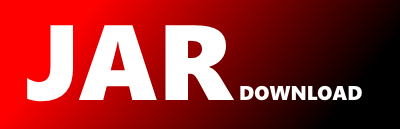
com.nimbusds.common.ldap.LDAPExceptionUtils Maven / Gradle / Ivy
package com.nimbusds.common.ldap;
import com.unboundid.ldap.sdk.LDAPException;
import com.unboundid.ldap.sdk.LDAPResult;
import com.unboundid.ldap.sdk.ResultCode;
import com.thetransactioncompany.jsonrpc2.JSONRPC2Error;
/**
* LDAP exception utilities.
*/
public class LDAPExceptionUtils {
/**
* Turns an LDAP exception into a JSON-RPC 2.0 error with corresponding
* code and message. The LDAP error codes are mapped directly (1:1) to
* JSON-RPC 2.0 error codes, e.g. LDAP error 34 (Invalid DN syntax)
* becomes JSON-RPC 2.0 error 34.
*
* @param ldapException A valid LDAP exception instance. Must not be
* {@code null}.
*
* @return A JSON-RPC 2.0 error representing the original LDAP
* exception, ready for insertion into a JSON-RPC 2.0 response.
*/
public static JSONRPC2Error toJSONRPC2Error(final LDAPException ldapException) {
final ResultCode ldapCode = ldapException.getResultCode();
// The LDAP code is mapped 1:1 to a Json2Ldap error code;
// it has a range from 0 to infinity
final int code = ldapCode.intValue();
String message = ldapCode.getName();
if (message != null && message.length() > 1) {
// Capitalise the first letter of the LDAP message text
message = "LDAP error: " +
message.substring(0,1).toUpperCase() +
message.substring(1);
}
else {
// No LDAP message was found, just state a generic LDAP error
message = "LDAP error";
}
return new JSONRPC2Error(code, message);
}
/**
* Throws a JSON-RPC 2.0 error with corresponding code and message if
* the specified LDAP result indicates failure.
*
* @param result The result from an LDAP request. Must not be
* {@code null}.
*
* @throws JSONRPC2Error On an LDAP result indicating a failure.
*/
public static void ensureSuccess(final LDAPResult result)
throws JSONRPC2Error {
final ResultCode ldapCode = result.getResultCode();
if (ldapCode == ResultCode.SUCCESS)
return;
final int code = ldapCode.intValue();
String message = result.getDiagnosticMessage();
if (message == null)
message = ldapCode.getName(); // try alternative
if (message != null && message.length() > 1) {
// Capitalise the first letter of the LDAP message text
message = "LDAP error: " +
message.substring(0,1).toUpperCase() +
message.substring(1);
}
else {
// No LDAP message was found, just state a generic LDAP error
message = "LDAP error";
}
throw new JSONRPC2Error(code, message);
}
/**
* Prevents instantiation.
*/
private LDAPExceptionUtils() {
// Nothing to do
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy