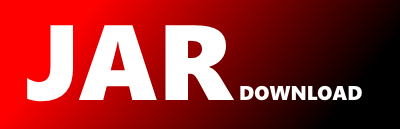
com.nimbusds.openid.connect.provider.spi.grants.ThirdPartyJWTGrantHandler Maven / Gradle / Ivy
Show all versions of c2id-server-sdk Show documentation
package com.nimbusds.openid.connect.provider.spi.grants;
import com.nimbusds.jose.JOSEObject;
import com.nimbusds.oauth2.sdk.GeneralException;
import com.nimbusds.oauth2.sdk.Scope;
import com.nimbusds.oauth2.sdk.id.ClientID;
import com.nimbusds.openid.connect.sdk.rp.OIDCClientMetadata;
/**
* Service Provider Interface (SPI) for handling JSON Web Token (JWT) assertion
* grants issued by a third-party security token service. Returns the matching
* {@link ThirdPartyAssertionAuthorization authorisation} on success. Must
* throw a {@link GeneralException} with an
* {@link com.nimbusds.oauth2.sdk.OAuth2Error#INVALID_GRANT invalid_grant}
* error code if the JWT assertion is invalid.
*
* The passed JWT assertion can be an instance of:
*
*
* - {@link com.nimbusds.jwt.SignedJWT} -- Signed or MAC protected with
* JWS;
*
- {@link com.nimbusds.jwt.EncryptedJWT} -- Encrypted with JWE;
*
- {@link com.nimbusds.jose.JWEObject} -- Signed or MAC protected with
* JWS, then encrypted with JWE.
*
*
* The handler should not specify access token lifetimes that exceed the
* validity period of the JWT assertion by a significant period. The issue of
* refresh tokens is not permitted. Clients can refresh an expired access token
* by requesting a new one using the same assertion, if it is still valid, or
* with a new assertion.
*
*
Implementations must be thread-safe.
*
*
Related specifications:
*
*
* - Assertion Framework for OAuth 2.0 Client Authentication and
* Authorization Grants (RFC 7521), section 4.1.
*
- JSON Web Token (JWT) Profile for OAuth 2.0 Client Authentication and
* Authorization Grants (RFC 7523), sections 2.1, 3 and 3.1.
*
*/
public interface ThirdPartyJWTGrantHandler extends JWTGrantHandler {
/**
* Handles a JWT bearer assertion grant issued by a third-party
* security token service (STS). The grant handler must verify the JWT
* assertion, using a previously agreed method to resolve the client's
* MAC or signature key.
*
* The following client authentication / identification cases may be
* handled:
*
*
* - Confidential client:
* If the client is confidential and has provided valid
* authentication (client_secret_basic, client_secret_post,
* client_secret_jwt or private_key_jwt) the
* {@code confidentialClient} flag will be {@code true}. The
* client_id and metadata arguments will be set.
*
- Public client:
* If the client is public and has a provided its registered
* {@code client_id} using the optional token request
* parameter, the {@code confidentialClient} flag will be
* {@code false} and the client metadata will be set.
*
- Handler must resolve client_id from JWT claims:
* If no client authentication or {@code client_id} is passed
* with the token request, the client information arguments
* will be {@code null} and the {@code confidentialClient} flag
* will be {@code false}. The grant handler must resolve the
* {@code client_id} for the authorisation result from claims
* of the JWT assertion. If such a use case is not supported or
* permitted the grant handler should throw a
* {@link GeneralException} with an
* {@link com.nimbusds.oauth2.sdk.OAuth2Error#INVALID_REQUEST
* invalid_request} error.
*
*
* If the JWT assertion is invalid the handler must throw a
* {@link GeneralException} with an
* {@link com.nimbusds.oauth2.sdk.OAuth2Error#INVALID_GRANT
* invalid_grant} error code.
*
*
If the requested scope is invalid, unknown, malformed, or exceeds
* the scope granted by the resource owner the handler must throw a
* {@link GeneralException} with an
* {@link com.nimbusds.oauth2.sdk.OAuth2Error#INVALID_SCOPE
* invalid_scope} error code.
*
* @param jwtAssertion The JWT assertion, to be verified /
* decrypted by the handler. Can be a signed
* JWT, an encrypted JWT, or a signed and
* encrypted (nested) JWT. Not {@code null}.
* @param scope The requested scope, {@code null} if not
* specified.
* @param clientID The client identifier, {@code null} if not
* specified or if no client authentication
* was provided.
* @param confidentialClient {@code true} if the client is confidential
* and has been authenticated, else
* {@code false}.
* @param clientMetadata The OAuth 2.0 / OpenID Connect client
* metadata, {@code null} if no
* {@code client_id} or client authentication
* was provided.
*
* @return The authorisation.
*
* @throws GeneralException If the grant is invalid, or another
* exception was encountered.
*/
ThirdPartyAssertionAuthorization processThirdPartyGrant(final JOSEObject jwtAssertion,
final Scope scope,
final ClientID clientID,
final boolean confidentialClient,
final OIDCClientMetadata clientMetadata)
throws GeneralException;
}