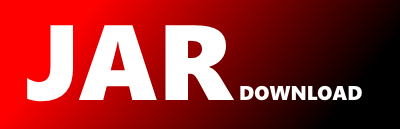
com.nimbusds.common.config.LDAPServerDetails Maven / Gradle / Ivy
Show all versions of common Show documentation
package com.nimbusds.common.config;
import java.util.Properties;
import com.nimbusds.common.ldap.LDAPConnectionSecurity;
import com.thetransactioncompany.util.PropertyParseException;
import com.thetransactioncompany.util.PropertyRetriever;
import com.unboundid.ldap.sdk.LDAPException;
import com.unboundid.ldap.sdk.LDAPURL;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
/**
* LDAP server connect details.
*
* The configuration is stored as public fields which become immutable
* (final) after their initialisation.
*
*
Property keys: [prefix]*
*/
public class LDAPServerDetails implements LoggableConfiguration {
/**
* Specifies an array of one or more LDAP server URLs. If not
* {@code null} the array is guaranteed to contain at least one LDAP
* URL.
*
*
Property key: [prefix]url
*/
public final LDAPURL[] url;
/**
* The preferred algorithm for selecting an LDAP server from the array
* specified by {@link #url}, {@code null} if only a single server URL
* is defined.
*
*
Property key: [prefix]selectionAlgorithm
*/
public final ServerSelectionAlgorithm selectionAlgorithm;
/**
* The default server selection algorithm.
*/
public static final ServerSelectionAlgorithm DEFAULT_SELECTION_ALGORITHM =
ServerSelectionAlgorithm.FAILOVER;
/**
* The LDAP connection security.
*
*
Property key: [prefix]security
*/
public final LDAPConnectionSecurity security;
/**
* The default LDAP connection security.
*/
public static final LDAPConnectionSecurity DEFAULT_SECURITY =
LDAPConnectionSecurity.STARTTLS;
/**
* The timeout in milliseconds for LDAP connect requests. If zero the
* underlying LDAP client library will determine this value.
*
*
Property key: [prefix]connectTimeout
*/
public final int connectTimeout;
/**
* The default timeout in milliseconds for LDAP connect requests.
*/
public static final int DEFAULT_CONNECT_TIMEOUT = 0;
/**
* The timeout in milliseconds for LDAP server responses. If zero the
* underlying LDAP client library will determine this value.
*
*
Property key: [prefix]responseTimeout
*/
public final int responseTimeout;
/**
* The default timeout in milliseconds for LDAP server responses.
*/
public static final int DEFAULT_RESPONSE_TIMEOUT = 0;
/**
* Determines whether to accept self-signed certificates presented by
* the LDAP server (for secure SSL or StartTLS connections).
*
*
Property key: [prefix]trustSelfSignedCerts
*/
public final boolean trustSelfSignedCerts;
/**
* The default trust for self-signed certificates.
*/
public static final boolean DEFAULT_SELF_SIGNED_CERTS_TRUST = false;
/**
* Creates a new LDAP server details instance.
*
* @param url The LDAP server URL. Must not be
* {@code null}.
* @param security The LDAP connection security. Must not
* be {@code null}.
* @param connectTimeout The LDAP server connect timeout, in
* milliseconds. If zero the underlying
* LDAP client library will determine this
* value.
* @param responseTimeout The LDAP server response timeout, in
* milliseconds. If zero the underlying
* LDAP client library will determine this
* value.
* @param trustSelfSignedCerts Determines whether to accept self-signed
* certificates presented by the LDAP
* server (for secure SSL or StartTLS
* connections).
*/
public LDAPServerDetails(final LDAPURL url,
final LDAPConnectionSecurity security,
final int connectTimeout,
final int responseTimeout,
final boolean trustSelfSignedCerts) {
this(new LDAPURL[]{ url }, null, security, connectTimeout, responseTimeout, trustSelfSignedCerts);
}
/**
* Creates a new LDAP server details instance.
*
* @param url An array of one or more LDAP server
* URLs. It must contain at least one LDAP
* URL and not be {@code null}.
* @param selectionAlgorithm The preferred algorithm for selecting an
* LDAP server from the URL array. May be
* {@code null} if only a single LDAP
* server URL is defined.
* @param security The LDAP connection security. Must not
* be {@code null}.
* @param connectTimeout The LDAP server connect timeout, in
* milliseconds. If zero the underlying
* LDAP client library will determine this
* value.
* @param responseTimeout The LDAP server response timeout, in
* milliseconds. If zero the underlying
* LDAP client library will determine this
* value.
* @param trustSelfSignedCerts Determines whether to accept self-signed
* certificates presented by the LDAP
* server (for secure SSL or StartTLS
* connections).
*/
public LDAPServerDetails(final LDAPURL[] url,
final ServerSelectionAlgorithm selectionAlgorithm,
final LDAPConnectionSecurity security,
final int connectTimeout,
final int responseTimeout,
final boolean trustSelfSignedCerts) {
if (url == null)
throw new IllegalArgumentException("The LDAP server URL must not be null");
if (url.length == 0)
throw new IllegalArgumentException("The LDAP server URL array must contain at least one entry");
this.url = url;
if (url.length > 1 && selectionAlgorithm == null)
throw new IllegalArgumentException("An LDAP server selection algorithm must be specified");
this.selectionAlgorithm = selectionAlgorithm;
if (security == null)
throw new IllegalArgumentException("The LDAP connection security must not be null");
this.security = security;
this.connectTimeout = connectTimeout;
this.responseTimeout = responseTimeout;
this.trustSelfSignedCerts = trustSelfSignedCerts;
}
/**
* Creates a new LDAP server details instance from the specified
* properties.
*
*
Mandatory properties:
*
*
* - [prefix]url
*
*
* Conditionally mandatory properties:
*
*
* - [prefix]selectionAlgorithm - if more than one LDAP server
* URL is specified.
*
*
* Optional properties, with defaults:
*
*
* - [prefix]security = STARTTLS
*
- [prefix]connectTimeout = 0
*
- [prefix]responseTimeout = 0
*
- [prefix]trustSelfSignedCerts = false
*
*
* @param prefix The properties prefix. Must not be {@code null}.
* @param props The properties. Must not be {@code null}.
*
* @throws PropertyParseException On a missing or invalid property.
*/
public LDAPServerDetails(final String prefix, final Properties props)
throws PropertyParseException {
this(prefix, props, true);
}
/**
* Creates a new LDAP server details instance from the specified
* properties.
*
* Mandatory properties:
*
*
* - none
*
*
* Conditionally mandatory properties:
*
*
* - [prefix]url
*
- [prefix]selectionAlgorithm - if more than one LDAP server
* URL is specified.
*
*
* Optional properties, with defaults:
*
*
* - [prefix]security = STARTTLS
*
- [prefix]connectTimeout = 0
*
- [prefix]responseTimeout = 0
*
- [prefix]trustSelfSignedCerts = false
*
*
* @param prefix The properties prefix. Must not be {@code null}.
* @param props The properties. Must not be {@code null}.
*
* @throws PropertyParseException On a missing or invalid property.
*/
public LDAPServerDetails(final String prefix,
final Properties props,
final boolean requireURL)
throws PropertyParseException {
var pr = new PropertyRetriever(props);
String urlsString = pr.getOptString(prefix + "url", null);
if (urlsString != null && ! urlsString.trim().isEmpty()) {
String[] tokens = urlsString.split("\\s+");
url = new LDAPURL[tokens.length];
for (int i=0; i < tokens.length; i++) {
try {
url[i] = new LDAPURL(tokens[i]);
} catch (LDAPException e) {
throw new PropertyParseException("Invalid LDAP URL",
prefix + "url",
tokens[i]);
}
if (url[i].getHost() == null)
throw new PropertyParseException("Missing host in LDAP URL",
prefix + "url",
tokens[i]);
}
} else if (requireURL) {
throw new PropertyParseException("Missing LDAP URL", prefix + "url");
} else {
url = null;
}
// Selection algorithm only required for multiple server URLs
if (url != null && url.length > 1) {
selectionAlgorithm = pr.getOptEnum(prefix + "selectionAlgorithm",
ServerSelectionAlgorithm.class,
DEFAULT_SELECTION_ALGORITHM);
} else {
selectionAlgorithm = null;
}
security = pr.getOptEnum(prefix + "security",
LDAPConnectionSecurity.class,
DEFAULT_SECURITY);
connectTimeout = pr.getOptInt(prefix + "connectTimeout",
DEFAULT_CONNECT_TIMEOUT);
responseTimeout = pr.getOptInt(prefix + "responseTimeout",
DEFAULT_RESPONSE_TIMEOUT);
trustSelfSignedCerts = pr.getOptBoolean(prefix + "trustSelfSignedCerts",
DEFAULT_SELF_SIGNED_CERTS_TRUST);
}
/**
* Logs the configuration details at INFO level.
*/
@Override
public void log() {
Logger log = LogManager.getLogger(LOG_CATEGORY);
if (url == null) {
log.info("[CM1000] LDAP server URL: not specified");
return;
}
for (int i=0; i < url.length; i++) {
log.info("[CM1001] LDAP server [{}]: {}:{} (transport security {})", i, url[i].getHost(), url[i].getPort(), security);
}
if (security == LDAPConnectionSecurity.NONE)
log.warn("[CM1002] LDAP server connection not protected (security=NONE), consider using STARTTLS or SSL");
if (url.length > 1)
log.info("[CM1003] LDAP server selection algorithm: {}", selectionAlgorithm);
if (connectTimeout > 0)
log.info("[CM1004] LDAP server connect timeout: {} ms", connectTimeout);
else
log.info("[CM1004] LDAP server connect timeout: Determined by LDAP client library");
if (responseTimeout > 0)
log.info("[CM1005] LDAP server response timeout: {} ms", responseTimeout);
else
log.info("[CM1005] LDAP server response timeout: Determined by LDAP client library");
log.info("[CM1006] Self-signed LDAP server certificates are trusted: {}", trustSelfSignedCerts);
}
}