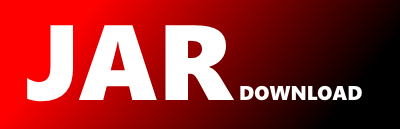
com.nimbusds.jose.crypto.AESCryptoProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nimbus-jose-jwt Show documentation
Show all versions of nimbus-jose-jwt Show documentation
Java library for Javascript Object Signing and Encryption (JOSE) and
JSON Web Tokens (JWT)
package com.nimbusds.jose.crypto;
import java.util.*;
import com.nimbusds.jose.EncryptionMethod;
import com.nimbusds.jose.JWEAlgorithm;
/**
* The base abstract class for AES encrypters and decrypters of {@link
* com.nimbusds.jose.JWEObject JWE objects}.
*
* Supports the following JSON Web Algorithms (JWAs):
*
*
* - {@link com.nimbusds.jose.JWEAlgorithm#A128KW}
*
- {@link com.nimbusds.jose.JWEAlgorithm#A192KW}
*
- {@link com.nimbusds.jose.JWEAlgorithm#A256KW}
*
- {@link com.nimbusds.jose.JWEAlgorithm#A128GCMKW}
*
- {@link com.nimbusds.jose.JWEAlgorithm#A192GCMKW}
*
- {@link com.nimbusds.jose.JWEAlgorithm#A256GCMKW}
*
*
* Supports the following encryption methods:
*
*
* - {@link com.nimbusds.jose.EncryptionMethod#A128CBC_HS256}
*
- {@link com.nimbusds.jose.EncryptionMethod#A192CBC_HS384}
*
- {@link com.nimbusds.jose.EncryptionMethod#A256CBC_HS512}
*
- {@link com.nimbusds.jose.EncryptionMethod#A128GCM}
*
- {@link com.nimbusds.jose.EncryptionMethod#A192GCM}
*
- {@link com.nimbusds.jose.EncryptionMethod#A256GCM}
*
- {@link com.nimbusds.jose.EncryptionMethod#A128CBC_HS256_DEPRECATED}
*
- {@link com.nimbusds.jose.EncryptionMethod#A256CBC_HS512_DEPRECATED}
*
*
* @author Melisa Halsband
* @version $version$ (2014-06-18)
*/
abstract class AESCryptoProvider extends BaseJWEProvider {
/**
* The supported JWE algorithms.
*/
public static final Set SUPPORTED_ALGORITHMS;
/**
* The supported encryption methods.
*/
public static final Set SUPPORTED_ENCRYPTION_METHODS;
/**
* The JWE algorithms compatible with each key size.
*/
public static final Map> COMPATIBLE_ALGORITHMS;
/**
* Initialises the supported algorithms and encryption methods.
*/
static {
Set algs = new HashSet<>();
algs.add(JWEAlgorithm.A128KW);
algs.add(JWEAlgorithm.A192KW);
algs.add(JWEAlgorithm.A256KW);
algs.add(JWEAlgorithm.A128GCMKW);
algs.add(JWEAlgorithm.A192GCMKW);
algs.add(JWEAlgorithm.A256GCMKW);
SUPPORTED_ALGORITHMS = Collections.unmodifiableSet(algs);
Set methods = new HashSet<>();
methods.add(EncryptionMethod.A128CBC_HS256);
methods.add(EncryptionMethod.A192CBC_HS384);
methods.add(EncryptionMethod.A256CBC_HS512);
methods.add(EncryptionMethod.A128GCM);
methods.add(EncryptionMethod.A192GCM);
methods.add(EncryptionMethod.A256GCM);
methods.add(EncryptionMethod.A128CBC_HS256_DEPRECATED);
methods.add(EncryptionMethod.A256CBC_HS512_DEPRECATED);
SUPPORTED_ENCRYPTION_METHODS = Collections.unmodifiableSet(methods);
Map> algsMap = new HashMap<>();
Set bit16Algs = new HashSet<>();
Set bit24Algs = new HashSet<>();
Set bit32Algs = new HashSet<>();
bit16Algs.add(JWEAlgorithm.A128GCMKW);
bit16Algs.add(JWEAlgorithm.A128KW);
bit24Algs.add(JWEAlgorithm.A192GCMKW);
bit24Algs.add(JWEAlgorithm.A192KW);
bit32Algs.add(JWEAlgorithm.A256GCMKW);
bit32Algs.add(JWEAlgorithm.A256KW);
algsMap.put(16,Collections.unmodifiableSet(bit16Algs));
algsMap.put(24,Collections.unmodifiableSet(bit24Algs));
algsMap.put(32,Collections.unmodifiableSet(bit32Algs));
COMPATIBLE_ALGORITHMS = Collections.unmodifiableMap(algsMap);
}
/**
* Creates a new AES encryption / decryption provider.
*/
protected AESCryptoProvider() {
super(SUPPORTED_ALGORITHMS, SUPPORTED_ENCRYPTION_METHODS);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy