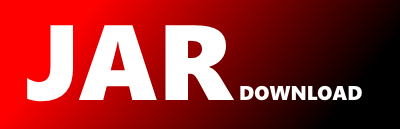
com.nimbusds.infinispan.persistence.dynamodb.config.DynamoDBStoreConfiguration Maven / Gradle / Ivy
package com.nimbusds.infinispan.persistence.dynamodb.config;
import java.util.Properties;
import java.util.Set;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.dynamodbv2.model.ProvisionedThroughput;
import com.nimbusds.common.config.LoggableConfiguration;
import com.nimbusds.infinispan.persistence.dynamodb.DynamoDBItemTransformer;
import com.nimbusds.infinispan.persistence.dynamodb.DynamoDBStore;
import com.nimbusds.infinispan.persistence.dynamodb.logging.Loggers;
import net.jcip.annotations.Immutable;
import org.infinispan.commons.configuration.BuiltBy;
import org.infinispan.commons.configuration.ConfigurationFor;
import org.infinispan.commons.configuration.attributes.Attribute;
import org.infinispan.commons.configuration.attributes.AttributeDefinition;
import org.infinispan.commons.configuration.attributes.AttributeSet;
import org.infinispan.commons.util.StringPropertyReplacer;
import org.infinispan.configuration.cache.AbstractStoreConfiguration;
import org.infinispan.configuration.cache.AsyncStoreConfiguration;
import org.infinispan.configuration.cache.SingletonStoreConfiguration;
/**
* DynamoDB store configuration.
*/
@Immutable
@BuiltBy(DynamoDBStoreConfigurationBuilder.class)
@ConfigurationFor(DynamoDBStore.class)
public class DynamoDBStoreConfiguration extends AbstractStoreConfiguration implements LoggableConfiguration {
/**
* The attribute definition for the DynamoDB endpoint.
*/
static final AttributeDefinition ENDPOINT = AttributeDefinition.builder("endpoint", null, String.class).build();
/**
* The attribute definition for the AWS region.
*/
static final AttributeDefinition REGION = AttributeDefinition.builder("region", Regions.DEFAULT_REGION, Regions.class).build();
/**
* The attribute definition for the item transformer class.
*/
static final AttributeDefinition ITEM_TRANSFORMER = AttributeDefinition.builder("recordTransformer", null, Class.class).build();
/**
* The attribute definition for the query executor class.
*/
static final AttributeDefinition QUERY_EXECUTOR = AttributeDefinition.builder("queryExecutor", null, Class.class).build();
/**
* The attribute definition for the indexed DynamoDB table attributes.
*/
static final AttributeDefinition INDEXED_ATTRIBUTES = AttributeDefinition.builder("indexedAttributes", null, Set.class).build();
/**
* The attribute definition for the read capacity to provision when
* creating a new DynamoDB table and indices.
*/
static final AttributeDefinition READ_CAPACITY = AttributeDefinition.builder("readCapacity", 1L, Long.class).build();
/**
* The attribute definition for the write capacity to provision when
* creating a new DynamoDB table and indices.
*/
static final AttributeDefinition WRITE_CAPACITY = AttributeDefinition.builder("writeCapacity", 1L, Long.class).build();
/**
* The attribute definition for the optional DynamoDB table prefix.
*/
static final AttributeDefinition TABLE_PREFIX = AttributeDefinition.builder("tablePrefix", "", String.class).build();
/**
* The attribute definition for the optional range key to apply to all
* DynamoDB operations.
*/
static final AttributeDefinition APPLY_RANGE_KEY = AttributeDefinition.builder("applyRangeKey", null, String.class).build();
/**
* The attribute definition for the value of the optional range key.
*/
static final AttributeDefinition RANGE_KEY_VALUE = AttributeDefinition.builder("rangeKeyValue", null, String.class).build();
/**
* Returns the attribute definitions for the DynamoDB store configuration.
*
* @return The attribute definitions.
*/
public static AttributeSet attributeDefinitionSet() {
return new AttributeSet(DynamoDBStoreConfiguration.class,
AbstractStoreConfiguration.attributeDefinitionSet(),
ENDPOINT,
REGION,
ITEM_TRANSFORMER,
QUERY_EXECUTOR,
INDEXED_ATTRIBUTES,
READ_CAPACITY,
WRITE_CAPACITY,
TABLE_PREFIX,
APPLY_RANGE_KEY,
RANGE_KEY_VALUE);
}
/**
* The DynamoDB endpoint. If set region is overridden (ignored).
*/
private final Attribute endpoint;
/**
* The AWS region.
*/
private final Attribute region;
/**
* The class for transforming between Infinispan entries (key / value
* pair and optional metadata) and a corresponding DynamoDB item.
*
* See {@link DynamoDBItemTransformer}.
*/
private final Attribute itemTransformerClass;
/**
* Optional class for executing direct DynamoDB queries against
* DynamoDB.
*
* See {@link com.nimbusds.infinispan.persistence.common.query.QueryExecutor}
*/
private final Attribute queryExecutorClass;
/**
* Optional set of the indexed DynamoDB attributes.
*/
private final Attribute indexedAttributes;
/**
* The optional DynamoDB table prefix.
*/
private final Attribute tablePrefix;
/**
* The read capacity to provision when creating a new DynamoDB table
* and indices.
*/
private final Attribute readCapacity;
/**
* The write capacity to provision when creating a new DynamoDB table
* and indices.
*/
private final Attribute writeCapacity;
/**
* The optional range key to apply to all DynamoDB operations.
*/
private final Attribute applyRangeKey;
/**
* The value of the optional range key.
*/
private final Attribute rangeKeyValue;
/**
* Creates a new DynamoDB store configuration.
*
* @param attributes The configuration attributes. Must not
* be {@code null}.
* @param asyncConfig Configuration for the async cache
* loader.
* @param singletonStoreConfig Configuration for a singleton store.
*/
public DynamoDBStoreConfiguration(final AttributeSet attributes,
final AsyncStoreConfiguration asyncConfig,
final SingletonStoreConfiguration singletonStoreConfig) {
super(attributes, asyncConfig, singletonStoreConfig);
endpoint = attributes.attribute(ENDPOINT);
assert endpoint != null;
region = attributes.attribute(REGION);
assert region != null;
itemTransformerClass = attributes.attribute(ITEM_TRANSFORMER);
assert itemTransformerClass != null;
queryExecutorClass = attributes.attribute(QUERY_EXECUTOR);
indexedAttributes = attributes.attribute(INDEXED_ATTRIBUTES);
readCapacity = attributes.attribute(READ_CAPACITY);
assert readCapacity != null;
writeCapacity = attributes.attribute(WRITE_CAPACITY);
assert writeCapacity != null;
tablePrefix = attributes.attribute(TABLE_PREFIX);
assert tablePrefix != null;
applyRangeKey = attributes.attribute(APPLY_RANGE_KEY);
assert applyRangeKey != null;
rangeKeyValue = attributes.attribute(RANGE_KEY_VALUE);
assert rangeKeyValue != null;
}
/**
* Returns the DynamoDB endpoint.
*
* @return The DynamoDB endpoint, {@code null} if not set.
*/
public String getEndpoint() {
return endpoint.get();
}
/**
* Returns the AWS region.
*
* @return The AWS region.
*/
public Regions getRegion() {
return region.get();
}
/**
* Returns the class for transforming between Infinispan entries (key /
* value pairs and optional metadata) and a corresponding DynamoDB
* item.
*
* See {@link DynamoDBItemTransformer}.
*
* @return The item transformer class.
*/
public Class getItemTransformerClass() {
return itemTransformerClass.get();
}
/**
* Returns the optional class for executing direct queries against
* DynamoDB.
*
*
See {@link com.nimbusds.infinispan.persistence.common.query.QueryExecutor}
*
* @return The query executor class, {@code null} if not specified.
*/
public Class getQueryExecutorClass() {
return queryExecutorClass.get();
}
/**
* Returns the optional indexed DynamoDB attributes.
*
*
See {@link #getQueryExecutorClass}
*
* @return The indexed attributes, {@code null} if no specified.
*/
@SuppressWarnings("unchecked")
public Set getIndexedAttributes() {
return (Set) indexedAttributes.get();
}
/**
* Returns the read and write capacity to provision when creating a new
* DynamoDB table.
*
* @return The read and write capacity.
*/
public ProvisionedThroughput getProvisionedThroughput() {
return new ProvisionedThroughput(readCapacity.get(), writeCapacity.get());
}
/**
* Returns the optional DynamoDB table prefix.
*
* @return The DynamoDB table prefix, empty string if not set.
*/
public String getTablePrefix() {
return tablePrefix.get();
}
/**
* Returns the name of the optional range key to apply to all DynamoDB
* operations.
*
* @return The range key name, {@code null} if not specified.
*/
public String getApplyRangeKey() {
return applyRangeKey.get();
}
/**
* Returns the value of the optional range key.
*
* @return The range key value, {@code null} if not specified.
*/
public String getRangeKeyValue() {
return rangeKeyValue.get();
}
@Override
public Properties properties() {
// Interpolate with system properties where ${sysPropName} is found
Properties interpolatedProps = new Properties();
for (String name: super.properties().stringPropertyNames()) {
interpolatedProps.setProperty(name, StringPropertyReplacer.replaceProperties(super.properties().getProperty(name)));
}
return interpolatedProps;
}
@Override
public void log() {
Loggers.MAIN_LOG.info("[DS0000] Infinispan DynamoDB store: Endpoint (overrides region): {}", getEndpoint() != null ? getEndpoint() : "not set");
Loggers.MAIN_LOG.info("[DS0001] Infinispan DynamoDB store: Region: {}", getRegion());
Loggers.MAIN_LOG.info("[DS0002] Infinispan DynamoDB store: Item transformer class: {}", getItemTransformerClass().getCanonicalName());
Loggers.MAIN_LOG.info("[DS0009] Infinispan DynamoDB store: Query executor class: {}", getQueryExecutorClass() != null ? getQueryExecutorClass().getCanonicalName() : "none");
Loggers.MAIN_LOG.info("[DS0010] Infinispan DynamoDB store: Indexed attributes: {}", getIndexedAttributes() != null ? getIndexedAttributes() : "none");
Loggers.MAIN_LOG.info("[DS0004] Infinispan DynamoDB store: AWS secret access key: [hidden]");
Loggers.MAIN_LOG.info("[DS0005] Infinispan DynamoDB store: Capacity to provision: read={} write={}", getProvisionedThroughput().getReadCapacityUnits(), getProvisionedThroughput().getWriteCapacityUnits());
Loggers.MAIN_LOG.info("[DS0006] Infinispan DynamoDB store: Table prefix: {}", getTablePrefix().isEmpty() ? "none" : getTablePrefix());
Loggers.MAIN_LOG.info("[DS0007] Infinispan DynamoDB store: Apply range key: {}", getApplyRangeKey() != null ? getApplyRangeKey() : "none");
Loggers.MAIN_LOG.info("[DS0008] Infinispan DynamoDB store: Range key value: {}", getRangeKeyValue() != null ? getRangeKeyValue() : "none");
}
}