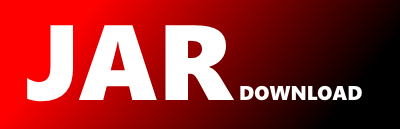
com.nimbusds.common.id.AuthzIdResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
Common Connect2id software classes
package com.nimbusds.common.id;
import com.unboundid.ldap.sdk.*;
import java.text.ParseException;
/**
* Resolves the authorisation identity (authzId) associated with a bind (auth)
* request.
*/
public class AuthzIdResolver {
/**
* Resolves the authzId (user) of a simple bind request.
*
* @param bindRequest The bind request, must not be {@code null}.
*
* @return The authzId, {@code null} if it couldn't be resolved.
*/
public static AuthzId resolve(final SimpleBindRequest bindRequest) {
if (bindRequest == null)
throw new IllegalArgumentException("The bind request must not be null");
DN user;
try {
user = new DN(bindRequest.getBindDN());
} catch (LDAPException e ) {
return null;
}
return new AuthzId(user);
}
/**
* Resolves the authzId (user) of a plain SASL bind request. If a
* target user is specified, the authorisation identity is returned,
* else the authentication identity.
*
* @param bindRequest The bind request, must not be {@code null}.
*
* @return The authzId, {@code null} if it couldn't be resolved.
*/
public static AuthzId resolve(final PLAINBindRequest bindRequest) {
if (bindRequest == null)
throw new IllegalArgumentException("The bind request must not be null");
String user;
if (bindRequest.getAuthorizationID() != null)
user = bindRequest.getAuthorizationID();
else
user = bindRequest.getAuthenticationID();
try {
return AuthzId.parse(user);
} catch (ParseException e) {
return null;
}
}
/**
* Resolves the authzId (user) of a bind request.
*
* @param bindRequest The bind request, must not be {@code null}.
*
* @return The authzId, {@code null} if it couldn't be resolved or the
* bind request type is not supported.
*/
public static AuthzId resolve(final BindRequest bindRequest) {
if (bindRequest == null)
throw new IllegalArgumentException("The bind request must not be null");
if (bindRequest instanceof SimpleBindRequest)
return resolve((SimpleBindRequest)bindRequest);
else if (bindRequest instanceof PLAINBindRequest)
return resolve((PLAINBindRequest)bindRequest);
else
return null;
}
/**
* Public instantiation disabled.
*/
private AuthzIdResolver() {
// empty
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy