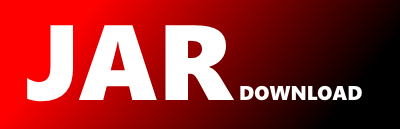
com.nimbusds.openid.connect.provider.spi.grants.SelfIssuedJWTGrantHandler Maven / Gradle / Ivy
Show all versions of c2id-server-sdk Show documentation
package com.nimbusds.openid.connect.provider.spi.grants;
import com.nimbusds.jwt.JWTClaimsSet;
import com.nimbusds.oauth2.sdk.GeneralException;
import com.nimbusds.oauth2.sdk.Scope;
import com.nimbusds.oauth2.sdk.id.ClientID;
import com.nimbusds.openid.connect.sdk.rp.OIDCClientMetadata;
/**
* Service Provider Interface (SPI) for handling self-issued JSON Web Token
* (JWT) bearer assertion grants. Returns the matching
* {@link SelfIssuedAssertionAuthorization authorisation} on success.
*
* The handler should not specify access token lifetimes that exceed the
* validity period of the JWT assertion by a significant period. The issue of
* refresh tokens is not permitted. Clients can refresh an expired access token
* by requesting a new one using the same assertion, if it is still valid, or
* with a new assertion.
*
*
Implementations must be thread-safe.
*
*
Related specifications:
*
*
* - Assertion Framework for OAuth 2.0 Client Authentication and
* Authorization Grants (RFC 7521), section 4.1.
*
- JSON Web Token (JWT) Profile for OAuth 2.0 Client Authentication and
* Authorization Grants (RFC 7523), sections 2.1, 3 and 3.1.
*
*/
public interface SelfIssuedJWTGrantHandler extends JWTGrantHandler {
/**
* Handles a self-issued JWT bearer assertion grant by a client
* registered with the Connect2id server.
*
* This method is called for JWT assertion grants which fulfil all
* of the following conditions:
*
*
* - Are issued by a client which is registered with the
* Connect2id server, i.e. the JWT issuer (iss) assertion
* matches a registered client_id;
*
- The client is registered for the
* {@code urn:ietf:params:oauth:grant-type:jwt-bearer} grant;
*
- The client is successfully authenticated, by means of
* separate client authentication included in the token request
* (client_secret_basic, client_secret_post, client_secret_jwt
* or private_key_jwt), and / or with the JWT assertion grant
* itself;
*
- The JWT MAC or signature was successfully verified using
* with a registered {@code client_secret} or {@code jwks} /
* {@code jwks_uri};
*
- The JWT audience (aud), expiration (exp) and not-before
* time (nbf) claims verify successfully.
*
*
* If the requested scope is invalid, unknown, malformed, or exceeds
* the scope granted by the resource owner the handler must throw a
* {@link GeneralException} with an
* {@link com.nimbusds.oauth2.sdk.OAuth2Error#INVALID_SCOPE
* invalid_scope} error code.
*
* @param jwtClaimsSet The claims set included in the verified JWT
* assertion grant. The audience (aud),
* expiration (exp) and not-before time (nbf)
* claims are verified by the Connect2id server.
* The issuer (iss) claims will equal the
* client_id. Not {@code null}.
* @param scope The requested scope, {@code null} if not
* specified.
* @param clientID The identifier of the authenticated client.
* Not {@code null}.
* @param clientMetadata The OAuth 2.0 / OpenID Connect metadata for
* the client. Not {@code null}.
*
* @return The authorisation.
*
* @throws GeneralException If the grant is invalid, or another
* exception was encountered.
*/
SelfIssuedAssertionAuthorization processSelfIssuedGrant(final JWTClaimsSet jwtClaimsSet,
final Scope scope,
final ClientID clientID,
final OIDCClientMetadata clientMetadata)
throws GeneralException;
}