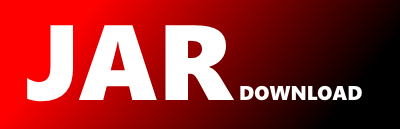
com.nimbusds.infinispan.persistence.dynamodb.config.DynamoDBStoreConfigurationParser Maven / Gradle / Ivy
package com.nimbusds.infinispan.persistence.dynamodb.config;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
import javax.xml.stream.XMLStreamConstants;
import javax.xml.stream.XMLStreamException;
import static org.infinispan.commons.util.StringPropertyReplacer.replaceProperties;
import com.amazonaws.regions.Regions;
import org.infinispan.commons.CacheConfigurationException;
import org.infinispan.configuration.cache.ConfigurationBuilder;
import org.infinispan.configuration.cache.PersistenceConfigurationBuilder;
import org.infinispan.configuration.parsing.*;
import org.kohsuke.MetaInfServices;
/**
* XML configuration parser for XML schema v1.0.
*/
@MetaInfServices
@Namespaces({
@Namespace(uri = "urn:infinispan:config:store:dynamodb:1.0", root = "dynamodb-store"),
@Namespace(root = "dynamodb-store")
})
public class DynamoDBStoreConfigurationParser implements ConfigurationParser {
@Override
public void readElement(final XMLExtendedStreamReader reader, final ConfigurationBuilderHolder holder)
throws XMLStreamException {
ConfigurationBuilder builder = holder.getCurrentConfigurationBuilder();
Element element = Element.forName(reader.getLocalName());
switch (element) {
case DYNAMODB_STORE: {
this.parseDynamoDBStore(reader, builder.persistence(), holder.getClassLoader());
break;
}
default: {
throw ParseUtils.unexpectedElement(reader);
}
}
}
/**
* Parses a DynamoDB store XML element.
*/
private void parseDynamoDBStore(final XMLExtendedStreamReader reader,
final PersistenceConfigurationBuilder persistenceBuilder,
final ClassLoader classLoader)
throws XMLStreamException {
DynamoDBStoreConfigurationBuilder builder = new DynamoDBStoreConfigurationBuilder(persistenceBuilder);
this.parseDynamoDBStoreAttributes(reader, builder, classLoader);
while (reader.hasNext() && (reader.nextTag() != XMLStreamConstants.END_ELEMENT)) {
Element element = Element.forName(reader.getLocalName());
switch (element) {
default: {
Parser.parseStoreElement(reader, builder);
break;
}
}
}
persistenceBuilder.addStore(builder);
}
/**
* Parses the attributes of an XML store element.
*/
private void parseDynamoDBStoreAttributes(final XMLExtendedStreamReader reader,
final DynamoDBStoreConfigurationBuilder builder,
final ClassLoader classLoader)
throws XMLStreamException {
for (int i = 0; i < reader.getAttributeCount(); i++) {
ParseUtils.requireNoNamespaceAttribute(reader, i);
String value = replaceProperties(reader.getAttributeValue(i));
Attribute attribute = Attribute.forName(reader.getAttributeLocalName(i));
switch (attribute) {
case ENDPOINT:
builder.endpoint(value);
break;
case REGION:
try {
builder.region(Regions.fromName(value));
} catch (Exception e) {
throw new CacheConfigurationException(e);
}
break;
case ITEM_TRANSFORMER:
try {
builder.itemTransformerClass(classLoader.loadClass(value));
} catch (ClassNotFoundException e) {
throw new CacheConfigurationException(e);
}
break;
case QUERY_EXECUTOR:
try {
builder.queryExecutorClass(classLoader.loadClass(value));
} catch (ClassNotFoundException e) {
throw new CacheConfigurationException(e);
}
case INDEXED_ATTRIBUTES:
builder.indexedAttributes(parseStringSet(value));
break;
case READ_CAPACITY:
try {
builder.readCapacity(Long.valueOf(value));
} catch (NumberFormatException e) {
throw new CacheConfigurationException(e);
}
break;
case WRITE_CAPACITY:
try {
builder.writeCapacity(Long.valueOf(value));
} catch (NumberFormatException e) {
throw new CacheConfigurationException(e);
}
break;
case TABLE_PREFIX:
builder.tablePrefix(value);
break;
case APPLY_RANGE_KEY:
builder.applyRangeKey(value);
break;
case RANGE_KEY_VALUE:
builder.rangeKeyValue(value);
break;
default: {
Parser.parseStoreAttribute(reader, i, builder);
break;
}
}
}
}
@Override
public Namespace[] getNamespaces() {
return ParseUtils.getNamespaceAnnotations(getClass());
}
/**
* Splits a string of comma and / or white space separated strings
* into a string set.
*
* @param s The string to split, {@code null} if not specified.
*
* @return The string set, {@code null} if not specified.
*/
static Set parseStringSet(final String s) {
if (null == s || s.trim().isEmpty()) {
return null;
}
return new HashSet<>(Arrays.asList(s.replaceAll("^[,\\s]+", "").split("[,\\s]+")));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy