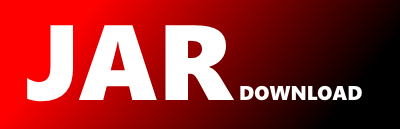
com.nimbusds.infinispan.persistence.dynamodb.DynamoDBItemTransformer Maven / Gradle / Ivy
Show all versions of infinispan-cachestore-dynamodb Show documentation
package com.nimbusds.infinispan.persistence.dynamodb;
import com.amazonaws.services.dynamodbv2.document.Item;
import com.nimbusds.infinispan.persistence.common.InfinispanEntry;
import com.nimbusds.infinispan.persistence.dynamodb.config.DynamoDBStoreConfiguration;
/**
* Interface for transforming between Infinispan entries (key / value pair and
* metadata) and a corresponding DynamoDB item. Implementations must be
* thread-safe.
*
* To specify an entry transformer for a given Infinispan cache that is
* backed by a DynamoDB store, provide its class name to the
* {@link DynamoDBStoreConfiguration store configuration}.
*/
public interface DynamoDBItemTransformer {
/**
* Returns the base name of the DynamoDB table. Required to create and
* to connect to the DynamoDB table for storing the cache entries. The
* final DynamoDB table name is formed by prefixing the optional
* configuration {@code table-prefix} to the base name.
*
* @return The table name.
*/
String getTableName();
/**
* Returns the DynamoDB hash key attribute name. Required to create the
* DynamoDB table for storing the cache entries.
*
* @return The hash key attribute name.
*/
String getHashKeyAttributeName();
/**
* Resolves the DynamoDB hash key (of scalar attribute type string) for
* the specified Infinispan entry key.
*
* @param key The Infinispan entry key. Not {@code null}.
*
* @return The DynamoDB hash key.
*/
String resolveHashKey(final K key);
/**
* Transforms the specified Infinispan entry (key / value pair with
* optional metadata) to a DynamoDB item.
*
* Example:
*
*
Infinispan entry:
*
*
* - Key: cae7t
*
- Value: Java POJO with fields {@code uid=cae7t},
* {@code givenName=Alice}, {@code surname=Adams} and
* {@code [email protected]}.
*
- Metadata: Specifies the entry expiration and other
* properties.
*
*
* Resulting DynamoDB item:
*
*
* uid: cae7t (key)
* surname: Adams
* given_name: Alice
* email: [email protected]
*
*
* @param infinispanEntry The Infinispan entry. Not {@code null}.
*
* @return The DynamoDB item.
*/
Item toItem(final InfinispanEntry infinispanEntry);
/**
* Transforms the specified DynamoDB item to an Infinispan entry (key /
* value / metadata triple).
*
* Example:
*
*
DynamoDB item:
*
*
* uid: cae7t
* surname: Adams
* given_name: Alice
* email: [email protected]
*
*
* Resulting Infinispan entry:
*
*
* - Key: cae7t
*
- Value: Java POJO with fields {@code uid=cae7t},
* {@code givenName=Alice}, {@code surname=Adams} and
* {@code [email protected]}.
*
- Metadata: Default metadata (no expiration, etc).
*
*
* @param item The DynamoDB item. Must not be {@code null}.
*
* @return The Infinispan entry (key / value pair with optional
* metadata).
*/
InfinispanEntry toInfinispanEntry(final Item item);
}