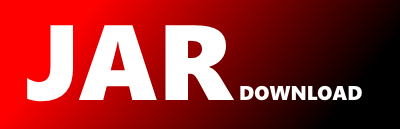
com.nimbusds.infinispan.persistence.dynamodb.MetadataUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-cachestore-dynamodb Show documentation
Show all versions of infinispan-cachestore-dynamodb Show documentation
Infinispan module for persisting data to an AWS DynamoDB table
The newest version!
package com.nimbusds.infinispan.persistence.dynamodb;
import com.amazonaws.services.dynamodbv2.document.Item;
import com.nimbusds.infinispan.persistence.common.InternalMetadataBuilder;
import org.infinispan.metadata.InternalMetadata;
/**
* Infinispan metadata persistence utilities.
*/
public class MetadataUtils {
/**
* Adds Infinispan metadata to the specified DynamoDB item.
*
* Each metadata timestamp, if set (greater than -1) is encoded as
* long integer representing the number of milliseconds since the Unix
* spec:
*
*
* - created - "iat"
*
- lifespan - "max"
*
- max idle - "idl"
*
- last used - "lat"
*
*
* @param item The DynamoDB item.
* @param metadata The Infinispan metadata, {@code null} if not
* specified.
*
* @return The DynamoDB item.
*/
public static Item addMetadata(final Item item, final InternalMetadata metadata) {
return addMetadata(item, metadata, false);
}
/**
* Adds Infinispan metadata to the specified DynamoDB item.
*
* Each metadata timestamp, if set (greater than -1) is encoded as
* long integer representing the number of milliseconds since the Unix
* spec:
*
*
* - created - "iat"
*
- lifespan - "max"
*
- max idle - "idl"
*
- last used - "lat"
*
- automatic DynamoDB item expiration - "ttl"
*
*
* @param item The DynamoDB item.
* @param metadata The Infinispan metadata, {@code null} if not
* specified.
* @param withTTL {@code true} to include a "ttl" attribute as Unix
* Unix timestamp, with second precision, for automatic
* DynamoDB item expiration.
*
* @return The DynamoDB item.
*/
public static Item addMetadata(final Item item, final InternalMetadata metadata, final boolean withTTL) {
if (metadata == null) {
return item;
}
if (metadata.created() > -1) {
item.withLong("iat", metadata.created());
}
if (metadata.lifespan() > -1) {
item.withLong("max", metadata.lifespan());
}
if (metadata.maxIdle() > -1) {
item.withLong("idl", metadata.maxIdle());
}
if (metadata.lastUsed() > -1) {
item.withLong("lat", metadata.lastUsed());
}
if (withTTL && metadata.created() > -1 && metadata.lifespan() > -1) {
item.withLong("ttl", (metadata.created() + metadata.lifespan()) / 1000);
}
return item;
}
/**
* Parses Infinispan metadata encoded with {@link #addMetadata} from
* the specified DynamoDB item.
*
* @param item The DynamoDB item.
*
* @return The Infinispan metadata.
*/
public static InternalMetadata parseMetadata(final Item item) {
InternalMetadataBuilder b = new InternalMetadataBuilder();
if (item.hasAttribute("iat")) {
b.created(item.getLong("iat"));
}
if (item.hasAttribute("max")) {
b.lifespan(item.getLong("max"));
}
if (item.hasAttribute("idl")) {
b.maxIdle(item.getLong("idl"));
}
if (item.hasAttribute("lat")) {
b.lastUsed(item.getLong("lat"));
}
return b.build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy