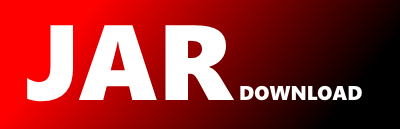
com.nimbusds.jose.jwk.JWKSelector Maven / Gradle / Ivy
package com.nimbusds.jose.jwk;
import java.util.*;
import com.nimbusds.jose.Algorithm;
/**
* Utility for selecting one or more JSON Web Keys (JWKs) from a JWK set.
*
* Supports key selection by:
*
*
* - Any, unspecified, one or more key types (typ).
*
- Any, unspecified, one or more key uses (use).
*
- Any, unspecified, one or more key operations (key_ops).
*
- Any, unspecified, one or more key algorithms (alg).
*
- Any, unspecified, one or more key identifiers (kid).
*
- Private only key.
*
- Public only key.
*
*
* Selection by X.509 certificate URL, thumbprint and chain is not
* supported.
*
* @author Vladimir Dzhuvinov
* @version $version$ (2014-04-03)
*/
public class JWKSelector {
/**
* The selected key types.
*/
private Set types;
/**
* The selected public key uses.
*/
private Set uses;
/**
* The selected key operations.
*/
private Set ops;
/**
* The selected algorithms.
*/
private Set algs;
/**
* The selected key IDs.
*/
private Set ids;
/**
* If {@code true} only private keys are matched.
*/
private boolean privateOnly = false;
/**
* If {@code true} only public keys are matched.
*/
private boolean publicOnly = false;
/**
* Gets the selected key types.
*
* @return The key types, {@code null} if not specified.
*/
public Set getKeyTypes() {
return types;
}
/**
* Sets a single selected key type.
*
* @param kty The key type, {@code null} if not specified.
*/
public void setKeyType(final KeyType kty) {
if (kty == null) {
types = null;
} else {
types = new HashSet<>(Arrays.asList(kty));
}
}
/**
* Sets the selected key types.
*
* @param types The key types.
*/
public void setKeyTypes(final KeyType ... types) {
setKeyTypes(new HashSet<>(Arrays.asList(types)));
}
/**
* Sets the selected key types.
*
* @param types The key types, {@code null} if not specified.
*/
public void setKeyTypes(final Set types) {
this.types = types;
}
/**
* Gets the selected public key uses.
*
* @return The public key uses, {@code null} if not specified.
*/
public Set getKeyUses() {
return uses;
}
/**
* Sets a single selected public key use.
*
* @param use The public key use, {@code null} if not specified.
*/
public void setKeyUse(final KeyUse use) {
if (use == null) {
uses = null;
} else {
uses = new HashSet<>(Arrays.asList(use));
}
}
/**
* Sets the selected public key uses.
*
* @param uses The public key uses.
*/
public void setKeyUses(final KeyUse... uses) {
setKeyUses(new HashSet<>(Arrays.asList(uses)));
}
/**
* Sets the selected public key uses.
*
* @param uses The public key uses, {@code null} if not specified.
*/
public void setKeyUses(final Set uses) {
this.uses = uses;
}
/**
* Gets the selected key operations.
*
* @return The key operations, {@code null} if not specified.
*/
public Set getKeyOperations() {
return ops;
}
/**
* Sets a single selected key operation.
*
* @param op The key operation, {@code null} if not specified.
*/
public void setKeyOperation(final KeyOperation op) {
if (op == null) {
ops = null;
} else {
ops = new HashSet<>(Arrays.asList(op));
}
}
/**
* Sets the selected key operations.
*
* @param ops The key operations.
*/
public void setKeyOperations(final KeyOperation... ops) {
setKeyOperations(new HashSet<>(Arrays.asList(ops)));
}
/**
* Sets the selected key operations.
*
* @param ops The key operations, {@code null} if not specified.
*/
public void setKeyOperations(final Set ops) {
this.ops = ops;
}
/**
* Gets the selected JOSE algorithms.
*
* @return The JOSE algorithms, {@code null} if not specified.
*/
public Set getAlgorithms() {
return algs;
}
/**
* Sets a singled selected JOSE algorithm.
*
* @param alg The JOSE algorithm, {@code null} if not specified.
*/
public void setAlgorithm(final Algorithm alg) {
if (alg == null) {
algs = null;
} else {
algs = new HashSet<>(Arrays.asList(alg));
}
}
/**
* Sets the selected JOSE algorithms.
*
* @param algs The JOSE algorithms.
*/
public void setAlgorithms(final Algorithm ... algs) {
setAlgorithms(new HashSet<>(Arrays.asList(algs)));
}
/**
* Sets the selected JOSE algorithms.
*
* @param algs The JOSE algorithms, {@code null} if not specified.
*/
public void setAlgorithms(final Set algs) {
this.algs = algs;
}
/**
* Gets the selected key IDs.
*
* @return The key IDs, {@code null} if not specified.
*/
public Set getKeyIDs() {
return ids;
}
/**
* Sets the selected key IDs.
*
* @param ids The key IDs.
*/
public void setKeyIDs(final String ... ids) {
setKeyIDs(new HashSet<>(Arrays.asList(ids)));
}
/**
* Sets the selected key IDs.
*
* @param ids The key IDs, {@code null} if not specified.
*/
public void setKeyIDs(final Set ids) {
this.ids = ids;
}
/**
* Sets a single selected key ID.
*
* @param id The key ID, {@code null} if not specified.
*/
public void setKeyID(final String id) {
if (id == null) {
ids = null;
} else {
ids = new HashSet<>(Arrays.asList(id));
}
}
/**
* Gets the selection of private keys.
*
* @return If {@code true} only private keys are selected.
*/
public boolean isPrivateOnly() {
return privateOnly;
}
/**
* Sets the selection of private keys.
*
* @param privateOnly If {@code true} only private keys are selected.
*/
public void setPrivateOnly(final boolean privateOnly) {
this.privateOnly = privateOnly;
}
/**
* Gets the selection of public keys.
*
* @return If {@code true} only public keys are selected.
*/
public boolean isPublicOnly() {
return publicOnly;
}
/**
* Sets the selection of public keys.
*
* @param publicOnly If {@code true} only public keys are selected.
*/
public void setPublicOnly(final boolean publicOnly) {
this.publicOnly = publicOnly;
}
/**
* Selects the keys from the specified JWK set that match the
* configured criteria.
*
* @param jwkSet The JWK set. May be {@code null}.
*
* @return The selected keys, ordered by their position in the JWK set,
* empty list if none were matched or the JWK is {@code null}.
*
*/
public List select(final JWKSet jwkSet) {
List matches = new LinkedList<>();
if (jwkSet == null)
return matches;
for (JWK key: jwkSet.getKeys()) {
if (privateOnly && ! key.isPrivate())
continue;
if (publicOnly && key.isPrivate())
continue;
if (types != null && ! types.contains(key.getKeyType()))
continue;
if (uses != null && ! uses.contains(key.getKeyUse()))
continue;
if (ops != null) {
if (ops.contains(null) && key.getKeyOperations() == null) {
// pass
} else if (key.getKeyOperations() != null && ops.containsAll(key.getKeyOperations())) {
// pass
} else {
continue;
}
}
if (algs != null && ! algs.contains(key.getAlgorithm()))
continue;
if (ids != null && ! ids.contains(key.getKeyID()))
continue;
matches.add(key);
}
return matches;
}
}