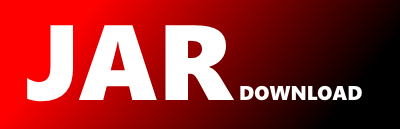
com.nimbusds.infinispan.persistence.dynamodb.config.DynamoDBStoreConfigurationChildBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-cachestore-dynamodb Show documentation
Show all versions of infinispan-cachestore-dynamodb Show documentation
Infinispan module for persisting data to an AWS DynamoDB table
package com.nimbusds.infinispan.persistence.dynamodb.config;
import java.util.Set;
import com.amazonaws.regions.Regions;
import org.infinispan.configuration.cache.StoreConfigurationChildBuilder;
/**
* DynamoDB store configuration child builder.
*/
public interface DynamoDBStoreConfigurationChildBuilder extends StoreConfigurationChildBuilder {
/**
* Sets the DynamoDB endpoint.
*
* @param endpoint The endpoint, {@code null} if not specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder endpoint(final String endpoint);
/**
* Sets the DynamoDB region.
*
* @param region The region, {@code null} if not specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder region(final Regions region);
/**
* Sets the class for transforming between Infinispan entries (key /
* value pair and optional metadata) and a corresponding DynamoDB item.
*
* @param itemTransformerClass The item transformer class. Must not
* be {@code null}.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder itemTransformerClass(final Class itemTransformerClass);
/**
* Sets the optional class for executing direct queries against
* DynamoDB. If set {@link #indexedAttributes} must also be specified.
*
* @param queryExecutorClass The query executor class, {@code null} if
* not required.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder queryExecutorClass(final Class queryExecutorClass);
/**
* Sets the optional indexed DynamoDB table attributes. If set
* {@link #queryExecutorClass} must also be specified.
*
* @param indexAttributes The indexed attributes, {@code null} if not
* required.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder indexedAttributes(final Set indexAttributes);
/**
* Sets the read capacity to provision when creating a new DynamoDB
* table.
*
* @param readCapacity The read capacity. Must be equal or larger than
* one.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder readCapacity(final long readCapacity);
/**
* Sets the write capacity to provision when creating a new DynamoDB
* table.
*
* @param writeCapacity The write capacity. Must be equal or larger
* than one.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder writeCapacity(final long writeCapacity);
/**
* Sets the DynamoDB table prefix.
*
* @param tablePrefix The table prefix, {@code null} if not specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder tablePrefix(final String tablePrefix);
/**
* Sets the name of the optional range key to apply to all DynamoDB
* operations.
*
* @param rangeKeyName The range key name, {@code null} if not
* specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder applyRangeKey(final String rangeKeyName);
/**
* Sets the value of the optional range key.
*
* @param rangeKeyValue The range key value, {@code null} if not
* specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder rangeKeyValue(final String rangeKeyValue);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy