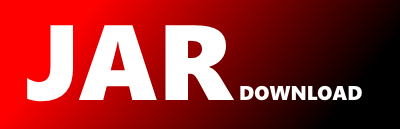
com.nimbusds.jose.jwk.JWK Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nimbus-jose-jwt Show documentation
Show all versions of nimbus-jose-jwt Show documentation
Java library for Javascript Object Signing and Encryption (JOSE) and
JSON Web Tokens (JWT)
/*
* nimbus-jose-jwt
*
* Copyright 2012-2016, Connect2id Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of the
* License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed
* under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.nimbusds.jose.jwk;
import com.nimbusds.jose.Algorithm;
import com.nimbusds.jose.JOSEException;
import com.nimbusds.jose.util.Base64;
import com.nimbusds.jose.util.*;
import com.nimbusds.jwt.util.DateUtils;
import java.io.Serializable;
import java.net.URI;
import java.security.*;
import java.security.cert.X509Certificate;
import java.security.interfaces.ECPrivateKey;
import java.security.interfaces.ECPublicKey;
import java.security.interfaces.RSAPrivateKey;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.ECParameterSpec;
import java.text.ParseException;
import java.util.*;
/**
* The base abstract class for JSON Web Keys (JWKs). It serialises to a JSON
* object.
*
* The following JSON object members are common to all JWK types:
*
*
* - {@link #getKeyType kty} (required)
*
- {@link #getKeyUse use} (optional)
*
- {@link #getKeyOperations key_ops} (optional)
*
- {@link #getKeyID kid} (optional)
*
- {@link #getX509CertURL() x5u} (optional)
*
- {@link #getX509CertThumbprint() x5t} (optional)
*
- {@link #getX509CertSHA256Thumbprint() x5t#S256} (optional)
*
- {@link #getX509CertChain() x5c} (optional)
*
- {@link #getExpirationTime() exp} (optional)
*
- {@link #getNotBeforeTime() nbf} (optional)
*
- {@link #getIssueTime() iat} (optional)
*
- {@link #getKeyRevocation() revoked} (optional)
*
- {@link #getKeyStore()}
*
*
* Example JWK (of the Elliptic Curve type):
*
*
* {
* "kty" : "EC",
* "crv" : "P-256",
* "x" : "MKBCTNIcKUSDii11ySs3526iDZ8AiTo7Tu6KPAqv7D4",
* "y" : "4Etl6SRW2YiLUrN5vfvVHuhp7x8PxltmWWlbbM4IFyM",
* "use" : "enc",
* "kid" : "1"
* }
*
*
* @author Vladimir Dzhuvinov
* @author Justin Richer
* @author Stefan Larsson
* @version 2024-04-27
*/
public abstract class JWK implements Serializable {
private static final long serialVersionUID = 1L;
/**
* The MIME type of JWK objects:
* {@code application/jwk+json; charset=UTF-8}
*/
public static final String MIME_TYPE = "application/jwk+json; charset=UTF-8";
/**
* The key type, required.
*/
private final KeyType kty;
/**
* The key use, optional.
*/
private final KeyUse use;
/**
* The key operations, optional.
*/
private final Set ops;
/**
* The intended JOSE algorithm for the key, optional.
*/
private final Algorithm alg;
/**
* The key ID, optional.
*/
private final String kid;
/**
* X.509 certificate URL, optional.
*/
private final URI x5u;
/**
* X.509 certificate SHA-1 thumbprint, optional.
*/
@Deprecated
private final Base64URL x5t;
/**
* X.509 certificate SHA-256 thumbprint, optional.
*/
private final Base64URL x5t256;
/**
* The X.509 certificate chain, optional.
*/
private final List x5c;
/**
* The key expiration time, optional.
*/
private final Date exp;
/**
* The key not-before time, optional.
*/
private final Date nbf;
/**
* The key issued-at time, optional.
*/
private final Date iat;
/**
* The key revocation, optional.
*/
private final KeyRevocation revocation;
/**
* The parsed X.509 certificate chain, optional.
*/
private final List parsedX5c;
/**
* Reference to the underlying key store, {@code null} if none.
*/
private final KeyStore keyStore;
/**
* Creates a new JSON Web Key (JWK).
*
* @param kty The key type. Must not be {@code null}.
* @param use The key use, {@code null} if not specified or if the
* key is intended for signing as well as encryption.
* @param ops The key operations, {@code null} if not specified.
* @param alg The intended JOSE algorithm for the key, {@code null}
* if not specified.
* @param kid The key ID, {@code null} if not specified.
* @param x5u The X.509 certificate URL, {@code null} if not
* specified.
* @param x5t The X.509 certificate thumbprint, {@code null} if not
* specified.
* @param x5t256 The X.509 certificate SHA-256 thumbprint, {@code null}
* if not specified.
* @param x5c The X.509 certificate chain, {@code null} if not
* specified.
* @param ks Reference to the underlying key store, {@code null} if
* none.
*/
@Deprecated
protected JWK(final KeyType kty,
final KeyUse use,
final Set ops,
final Algorithm alg,
final String kid,
final URI x5u,
final Base64URL x5t,
final Base64URL x5t256,
final List x5c,
final KeyStore ks) {
this(kty, use, ops, alg, kid, x5u, x5t, x5t256, x5c, null, null, null, ks);
}
/**
* Creates a new JSON Web Key (JWK).
*
* @param kty The key type. Must not be {@code null}.
* @param use The key use, {@code null} if not specified or if the
* key is intended for signing as well as encryption.
* @param ops The key operations, {@code null} if not specified.
* @param alg The intended JOSE algorithm for the key, {@code null}
* if not specified.
* @param kid The key ID, {@code null} if not specified.
* @param x5u The X.509 certificate URL, {@code null} if not
* specified.
* @param x5t The X.509 certificate thumbprint, {@code null} if not
* specified.
* @param x5t256 The X.509 certificate SHA-256 thumbprint, {@code null}
* if not specified.
* @param x5c The X.509 certificate chain, {@code null} if not
* specified.
* @param exp The key expiration time, {@code null} if not
* specified.
* @param nbf The key not-before time, {@code null} if not
* specified.
* @param iat The key issued-at time, {@code null} if not specified.
* @param ks Reference to the underlying key store, {@code null} if
* none.
*/
@Deprecated
protected JWK(final KeyType kty,
final KeyUse use,
final Set ops,
final Algorithm alg,
final String kid,
final URI x5u,
final Base64URL x5t,
final Base64URL x5t256,
final List x5c,
final Date exp,
final Date nbf,
final Date iat,
final KeyStore ks) {
this(kty, use, ops, alg, kid, x5u, x5t, x5t256, x5c, exp, nbf, iat, null, ks);
}
/**
* Creates a new JSON Web Key (JWK).
*
* @param kty The key type. Must not be {@code null}.
* @param use The key use, {@code null} if not specified or if
* the key is intended for signing as well as
* encryption.
* @param ops The key operations, {@code null} if not specified.
* @param alg The intended JOSE algorithm for the key,
* {@code null} if not specified.
* @param kid The key ID, {@code null} if not specified.
* @param x5u The X.509 certificate URL, {@code null} if not
* specified.
* @param x5t The X.509 certificate thumbprint, {@code null} if
* not specified.
* @param x5t256 The X.509 certificate SHA-256 thumbprint,
* {@code null} if not specified.
* @param x5c The X.509 certificate chain, {@code null} if not
* specified.
* @param exp The key expiration time, {@code null} if not
* specified.
* @param nbf The key not-before time, {@code null} if not
* specified.
* @param iat The key issued-at time, {@code null} if not
* specified.
* @param revocation The key revocation, {@code null} if not specified.
* @param ks Reference to the underlying key store,
* {@code null} if none.
*/
protected JWK(final KeyType kty,
final KeyUse use,
final Set ops,
final Algorithm alg,
final String kid,
final URI x5u,
final Base64URL x5t,
final Base64URL x5t256,
final List x5c,
final Date exp,
final Date nbf,
final Date iat,
final KeyRevocation revocation,
final KeyStore ks) {
this.kty = Objects.requireNonNull(kty, "The key type \"" + JWKParameterNames.KEY_TYPE + "\" parameter must not be null");
if (! KeyUseAndOpsConsistency.areConsistent(use, ops)) {
throw new IllegalArgumentException("The key use \"" + JWKParameterNames.PUBLIC_KEY_USE + "\" and key options \"" + JWKParameterNames.KEY_OPS + "\" parameters are not consistent, " +
"see RFC 7517, section 4.3");
}
this.use = use;
this.ops = ops;
this.alg = alg;
this.kid = kid;
this.x5u = x5u;
this.x5t = x5t;
this.x5t256 = x5t256;
if (x5c != null && x5c.isEmpty()) {
throw new IllegalArgumentException("The X.509 certificate chain \"" + JWKParameterNames.X_509_CERT_CHAIN + "\" must not be empty");
}
this.x5c = x5c;
try {
parsedX5c = X509CertChainUtils.parse(x5c);
} catch (ParseException e) {
throw new IllegalArgumentException("Invalid X.509 certificate chain \"" + JWKParameterNames.X_509_CERT_CHAIN + "\": " + e.getMessage(), e);
}
this.exp = exp;
this.nbf = nbf;
this.iat = iat;
this.revocation = revocation;
this.keyStore = ks;
}
/**
* Returns the type ({@code kty}) of this JWK.
*
* @return The key type.
*/
public KeyType getKeyType() {
return kty;
}
/**
* Returns the use ({@code use}) of this JWK.
*
* @return The key use, {@code null} if not specified or if the key is
* intended for signing as well as encryption.
*/
public KeyUse getKeyUse() {
return use;
}
/**
* Returns the operations ({@code key_ops}) for this JWK.
*
* @return The key operations, {@code null} if not specified.
*/
public Set getKeyOperations() {
return ops;
}
/**
* Returns the intended JOSE algorithm ({@code alg}) for this JWK.
*
* @return The intended JOSE algorithm, {@code null} if not specified.
*/
public Algorithm getAlgorithm() {
return alg;
}
/**
* Returns the ID ({@code kid}) of this JWK. The key ID can be used to
* match a specific key. This can be used, for instance, to choose a
* key within a {@link JWKSet} during key rollover. The key ID may also
* correspond to a JWS/JWE {@code kid} header parameter value.
*
* @return The key ID, {@code null} if not specified.
*/
public String getKeyID() {
return kid;
}
/**
* Returns the X.509 certificate URL ({@code x5u}) of this JWK.
*
* @return The X.509 certificate URL, {@code null} if not specified.
*/
public URI getX509CertURL() {
return x5u;
}
/**
* Returns the X.509 certificate SHA-1 thumbprint ({@code x5t}) of this
* JWK.
*
* @return The X.509 certificate SHA-1 thumbprint, {@code null} if not
* specified.
*/
@Deprecated
public Base64URL getX509CertThumbprint() {
return x5t;
}
/**
* Returns the X.509 certificate SHA-256 thumbprint ({@code x5t#S256})
* of this JWK.
*
* @return The X.509 certificate SHA-256 thumbprint, {@code null} if
* not specified.
*/
public Base64URL getX509CertSHA256Thumbprint() {
return x5t256;
}
/**
* Returns the X.509 certificate chain ({@code x5c}) of this JWK.
*
* @return The X.509 certificate chain as a unmodifiable list,
* {@code null} if not specified.
*/
public List getX509CertChain() {
if (x5c == null) {
return null;
}
return Collections.unmodifiableList(x5c);
}
/**
* Returns the parsed X.509 certificate chain ({@code x5c}) of this
* JWK.
*
* @return The X.509 certificate chain as a unmodifiable list,
* {@code null} if not specified.
*/
public List getParsedX509CertChain() {
if (parsedX5c == null) {
return null;
}
return Collections.unmodifiableList(parsedX5c);
}
/**
* Returns the expiration time ({@code exp}) if this JWK.
*
* @return The expiration time, {@code null} if not specified.
*/
public Date getExpirationTime() {
return exp;
}
/**
* Returns the not-before ({@code nbf}) of this JWK.
*
* @return The not-before time, {@code null} if not specified.
*/
public Date getNotBeforeTime() {
return nbf;
}
/**
* Returns the issued-at ({@code iat}) time of this JWK.
*
* @return The issued-at time, {@code null} if not specified.
*/
public Date getIssueTime() {
return iat;
}
/**
* Returns the key revocation ({@code revoked}) of this JWK.
*
* @return The key revocation, {@code null} if not specified.
*/
public KeyRevocation getKeyRevocation() {
return revocation;
}
/**
* Returns a reference to the underlying key store.
*
* @return The underlying key store, {@code null} if none.
*/
public KeyStore getKeyStore() {
return keyStore;
}
/**
* Returns the required JWK parameters. Intended as input for JWK
* thumbprint computation. See RFC 7638 for more information.
*
* @return The required JWK parameters, sorted alphanumerically by key
* name and ready for JSON serialisation.
*/
public abstract LinkedHashMap getRequiredParams();
/**
* Computes the SHA-256 thumbprint of this JWK. See RFC 7638 for more
* information.
*
* @return The SHA-256 thumbprint.
*
* @throws JOSEException If the SHA-256 hash algorithm is not
* supported.
*/
public Base64URL computeThumbprint()
throws JOSEException {
return computeThumbprint("SHA-256");
}
/**
* Computes the thumbprint of this JWK using the specified hash
* algorithm. See RFC 7638 for more information.
*
* @param hashAlg The hash algorithm. Must not be {@code null}.
*
* @return The SHA-256 thumbprint.
*
* @throws JOSEException If the hash algorithm is not supported.
*/
public Base64URL computeThumbprint(final String hashAlg)
throws JOSEException {
return ThumbprintUtils.compute(hashAlg, this);
}
/**
* Computes the SHA-256 thumbprint URI of this JWK. See RFC 7638 and
* draft-ietf-oauth-jwk-thumbprint-uri for more information.
*
* @return The SHA-256 thumbprint URI.
*
* @throws JOSEException If the SHA-256 hash algorithm is not
* supported.
*/
public ThumbprintURI computeThumbprintURI()
throws JOSEException {
return new ThumbprintURI("sha-256", computeThumbprint("SHA-256"));
}
/**
* Returns {@code true} if this JWK contains private or sensitive
* (non-public) parameters.
*
* @return {@code true} if this JWK contains private parameters, else
* {@code false}.
*/
public abstract boolean isPrivate();
/**
* Creates a copy of this JWK with all private or sensitive parameters
* removed.
*
* @return The newly created public JWK, or {@code null} if none can be
* created.
*/
public abstract JWK toPublicJWK();
/**
* Returns the size of this JWK.
*
* @return The JWK size, in bits.
*/
public abstract int size();
/**
* Casts this JWK to an RSA JWK.
*
* @return The RSA JWK.
*/
public RSAKey toRSAKey() {
return (RSAKey)this;
}
/**
* Casts this JWK to an EC JWK.
*
* @return The EC JWK.
*/
public ECKey toECKey() {
return (ECKey)this;
}
/**
* Casts this JWK to an octet sequence JWK.
*
* @return The octet sequence JWK.
*/
public OctetSequenceKey toOctetSequenceKey() {
return (OctetSequenceKey)this;
}
/**
* Casts this JWK to an octet key pair JWK.
*
* @return The octet key pair JWK.
*/
public OctetKeyPair toOctetKeyPair() {
return (OctetKeyPair)this;
}
/**
* Returns a JSON object representation of this JWK. This method is
* intended to be called from extending classes.
*
* Example:
*
*
* {
* "kty" : "RSA",
* "use" : "sig",
* "kid" : "fd28e025-8d24-48bc-a51a-e2ffc8bc274b"
* }
*
*
* @return The JSON object representation.
*/
public Map toJSONObject() {
Map o = JSONObjectUtils.newJSONObject();
o.put(JWKParameterNames.KEY_TYPE, kty.getValue());
if (use != null) {
o.put(JWKParameterNames.PUBLIC_KEY_USE, use.identifier());
}
if (ops != null) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy