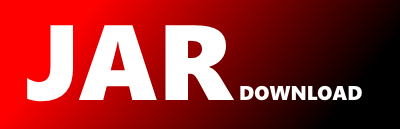
com.nimbusds.common.appendable.JSONArrayWriter Maven / Gradle / Ivy
package com.nimbusds.common.appendable;
import java.io.IOException;
import java.io.Writer;
import java.util.HashSet;
import java.util.Set;
import javax.ws.rs.WebApplicationException;
import net.minidev.json.JSONAware;
/**
* JSON array writer for JAX-RS result streaming.
*/
public class JSONArrayWriter implements Appendable {
/**
* Writer for the appended elements.
*/
private final Writer writer;
/**
* No duplicates switch.
*/
private final boolean noDuplicates;
/**
* Keeps track of the written elements if {@link #noDuplicates} is
* {@code true}.
*/
private final Set writtenItems;
/**
* The position of the appender.
*/
private boolean first;
/**
* Creates a new JSON array writer. The output JSON array may contain
* duplicates.
*
* @param writer Writer for the JSON array. Must not be {@code null}.
*/
public JSONArrayWriter(final Writer writer) {
this(writer, false);
}
/**
* Creates a new JSON array writer.
*
* @param writer Writer for the JSON array. Must not be
* {@code null}.
* @param noDuplicates {@code true} to ensure no duplicates are written
* to the JSON array.
*/
public JSONArrayWriter(final Writer writer,
final boolean noDuplicates) {
if (writer == null) {
throw new IllegalArgumentException("The writer must not be null");
}
this.writer = writer;
this.noDuplicates = noDuplicates;
if (noDuplicates) {
writtenItems = new HashSet<>();
} else {
writtenItems = null;
}
first = true;
}
/**
* Writes out the opening '[' of the JSON array.
*/
public void writeStart() {
try {
writer.write('[');
} catch (IOException e) {
throw new WebApplicationException("Couldn't write JSON array: " + e.getMessage(), e);
}
}
@Override
public void append(final T element) {
if (element == null) {
return; // Nothing to append
}
if (noDuplicates) {
if (writtenItems.contains(element)) {
return; // item already written out
} else {
writtenItems.add(element);
}
}
StringBuilder sb = new StringBuilder();
if (first) {
first = false;
} else {
sb.append(',');
}
sb.append(element.toJSONString());
try {
writer.write(sb.toString());
writer.flush();
} catch (IOException e) {
throw new WebApplicationException("Couldn't write JSON array: " + e.getMessage(), e);
}
}
/**
* Writes out the closing ']' of the JSON array and closes the writer.
*/
public void writeEnd() {
try {
writer.write(']');
writer.close();
} catch (IOException e) {
throw new WebApplicationException("Couldn't write JSON array: " + e.getMessage(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy