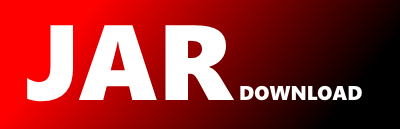
com.nimbusds.openid.connect.provider.spi.grants.TokenSpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of c2id-server-sdk Show documentation
Show all versions of c2id-server-sdk Show documentation
Toolkit for developing Connect2id Server extensions, such as
custom OpenID Connect claims sources and grant handlers.
package com.nimbusds.openid.connect.provider.spi.grants;
import java.util.ArrayList;
import java.util.List;
import com.nimbusds.oauth2.sdk.ParseException;
import com.nimbusds.oauth2.sdk.id.Audience;
import com.nimbusds.oauth2.sdk.id.Subject;
import com.nimbusds.oauth2.sdk.util.JSONObjectUtils;
import net.jcip.annotations.Immutable;
import net.minidev.json.JSONObject;
import org.apache.commons.collections4.CollectionUtils;
/**
* Base token specification.
*/
@Immutable
public class TokenSpec {
/**
* The token lifetime, in seconds. Zero if permanent / not specified.
*/
private final long lifetime;
/**
* Explicit list of audiences for the token, {@code null} if none.
*/
private final List audList;
/**
* The subject in impersonation and delegation cases, {@code null} if
* not applicable.
*/
private final Subject impersonatedSubject;
/**
* Creates a new token specification. No explicit token audience is
* specified. No subject in impersonation and delegation cases is
* specified.
*
* @param lifetime The token lifetime, in seconds. Zero implies
* permanent or not specified (to let the Connect2id
* server apply the default configured lifetime),
* depending on the token type. Must not be a negative
* integer.
*/
public TokenSpec(final long lifetime) {
this(lifetime, null, null);
}
/**
* Creates a new token specification.
*
* @param lifetime The token lifetime, in seconds. Zero
* implies permanent or not specified (to
* let the Connect2id server apply the
* default configured lifetime), depending
* on the token type. Must not be a negative
* integer.
* @param audList Explicit list of audiences for the token,
* {@code null} if not specified.
* @param impersonatedSubject The subject in impersonation and
* delegation cases, {@code null} if not
* applicable.
*/
public TokenSpec(final long lifetime, final List audList, final Subject impersonatedSubject) {
if (lifetime < 0L) {
throw new IllegalArgumentException("The token lifetime must not be negative");
}
this.lifetime = lifetime;
this.audList = audList;
this.impersonatedSubject = impersonatedSubject;
}
/**
* Returns the token lifetime.
*
* @return The token lifetime, in seconds. Zero implies permanent or
* not specified (to let the Connect2id server apply the
* default configured lifetime), depending on the token type.
*/
public long getLifetime() {
return lifetime;
}
/**
* Returns the explicit list of audiences for the token.
*
* @return The explicit list of audiences for the token, {@code null}
* if not specified.
*/
public List getAudience() {
return audList;
}
/**
* Returns the subject in impersonation and delegation cases.
*
* @return The subject in impersonation and delegation cases,
* {@code null} if not applicable.
*/
public Subject getImpersonatedSubject() {
return impersonatedSubject;
}
/**
* Returns a JSON object representation of this token specification.
*
* @return The JSON object.
*/
public JSONObject toJSONObject() {
JSONObject o = new JSONObject();
if (lifetime > 0L) {
o.put("lifetime", lifetime);
}
if (CollectionUtils.isNotEmpty(audList)) {
List sl = new ArrayList<>(audList.size());
for (Audience aud: audList) {
sl.add(aud.getValue());
}
o.put("audience", sl);
}
if (impersonatedSubject != null) {
o.put("impersonated_sub", impersonatedSubject.getValue());
}
return o;
}
@Override
public String toString() {
return toJSONObject().toJSONString();
}
/**
* Parses a token specification from the specified JSON object.
*
* @param jsonObject The JSON object. Must not be {@code null}.
*
* @return The token specification.
*
* @throws ParseException If parsing failed.
*/
public static TokenSpec parse(final JSONObject jsonObject)
throws ParseException {
long lifetime = 0L;
if (jsonObject.containsKey("lifetime")) {
lifetime = JSONObjectUtils.getLong(jsonObject, "lifetime");
}
List audList = null;
if (jsonObject.containsKey("audience")) {
String[] sa = JSONObjectUtils.getStringArray(jsonObject, "audience");
audList = new ArrayList<>(sa.length);
for (String s: sa) {
audList.add(new Audience(s));
}
}
Subject imersonatedSub = null;
if (jsonObject.containsKey("impersonated_sub")) {
try {
imersonatedSub = new Subject(JSONObjectUtils.getString(jsonObject, "impersonated_sub"));
} catch (IllegalArgumentException e) {
// On invalid subject
throw new ParseException(e.getMessage(), e);
}
}
return new TokenSpec(lifetime, audList, imersonatedSub);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy