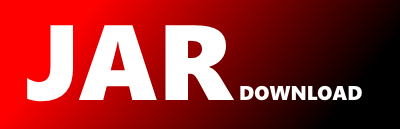
com.nimbusds.jose.jwk.JWKParameterNames Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nimbus-jose-jwt Show documentation
Show all versions of nimbus-jose-jwt Show documentation
Java library for Javascript Object Signing and Encryption (JOSE) and
JSON Web Tokens (JWT)
package com.nimbusds.jose.jwk;
import com.nimbusds.jose.HeaderParameterNames;
import com.nimbusds.jwt.JWTClaimNames;
/**
* JSON Web Key (JWK) parameter names. The JWK parameter names defined in
* RFC 7517 (JWK),
* RFC 7518 (JWA)
* and other JOSE related standards are tracked in a
* JWK
* parameters registry administered by IANA.
*
* @author Nathaniel Hart
* @version 2024-04-27
*/
public final class JWKParameterNames {
////////////////////////////////////////////////////////////////////////////////
// Generic Key Parameters
////////////////////////////////////////////////////////////////////////////////
/**
* @see RFC 7517 "kty" (Key Type) Parameter
*/
public static final String KEY_TYPE = "kty";
/**
* @see RFC 7517 "use" (Public Key Use) Parameter
*/
public static final String PUBLIC_KEY_USE = "use";
/**
* @see RFC 7517 "key_ops" (Key Operations) Parameter
*/
public static final String KEY_OPS = "key_ops";
/**
* @see RFC 7517 "alg" (Algorithm) Parameter
*/
public static final String ALGORITHM = HeaderParameterNames.ALGORITHM;
/**
* @see RFC 7517 "kid" (Key ID) Parameter
*/
public static final String KEY_ID = HeaderParameterNames.KEY_ID;
/**
* @see RFC 7517 "x5u" (X.509 Certificate URL) Parameter
*/
public static final String X_509_CERT_URL = HeaderParameterNames.X_509_CERT_URL;
/**
* @see RFC 7517 "x5c" (X.509 Certificate Chain) Parameter
*/
public static final String X_509_CERT_CHAIN = HeaderParameterNames.X_509_CERT_CHAIN;
/**
* @see RFC 7517 "x5t" (X.509 Certificate SHA-1 Thumbprint) Parameter
*/
public static final String X_509_CERT_SHA_1_THUMBPRINT = HeaderParameterNames.X_509_CERT_SHA_1_THUMBPRINT;
/**
* @see RFC 7517 "x5t#S256" (X.509 Certificate SHA-256 Thumbprint) Header
* Parameter
*/
public static final String X_509_CERT_SHA_256_THUMBPRINT = HeaderParameterNames.X_509_CERT_SHA_256_THUMBPRINT;
/**
* @see OpenID Federation 1.0
*/
public static final String EXPIRATION_TIME = JWTClaimNames.EXPIRATION_TIME;
/**
* @see OpenID Federation 1.0
*/
public static final String NOT_BEFORE = JWTClaimNames.NOT_BEFORE;
/**
* @see OpenID Federation 1.0
*/
public static final String ISSUED_AT = JWTClaimNames.ISSUED_AT;
/**
* @see OpenID Federation 1.0
*/
public static final String REVOKED = "revoked";
////////////////////////////////////////////////////////////////////////////////
// Algorithm-Specific Key Parameters
////////////////////////////////////////////////////////////////////////////////
// EC
/**
* Used with {@link KeyType#EC}.
*
* @see RFC 7518 "crv" (EC Curve) Parameter
*/
public static final String ELLIPTIC_CURVE = "crv";
/**
* Used with {@link KeyType#EC}.
*
* @see RFC 7518 "x" (EC X Coordinate) Parameter
*/
public static final String ELLIPTIC_CURVE_X_COORDINATE = "x";
/**
* Used with {@link KeyType#EC}.
*
* @see RFC 7518 "y" (EC Y Coordinate) Parameter
*/
public static final String ELLIPTIC_CURVE_Y_COORDINATE = "y";
/**
* Used with {@link KeyType#EC}.
*
* @see RFC 7518 "d" (EC Private Key) Parameter
*/
public static final String ELLIPTIC_CURVE_PRIVATE_KEY = "d";
// RSA
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "n" (RSA Modulus) Parameter
*/
public static final String RSA_MODULUS = "n";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "e" (RSA Exponent) Parameter
*/
public static final String RSA_EXPONENT = "e";
/**
* Used with {@link KeyType#OKP}.
*
* @see RFC 7518 "d" (RSA Private Exponent) Parameter
*/
public static final String RSA_PRIVATE_EXPONENT = ELLIPTIC_CURVE_PRIVATE_KEY;
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "p" (RSA First Prime Factor) Parameter
*/
public static final String RSA_FIRST_PRIME_FACTOR = "p";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "q" (RSA Second Prime Factor) Parameter
*/
public static final String RSA_SECOND_PRIME_FACTOR = "q";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "dp" (RSA First Factor CRT Exponent) Parameter
*/
public static final String RSA_FIRST_FACTOR_CRT_EXPONENT = "dp";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "dq" (RSA Second Factor CRT Exponent) Parameter
*/
public static final String RSA_SECOND_FACTOR_CRT_EXPONENT = "dq";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "qi" (RSA First CRT Coefficient) Parameter
*/
public static final String RSA_FIRST_CRT_COEFFICIENT = "qi";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "oth" (RSA Other Primes Info) Parameter
*/
public static final String RSA_OTHER_PRIMES = "oth";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "r" (RSA Other Primes Info - Prime Factor)
*/
public static final String RSA_OTHER_PRIMES__PRIME_FACTOR = "r";
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "d" (RSA Other Primes Info - Factor CRT Exponent)
*/
public static final String RSA_OTHER_PRIMES__FACTOR_CRT_EXPONENT = ELLIPTIC_CURVE_PRIVATE_KEY;
/**
* Used with {@link KeyType#RSA}.
*
* @see RFC 7518 "t" (RSA Other Primes Info - Factor CRT Coefficient)
*/
public static final String RSA_OTHER_PRIMES__FACTOR_CRT_COEFFICIENT = "t";
// OCT
/**
* Used with {@link KeyType#OCT}
*
* @see RFC 7518 "k" (OCT Key Value) Parameter
*/
public static final String OCT_KEY_VALUE = "k";
// OKP
/**
* Used with {@link KeyType#OKP}.
*
* @see RFC 8037 "crv" (OKP Key Subtype) Parameter
*/
public static final String OKP_SUBTYPE = ELLIPTIC_CURVE;
/**
* Used with {@link KeyType#OKP}.
*
* @see RFC 8037 "x" (OKP Public Key) Parameter
*/
public static final String OKP_PUBLIC_KEY = ELLIPTIC_CURVE_X_COORDINATE;
/**
* Used with {@link KeyType#OKP}.
*
* @see RFC 8037 "d" (OKP Private Key) Parameter
*/
public static final String OKP_PRIVATE_KEY = ELLIPTIC_CURVE_PRIVATE_KEY;
private JWKParameterNames() {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy