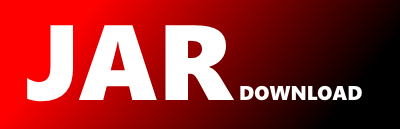
com.nimbusds.jose.jwk.loader.JWKMetaLogger Maven / Gradle / Ivy
package com.nimbusds.jose.jwk.loader;
import java.security.cert.X509Certificate;
import java.time.format.DateTimeFormatter;
import java.util.Arrays;
import java.util.Date;
import com.nimbusds.jose.jwk.JWK;
import com.nimbusds.jose.jwk.JWKSet;
import com.nimbusds.jose.jwk.KeyType;
import com.nimbusds.jose.util.X509CertUtils;
/**
* JSON Web Key (JWK) meta parameter logger.
*/
class JWKMetaLogger {
/**
* Logs the meta parameters of the keys in the JWK set at INFO level.
*
* @param jwkSet The JWK set. Must not be {@code null}.
*/
static void log(final JWKSet jwkSet) {
Loggers.MAIN_LOG.info("[SE1008] JWK set:");
for (int i = 0; i < jwkSet.getKeys().size(); i++) {
JWKMetaLogger.log(i + 1, jwkSet.getKeys().get(i));
}
}
/**
* Logs the JWK meta parameters at INFO level.
*
* @param pos The JWK position (index).
* @param jwk The JWK, {@code null} if not specified.
*/
static void log(final int pos, final JWK jwk) {
if (jwk == null) {
return;
}
String isPrivate = "";
if (Arrays.asList(KeyType.RSA, KeyType.EC).contains(jwk.getKeyType())) {
isPrivate = jwk.isPrivate() ? " private=true" : " private=false";
}
String validityTimeWindow = "";
final boolean x509CertPresent =
jwk.getX509CertChain() != null
&& ! jwk.getX509CertChain().isEmpty()
&& jwk.getX509CertChain().get(0) != null;
if (x509CertPresent) {
byte[] derCert = jwk.getX509CertChain().get(0).decode();
X509Certificate cert = X509CertUtils.parse(derCert);
if (cert != null) {
Date nbf = cert.getNotBefore();
if (nbf != null) {
validityTimeWindow = "nbf=" +
DateTimeFormatter.ISO_INSTANT.format(nbf.toInstant());
}
Date exp = cert.getNotAfter();
if (exp != null) {
validityTimeWindow += " exp=" +
DateTimeFormatter.ISO_INSTANT.format(exp.toInstant());
}
}
}
Loggers.MAIN_LOG.info("[SE3000] [{}] JWK type={} id={}{} use={} size={} x5c={} pkcs#11={} {}",
pos,
jwk.getKeyType(),
jwk.getKeyID(),
isPrivate,
jwk.getKeyUse(),
jwk.size(),
x509CertPresent,
PKCS11Utils.isPKCS11Key(jwk),
validityTimeWindow
);
}
/**
* Prevents public instantiation.
*/
private JWKMetaLogger() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy