences for the access token,
* {@code null} if not specified.
*/
public List getAudience() {
return getAccessTokenSpec().getAudience();
}
/**
* Returns the access token specification.
*
* @return The access token specification.
*/
public AccessTokenSpec getAccessTokenSpec() {
return accessTokenSpec;
}
/**
* Returns the additional data as a JSON object.
*
* @return The additional data, {@code null} if not specified.
*/
public JSONObject getData() {
return data;
}
/**
* Returns a JSON object representation of this authorisation.
*
* @return The JSON object representation.
*/
public JSONObject toJSONObject() {
JSONObject o = new JSONObject();
o.put("scope", scope.toStringList());
JSONObject accessTokenSpecJSONObject = accessTokenSpec.toJSONObject();
// Backward API compat
if (CollectionUtils.isNotEmpty(getAccessTokenSpec().getAudience())) {
o.put("audience", accessTokenSpecJSONObject.remove("audience"));
}
o.put("access_token", accessTokenSpecJSONObject);
if (MapUtils.isNotEmpty(data)) {
o.put("data", data);
}
return o;
}
/**
* Parses a basic authorisation from the specified JSON object.
*
* @param jsonObject The JSON object to parse. Must not be
* {@code null}.
*
* @return The basic authorisation.
*
* @throws ParseException If parsing failed.
*/
public static GrantAuthorization parse(final JSONObject jsonObject)
throws ParseException {
Scope scope = Scope.parse(Arrays.asList(JSONObjectUtils.getStringArray(jsonObject, "scope")));
// Backward API compat
List topLevelAudList = null;
if (jsonObject.containsKey("audience")) {
String[] sa = JSONObjectUtils.getStringArray(jsonObject, "audience");
topLevelAudList = new ArrayList<>(sa.length);
for (String s: sa) {
topLevelAudList.add(new Audience(s));
}
}
AccessTokenSpec accessTokenSpec;
if (jsonObject.containsKey("access_token")) {
// Parse
accessTokenSpec = AccessTokenSpec.parse(JSONObjectUtils.getJSONObject(jsonObject, "access_token"));
if (topLevelAudList != null) {
accessTokenSpec = new AccessTokenSpec(
accessTokenSpec.getLifetime(),
topLevelAudList, // Backward API compat
accessTokenSpec.getEncoding(),
accessTokenSpec.encrypt());
}
} else {
// Apply default settings
accessTokenSpec = new AccessTokenSpec();
}
JSONObject data = null;
if (jsonObject.containsKey("data")) {
data = JSONObjectUtils.getJSONObject(jsonObject, "data");
}
return new GrantAuthorization(scope, accessTokenSpec, data);
}
/**
* Parses a basic authorisation from the specified JSON object string.
*
* @param json The JSON object string to parse. Must not be
* {@code null}.
*
* @return The basic authorisation.
*
* @throws ParseException If parsing failed.
*/
public static GrantAuthorization parse(final String json)
throws ParseException {
return parse(JSONObjectUtils.parse(json));
}
}