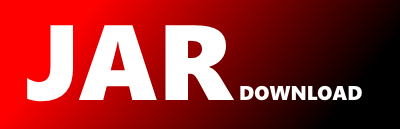
com.nimbusds.openid.connect.provider.spi.grants.OptionalTokenSpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of c2id-server-sdk Show documentation
Show all versions of c2id-server-sdk Show documentation
Toolkit for developing Connect2id Server extensions, such as
custom OpenID Connect claims sources and grant handlers.
package com.nimbusds.openid.connect.provider.spi.grants;
import java.util.List;
import com.nimbusds.oauth2.sdk.ParseException;
import com.nimbusds.oauth2.sdk.id.Audience;
import com.nimbusds.oauth2.sdk.id.Subject;
import com.nimbusds.oauth2.sdk.util.JSONObjectUtils;
import net.jcip.annotations.Immutable;
import net.minidev.json.JSONObject;
/**
* Optional token specification.
*/
@Immutable
public class OptionalTokenSpec extends TokenSpec {
/**
* Specifies if a token is to be issued. If {@code true} a token must
* be issued, {@code false} to prohibit issue.
*/
private final boolean issue;
/**
* Creates a new optional token specification.
*
* @param issue If {@code true} a token must be issued,
* {@code false} to prohibit issue.
* @param lifetime The token lifetime, in seconds. Zero
* implies permanent or not specified (to
* apply the default configured token
* lifetime), depending on the token type.
* Must not be negative integer.
* @param audList Explicit list of audiences for the token,
* {@code null} if not specified.
* @param impersonatedSubject The subject in impersonation and
* delegation cases, {@code null} if not
* applicable.
*/
public OptionalTokenSpec(final boolean issue,
final long lifetime,
final List audList,
final Subject impersonatedSubject) {
super(lifetime, audList, impersonatedSubject);
this.issue = issue;
}
/**
* Returns the token issue policy.
*
* @return {@code true} if a token must be issued, {@code false} to
* prohibit issue.
*/
public boolean issue() {
return issue;
}
@Override
public JSONObject toJSONObject() {
JSONObject o = super.toJSONObject();
o.put("issue", issue);
return o;
}
/**
* Parses an optional token specification from the specified JSON
* object.
*
* @param jsonObject The JSON object. Must not be {@code null}.
*
* @return The optional token specification.
*
* @throws ParseException If parsing failed.
*/
public static OptionalTokenSpec parse(final JSONObject jsonObject)
throws ParseException {
TokenSpec tokenSpec = TokenSpec.parse(jsonObject);
boolean issue = false;
if (jsonObject.containsKey("issue")) {
issue = JSONObjectUtils.getBoolean(jsonObject, "issue");
}
return new OptionalTokenSpec(issue, tokenSpec.getLifetime(), tokenSpec.getAudience(), tokenSpec.getImpersonatedSubject());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy