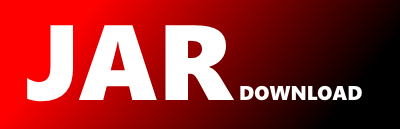
com.nimbusds.infinispan.persistence.dynamodb.config.DynamoDBStoreConfigurationChildBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-cachestore-dynamodb Show documentation
Show all versions of infinispan-cachestore-dynamodb Show documentation
Infinispan module for persisting data to an AWS DynamoDB table
The newest version!
package com.nimbusds.infinispan.persistence.dynamodb.config;
import com.amazonaws.regions.Regions;
import com.codahale.metrics.MetricRegistry;
import org.infinispan.configuration.cache.StoreConfigurationChildBuilder;
import java.util.Set;
/**
* DynamoDB store configuration child builder.
*/
public interface DynamoDBStoreConfigurationChildBuilder extends StoreConfigurationChildBuilder {
/**
* Sets the DynamoDB endpoint.
*
* @param endpoint The endpoint, {@code null} if not specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder endpoint(final String endpoint);
/**
* Sets the DynamoDB region.
*
* @param region The region, {@code null} if not specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder region(final Regions region);
/**
* Sets the class for transforming between Infinispan entries (key /
* value pair and optional metadata) and a corresponding DynamoDB item.
*
* @param itemTransformerClass The item transformer class. Must not
* be {@code null}.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder itemTransformerClass(final Class itemTransformerClass);
/**
* Sets the optional class for executing direct queries against
* DynamoDB. If set {@link #indexAttributes} must also be specified.
*
* @param queryExecutorClass The query executor class, {@code null} if
* not required.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder queryExecutorClass(final Class queryExecutorClass);
/**
* Sets the optional DynamoDB table attributes to index. If set
* {@link #queryExecutorClass} must also be specified.
*
* @param indexAttributes The attributes to index, {@code null} if not
* required.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder indexAttributes(final Set indexAttributes);
/**
* Controls consistent reads.
*
* @param enable {@code true} for consistent reads, {@code false} for
* eventually consistent.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder consistentReads(final boolean enable);
/**
* Sets the read capacity to provision when creating a new DynamoDB
* table.
*
* @param readCapacity The read capacity. Must be equal or larger than
* one.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder readCapacity(final long readCapacity);
/**
* Sets the write capacity to provision when creating a new DynamoDB
* table.
*
* @param writeCapacity The write capacity. Must be equal or larger
* than one.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder writeCapacity(final long writeCapacity);
/**
* Sets the maximum read capacity to use when scanning a DynamoDB table
* for expired items.
*
* @param purgeReadCapacity The maximum read capacity to use when
* scanning a DynamoDB table for expired
* items.
*
* @return This builder.
*/
DynamoDBStoreConfigurationChildBuilder purgeMaxReadCapacity(final Capacity purgeReadCapacity);
/**
* Sets the limit of expired entries to purge during a run of the
* expired entry reaper task.
*
* @param purgeLimit The purge limit, -1 for no limit.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder purgeLimit(final long purgeLimit);
/**
* Enables encryption at rest when provisioning a new DynamoDB table.
*
* @param enable {@code true} to create the DynamoDB table with
* encryption at rest, {@code false} without encryption.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder encryptionAtRest(final boolean enable);
/**
* Enables a stream for a global table when provisioning a new DynamoDB
* table.
*
* @param enable {@code true} to create the DynamoDB table with an
* enabled stream for a global table, {@code false} for a
* regular table.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder stream(final boolean enable);
/**
* Sets the DynamoDB table prefix.
*
* @param tablePrefix The table prefix, {@code null} if not specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder tablePrefix(final String tablePrefix);
/**
* Sets an explicit metric registry to use (other than singleton
* {@link com.nimbusds.common.monitor.MonitorRegistries}).
*
* @param metricRegistry The metric registry to use.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder metricRegistry(final MetricRegistry metricRegistry);
/**
* Sets the name of the optional range key to apply to all DynamoDB
* operations.
*
* @param rangeKeyName The range key name, {@code null} if not
* specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder applyRangeKey(final String rangeKeyName);
/**
* Sets the value of the optional range key.
*
* @param rangeKeyValue The range key value, {@code null} if not
* specified.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder rangeKeyValue(final String rangeKeyValue);
/**
* Enables deletion protection.
*
* @param enable {@code true} to enable deletion protection,
* {@code false} to not enable.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder deletionProtection(final boolean enable);
/**
* Enables continuous backups / point in time recovery (PITR).
*
* @param enable {@code true} to enable continuous backups,
* {@code false} to not enable.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder continuousBackups(final boolean enable);
/**
* Enables DynamoDB item expiration.
*
* @param enable {@code true} to enable item expiration, {@code false}
* to not enable.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder ttl(final boolean enable);
/**
* Sets the HTTP proxy host.
*
* @param host The host, {@code null} if none.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder httpProxyHost(final String host);
/**
* Sets the HTTP proxy port.
*
* @param port The port, -1 if none.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder httpProxyPort(final int port);
/**
* Sets the HMAC SHA-256 key.
*
* @param key The HMAC SHA-256 key, {@code null} if none.
*
* @return The builder.
*/
DynamoDBStoreConfigurationChildBuilder hmacSHA256Key(final String key);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy