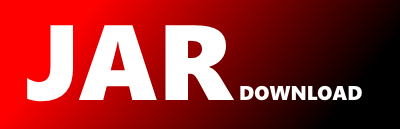
com.nimbusds.common.ldap.LDAPConnectionPoolFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
Common Connect2id software classes
package com.nimbusds.common.ldap;
import java.security.GeneralSecurityException;
import java.security.KeyStoreException;
import javax.net.SocketFactory;
import javax.net.ssl.SSLContext;
import com.nimbusds.common.config.CustomKeyStoreConfiguration;
import com.nimbusds.common.config.CustomTrustStoreConfiguration;
import com.nimbusds.common.config.DirectoryUser;
import com.nimbusds.common.config.LDAPServerConnectionPoolDetails;
import com.unboundid.ldap.sdk.*;
import com.unboundid.util.ssl.SSLUtil;
/**
* Factory for establishing LDAP connection pools to a directory server.
*/
public class LDAPConnectionPoolFactory {
/**
* The LDAP server connect details.
*/
private final LDAPServerConnectionPoolDetails ldapServer;
/**
* The custom trust store for LDAP over SSL/TLS.
*/
private final CustomTrustStoreConfiguration customTrustStore;
/**
* The custom key store for LDAP over SSL/TLS.
*/
private final CustomKeyStoreConfiguration customKeyStore;
/**
* The directory user to bind to for each connection, {@code null} if
* not specified (for unauthenticated connections).
*/
private final DirectoryUser dirUser;
/**
* Creates a LDAP connection pool factory.
*
* @param ldapServer The LDAP server connect details. Must
* specify a URL and must not be {@code null}.
* @param customTrustStore The custom trust store configuration. Must
* not be {@code null}.
* @param customKeyStore The custom key store configuration. Must not
* be {@code null}.
* @param dirUser The directory user to bind to for each
* LDAP connection. If {@code null} connections
* will be unauthenticated.
*/
public LDAPConnectionPoolFactory(final LDAPServerConnectionPoolDetails ldapServer,
final CustomTrustStoreConfiguration customTrustStore,
final CustomKeyStoreConfiguration customKeyStore,
final DirectoryUser dirUser) {
if (ldapServer == null || ldapServer.url.length == 0)
throw new IllegalArgumentException("The LDAP server details must not be null");
this.ldapServer = ldapServer;
if (customTrustStore == null)
throw new IllegalArgumentException("The custom trust store must not be null");
this.customTrustStore = customTrustStore;
if (customKeyStore == null)
throw new IllegalArgumentException("The custom key store must not be null");
this.customKeyStore = customKeyStore;
this.dirUser = dirUser;
}
/**
* Creates a new LDAP connection pool. No initial connections are
* established, to prevent connect exceptions if the backend is
* offline at the time the pool is created.
*
* @return The LDAP connection pool.
*
* @throws KeyStoreException If the key store could not be
* unlocked (for SSL/StartTLS
* connections).
* @throws GeneralSecurityException On a general security exception
* (for SSL/StartTLS connections).
* @throws LDAPException If an LDAP exception is
* encountered (during creation of the
* initial pooled LDAP connections).
*/
public LDAPConnectionPool createLDAPConnectionPool()
throws GeneralSecurityException, LDAPException {
SocketFactory socketFactory = null;
PostConnectProcessor postProc = null;
if (ldapServer.security.equals(LDAPConnectionSecurity.SSL)) {
SSLUtil sslUtil = LDAPConnectionFactory.initSecureConnectionContext(
customTrustStore,
customKeyStore,
ldapServer.trustSelfSignedCerts);
socketFactory = sslUtil.createSSLSocketFactory();
} else if (ldapServer.security.equals(LDAPConnectionSecurity.STARTTLS)) {
SSLUtil sslUtil = LDAPConnectionFactory.initSecureConnectionContext(
customTrustStore,
customKeyStore,
ldapServer.trustSelfSignedCerts);
SSLContext sslContext = sslUtil.createSSLContext();
postProc = new StartTLSPostConnectProcessor(sslContext);
}
LDAPConnectionOptions opts = new LDAPConnectionOptions();
opts.setConnectTimeoutMillis(ldapServer.connectTimeout);
opts.setResponseTimeoutMillis(ldapServer.responseTimeout);
ServerSet serverSet = LDAPServerSetFactory.create(
ldapServer.url,
ldapServer.selectionAlgorithm,
socketFactory,
opts);
// Prepare bind request?
BindRequest bindRequest = null;
if (dirUser != null) {
bindRequest = new SimpleBindRequest(dirUser.dn, dirUser.password);
}
LDAPConnectionPool pool = new LDAPConnectionPool(serverSet,
bindRequest,
ldapServer.connectionPoolInitialSize,
ldapServer.connectionPoolSize,
postProc);
// Additional configuration
pool.setCreateIfNecessary(true);
pool.setMaxWaitTimeMillis(ldapServer.connectionPoolMaxWaitTime);
pool.setMaxConnectionAgeMillis(ldapServer.connectionMaxAge);
return pool;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy